Express JS HTTP Methods
Last Updated :
21 Nov, 2023
In this article, we are going to learn about the different HTTP methods of Express JS. HTTP methods are hypertext transfer protocol methods to exchange data between two or more layers of an application using a request and response cycle.
In web development, we use HTTP methods to connect client-side code to server-side code and databases. The database for a particular website is too large, so it is not possible to store the database on the client side. That’s why we implement a server and setup different HTTP routes through which we can exchange data from the client side to the server side.
GET Method
The get method is a kind of HTTP request that a client makes to request some data from the server. Suppose we are creating a weather application. In order to get data about the weather, the client will make a get request from the server to get data.
The get method comes with two parameters: the first is the URL, which means the URL for which the method is listening, and the second is the callback function, where that particular callback function takes two default arguments: one is a request made by the client, and the other is a response that will be sent to the client.
Syntax:
app.get("URL",(req,res)=>{})
POST Method
The post method is used to send data from the client side to the server side that will be further stored in the database. Suppose in an application, the user registers with their credentials and details to receive those details in the backend through the request body, and storing that data in the database will be achieved under the post method.
Similarly, the post method contains two parameters:the first is the URL, which means the URL for which the method is listening, and the second is the callback function, where that particular callback function takes two default arguments: one is a request made by the client, and the other is a response that will be sent to the client.
The post-request body contains the data sent by the client, and this data can only be accessed after parsing it into JSON.
Syntax:
app.post("URL",(req,res)=>{})
PUT Method
The put method is used to update the data that is already present in the database. Suppose in an application you are updating your name, so to update that name, you need to call a PUT method request from the client side to the server side, and that data will again be sent in the body of the request, and once the data is received on the server, the data will be updated in the database.
Similarly, the put method contains two parameters:the first is the URL, which means the URL for which the method is listening, and the second is the callback function, where that particular callback function takes two default arguments: one is a request made by the client, and the other is a response that will be sent to the client.
Again the data wants to client update will comes under body section of request.
Syntax:
app.put("URL",(req,res)=>{})
DELETE Method
Delete is one of the most commonly used HTTP methods; it is used to delete particular data from a database. Suppose you are using an application consisting of notes. When you click on delete any note, it will make an HTTP delete request to the server and pass the ID of that particular note in the body of the request. Once that ID is received by the server, it will delete that particular note from Database.
Similarly, the delete method contains two parameters:the first is the URL, which means the URL for which the method is listening, and the second is the callback function, where that particular callback function takes two default arguments: one is a request made by the client, and the other is a response that will be sent to the client.
Again the ID will be comes under body section of request.
Syntax:
app.delete("URL",(req,res)=>{})
Example: Implementation of above HTTP methods.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use(express.json());
app.get( "/" , (req, res) => {
console.log( "GET Request Successfull!" );
res.send( "Get Req Successfully initiated" );
})
app.put( "/put" , (req, res) => {
console.log( "PUT REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data Update Request Recieved`);
})
app.post( "/post" , (req, res) => {
console.log( "POST REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data POSt Request Recieved`);
})
app. delete ( "/delete" , (req, res) => {
console.log( "DELETE REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data DELETE Request Recieved`);
})
app.listen(PORT, () => {
console.log(`Server established at ${PORT}`);
})
|
Output:
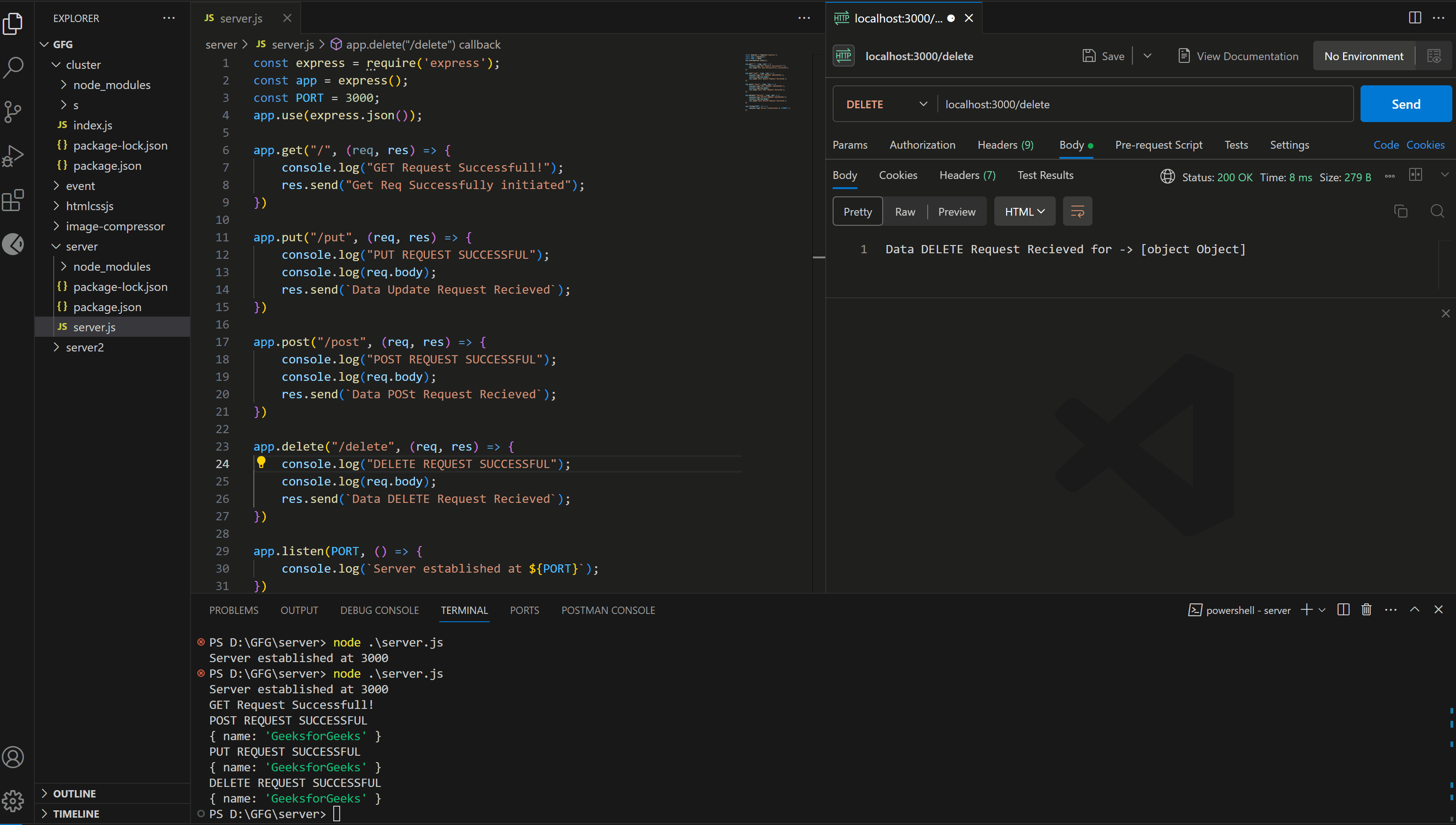
Share your thoughts in the comments
Please Login to comment...