Encapsulation in Golang
Last Updated :
03 Oct, 2019
Encapsulation is defined as the wrapping up of data under a single unit. It is the mechanism that binds together code and the data it manipulates. In a different way, encapsulation is a protective shield that prevents the data from being accessed by the code outside this shield.
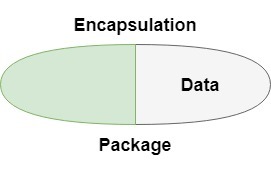
In object-oriented languages, the variables or data of a class are hidden from any other class and can be accessed only through any member function of own class in which they are declared. But Go language does not support classes and objects. So, in the Go language, encapsulation is achieved by using packages. Go provides two different types of identifiers, i.e. exported and unexported identifiers. Encapsulation is achieved by exported elements(variables, functions, methods, fields, structures) from the packages, it helps to control the visibility of the elements(variables, functions, methods, fields, structures). The elements are visible if the package in which they are defined is available in your program.
1. Exported Identifiers: Exported identifiers are those identifiers which are exported from the package in which they are defined. The first letter of these identifiers is always in capital letter. This capital letter indicates that the given identifier is exported identifier. Exported identifiers are always limited to the package in which they are defined. When you export the specified identifier from the package you simple just export the name not the implementation of that identifier. This mechanism is also applicable for function, fields, methods, and structures.
Example:
package main
import (
"fmt"
"strings"
)
func main() {
slc := []string{ "GeeksforGeeks" , "geeks" , "gfg" }
for x := 0; x < len(slc); x++ {
res := strings.ToUpper(slc[x])
fmt.Println(res)
}
}
|
Output:
GEEKSFORGEEKS
GEEKS
GFG
Explanation: In the above example, we convert the case of the elements of the slc slice to uppercase by exporting ToUpper() function from strings package.
res := strings.ToUpper(slc[x])
Here, the first letter of the ToUpper() function is in uppercase, which indicates that this function is exported function. If you try to change the case of the ToUpper() function into lowercase, then the compiler will give an error, as shown below:
res := strings.toUpper(slc[x])
Output:
./prog.go:22:9: cannot refer to unexported name strings.toUpper
./prog.go:22:9: undefined: strings.toUpper
So, this process(exporting of variables, fields, methods, functions, structures) is known as encapsulation. Due to encapsulation we only export the name of the function not the whole definition of the function in our program and the definition of the ToUpper() function is wrapped inside strings package, so for exporting ToUpper() function first you need to import strings package in your program.
2. Unexported Identifiers: Unexported identifiers are those identifiers which are not exported from any package. They are always in lowercase. As shown in the below example addition function is not related to any package, so it is an unexported function and the visibility of this method is limited to this program only.
Example:
package main
import "fmt"
func addition(val ... int ) int {
s := 0
for x := range val {
s += val[x]
}
fmt.Println( "Total Sum: " , s)
return s
}
func main() {
addition(23, 546, 65, 42, 21, 24, 67)
}
|
Output:
Total Sum: 788
Benefits of Encapsulation:
- Hiding implementation details from the user.
- Increase the reusability of the code.
- It prevents users from setting the function’s variables arbitrarily. It only sets by the function in the same package and the author of that package ensure that the function maintain their internal invariants.
Share your thoughts in the comments
Please Login to comment...