EventEmitter Object in Node.js
Last Updated :
29 Mar, 2023
We all know NodeJs applications are run in the single-thread event-driven model. However, NodeJs executes a threads pool in the background of the application, while applications don’t support multi-threads.
This is all possible by using the event model in NodeJs. In this article, we will see the EventEmitter object and how it creates the event and fires the event. But you must need to know what the event is.
Event handling is quite similar to the callback function, but the callback function is used when asynchronous functions return their result and event handling works on the observer pattern. In NodeJs the functions that listeners the events are called observers. When an event occurs listeners’ functions start their execution. There are multiple in-built events available in NodeJs like EventEmitter class. We will show how we can use EventEmitter class in event handling.
EventEmitter is an in-built event available in NodeJs. Much of the Node.js core API is built around an idiomatic asynchronous event-driven architecture in which certain kinds of objects (called “emitters”) emit named events that cause Function objects (“listeners”) to be called” EventEmitter help event emitters to fire events and listeners to act on these events in a simple manner.
Creating EventEmitter object: We can use EventEmitter by creating its object and then using it for fire events. We will syntax for EventEmitter object creation. Before creating the EventEmitter object you should import the event.
Step 1: Import events module.
var events = require('events');
Step 2: Creates an EventEmitter object.
var eventEmitter = new events.EventEmitter();
Step 3: Then bind the event and event handler together
eventEmitter.on('eventName', eventHandler);
Step 4: We can fire the event by using emit method
eventEmitter.emit('eventName');
Example 1: In this example, we show how to create an EventEmitter object and then fire an event by using emit() method, and bind the connection used on() of EventEmitter. When execution is stopped we fire connections by using emit().
index.js
Javascript
const events = require( 'events' );
let eventEmitter = new events.EventEmitter()
let connectHandler = function connected() {
console.log( 'connection successful.' );
eventEmitter.emit( 'data_received' );
}
eventEmitter.on( 'connection' , connectHandler);
eventEmitter.on( 'data_received' , function () {
console.log( 'data received successfully.' );
});
eventEmitter.emit( 'connection' );
console.log( "Program Ended." );
|
Step to run the application: Run the below command to execute script.js
node script.js
Output:
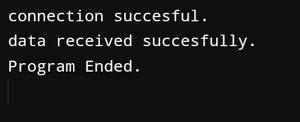
Example of EventEmitter object
Extending EventEmitter class: The EventEmitter class supports inheritance. Sometimes you need to implement a subclass of EventEmitter.
Example 2: In this example, MyEmitter inherits the EventEmitter class. MyEmitter uses the on() method to listen to events. This event is fire using emit().
index.js
Javascript
const EventEmitter = require( 'events' );
class MyEmitter extends EventEmitter {
foo() {
this .emit( 'test' );
}
}
let myEmitter = new MyEmitter();
myEmitter.on( 'test' , () => console.log( 'Yay, it works!' ));
myEmitter.foo();
|
Output:
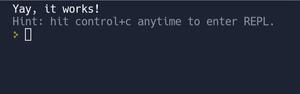
Example of Extending EventEmitter class
Features of EventEmitter class:
- This class is for handling events.
- It can inherit event functionality in other classes.
- It emits events.
- It listens to and handles events.
- It helps to run multiple threads in the background.
EventEmitter Methods:
- emitobj.on(event, listener)
- emitobj.emit(event,[arg1],[arg2],..[argN])
- emitobj.once(event, listener)
- emitobj.removeListener(event, listener)
Implementation of EventEmitter Methods:
Example: In this example, we see the practical implementation of EventEmitter Methods. We see all four methods like on(), emit(), once(), and removeListener() of EventEmitter.
Javascript
const events = require( 'events' );
const eventEmitter = new events.EventEmitter();
let listener1 = function listener1() {
console.log( 'listener1 executed.' );
}
let listener2 = function listener2() {
console.log( 'listener2 executed.' );
}
eventEmitter.addListener( 'connection' , listener1);
eventEmitter.on( 'connection' , listener2);
let eventListeners = require( 'events' ).EventEmitter.listenerCount
(eventEmitter, 'connection' );
console.log(eventListeners + " Listener(s) listening to connection event" );
eventEmitter.emit( 'connection' );
eventEmitter.removeListener( 'connection' , listener1);
console.log( "Listener1 will not listen now." );
eventEmitter.emit( 'connection' );
eventListeners = require( 'events' ).EventEmitter.listenerCount
(eventEmitter, 'connection' );
console.log(eventListeners + " Listener(s) listening to connection event" );
console.log( "Program Ended." );
|
Output:
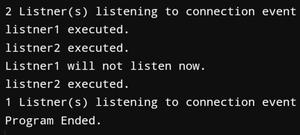
EventEmitter Methods
Share your thoughts in the comments
Please Login to comment...