Fast Output in Java
Last Updated :
04 Jul, 2022
While doing problems in various coding platforms in some questions we end up with (TLE). At that point of time even if we use fast input then also, sometimes (TLE) remains in Java. At that time if we use fast output with fast input it can reduce the time taken. The fast output is generally used when we have to print numerous items which is shown later and discussed below as method 2
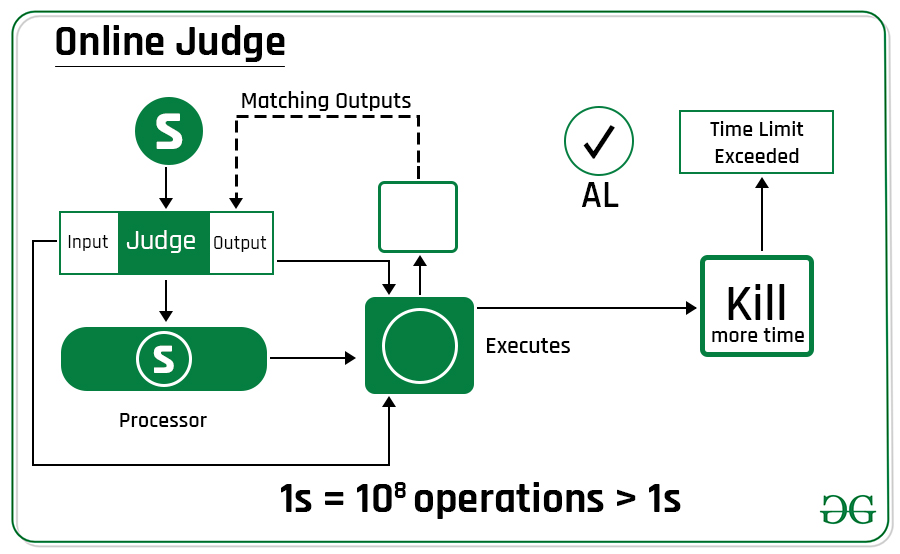
Methods:
- Without using fast output(Naive)
- Using fast output (Optimal)
Method 1: Without using fast output (Standard Method)
System.out.println() is used to print an argument that is passed to it. It can be broken into three parts
- System: It is a final class defined in java.lang package.
- out: It is a static member field of System class and is of type PrintStream
- println(): It is a method of PrintStream class. println prints the argument passed to the standard console and a newline.
Implementation: Program in which we are printing the value from 0 to 10^5
Example
Java
/**
* @author Akhil
*/
import java.io.*;
class GFG {
public static void main(String[] args)
{
for ( int i = 0 ; i < 100000 ; i++)
System.out.println(i);
}
}
|
Output: 10000 numbers from 0 till 9999 are printed and this program takes 0.88 seconds to execute
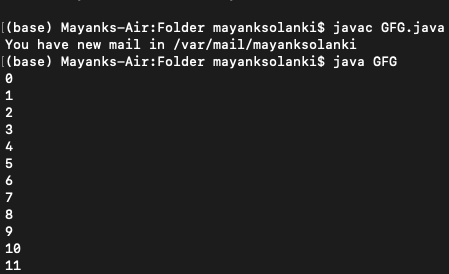
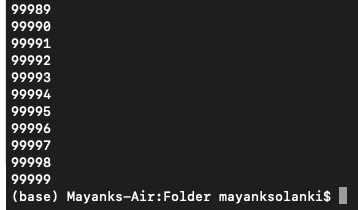
Method 2: Using fast output (Optimal)
PrintWriter class is the implementation of Writer class. By using PrintWriter than using System.out.println is preferred when we have to print a lot of items as PrintWriter is faster than the other to print data to the console.
The System.out variable is referencing an object of type PrintStream which wraps a BufferedOutputStream. When you call one of the write() methods on PrintStream, it internally flushes the buffer of the underlying BufferedOutputStream. It doesn’t happen with PrintWriter. We have to do it ourselves.
Implementation:
Example
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
PrintWriter out= new PrintWriter(System.out);
for ( int i = 0 ; i < 100000 ; i++)
out.print(i + "\n" );
out.flush();
}
}
|
Output:
Same output is generated that is 10000 numbers from 0 till 9999 are printed and this program takes 0.14 seconds to execute which is 84% faster output is generated in respect to time comparing the above two examples as illustrated.
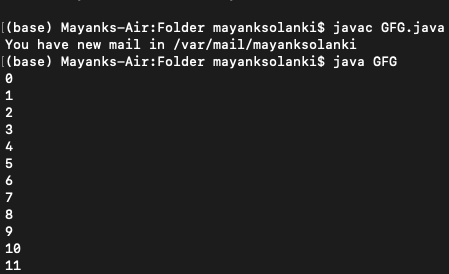
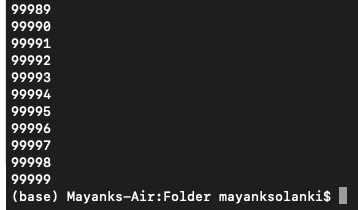
Share your thoughts in the comments
Please Login to comment...