Explain the concept of Redux in React.
Last Updated :
30 Jan, 2024
Redux is a state management library commonly used with React, although it can also be used with other JavaScript frameworks. It helps manage the state of your application. It was inspired by Flux, another state management architecture developed by Facebook for building client-side web applications. In this tutorial, we’ll learn the concept of Redux in React.
Prerequisites:
How does redux work?
- Redux operates by maintaining a centralized state tree, offering a method to manage the application state and address state changes.
- Actions are dispatched to the store, initiating reducers to define how the state should change.
- Reducers, being pure functions, take the previous state and an action as input and produce the new state as output.
- Components can subscribe to the store to access and update the state, ensuring a predictable uni-directional data flow throughout the application.
Steps to Create React Application And Installing Redux:
Step 1: Create a React Vite application and Navigate to the project directory the following command:
npm init vite@latest my-react-redux-project --template react
cd my-react-redux-project
Step 2: Install Redux and React-Redux packages
npm install redux react-redux
Project Structure:
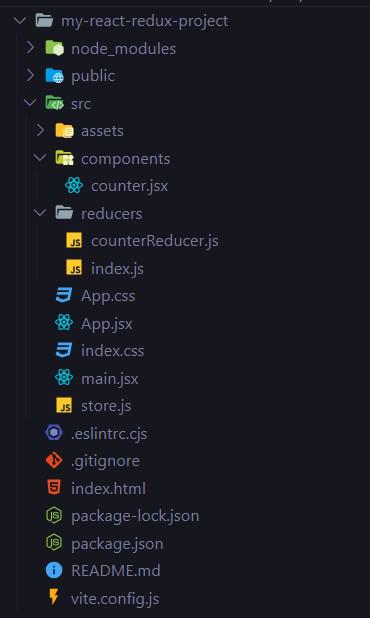
Folder Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"redux": "^5.0.1"
}
Step 3: Create a new file named store.js in the src directory of your project.
Step 4: Create one or more reducer files inside the src Folder.
Step 5: Create a file named index.js inside the src/reducers directory to Combine reducers.
Step 6: Connect Redux to React.
- Open src/main.js (or src/main.jsx) file.
- Import ‘<Provider>’ from react-redux and your Redux store.
- Wrap your root component with ‘<Provider>’ and pass the Redux store as a prop.
Step 7: Now, Create your React components as usual and use the connect function from react-redux to connect your components to the Redux store.
Javascript
import { createStore } from 'redux' ;
import rootReducer from './reducers' ;
const store = createStore(rootReducer);
export default store;
|
Javascript
const initialState = {
count: 0
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT' :
return { ...state, count: state.count + 1 };
case 'DECREMENT' :
return { ...state, count: state.count - 1 };
default :
return state;
}
};
export default counterReducer;
|
Javascript
import { combineReducers } from 'redux' ;
import counterReducer from './counterReducer' ;
const rootReducer = combineReducers({
counter: counterReducer
});
export default rootReducer;
|
Javascript
import React from 'react' ;
import { connect } from 'react-redux' ;
const Counter = ({ count, increment, decrement }) => {
return (
<div>
<h1 style={{ color: 'green' }}>
GFG Counter App using Redux</h1>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => ({
count: state.counter.count
});
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
});
export default connect(mapStateToProps,
mapDispatchToProps)(Counter);
|
Start Your Application using the following command:
npm run dev
Output:
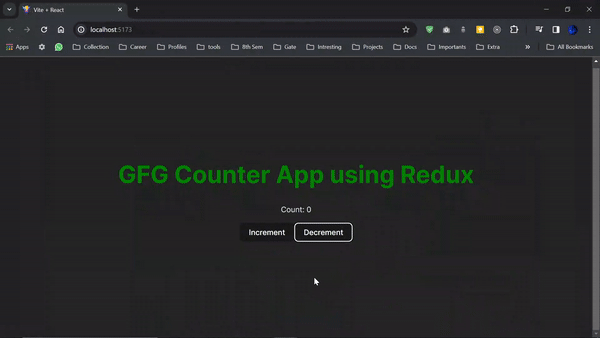
Final output
Advantages of Redux:
- Centralized State Management: Redux maintains a single, global state tree for effective management of application state.
- Predictable State Changes: Redux follows a clear and predictable pattern where actions trigger reducers to update the state, simplifying debugging and understanding of state changes.
- Unidirectional Data Flow: Redux enforces a unidirectional data flow, making it easy for components to subscribe to the state and ensuring consistency in application behavior.
- Scalability: Well-suited for larger applications, Redux provides a structured approach that scales efficiently as the complexity of the application grows.
Share your thoughts in the comments
Please Login to comment...