Domain-Driven Design (DDD)
Last Updated :
22 Feb, 2024
Domain-Driven Design (DDD) is an approach to software development that focuses on understanding and modeling the problem domain within which a software system operates. It emphasizes the importance of collaborating closely with domain experts to develop a deep understanding of the domain’s intricacies and complexities. DDD provides a set of principles, patterns, and practices to help developers effectively capture and express domain concepts in their software designs.
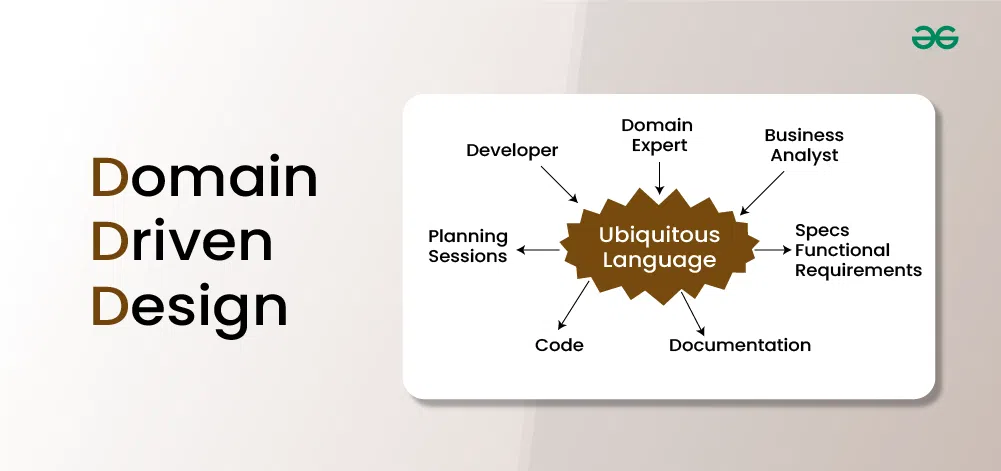
Important Topics for the Domain-Driven Design(DDD)
What is Domain-Driven Design (DDD)?
Domain
It refers to the subject area or problem space that the software system is being built to address. It encompasses the real-world concepts, rules, and processes that the software is intended to model or support. For example, in a banking application, the domain includes concepts like accounts, transactions, customers, and regulations related to banking operations.
Driven
“Driven” implies that the design of the software system is guided or influenced by the characteristics and requirements of the domain. In other words, the design decisions are based on a deep understanding of the domain, rather than being driven solely by technical considerations or implementation details.
Design
“Design” refers to the process of creating a plan or blueprint for the software system. This includes decisions about how the system will be structured, how different components will interact, and how the system will fulfill its functional and non-functional requirements. In the context of Domain-Driven Design, the focus is on designing the software in a way that accurately reflects the structure and behavior of the domain.
Domain-Driven Design is a concept introduced by a programmer Eric Evans in 2004 in his book Domain-Driven Design: Tackling Complexity in Heart of Software
Importance of Domain Knowledge
Suppose we have designed software using all the latest tech stack and infrastructure and our software design architecture is amazing, but when we release this software in the market, it is ultimately the end user who decides whether our system is great or not. Also if the system does not solve business needs, then it is of no use to anyone. No matter how pretty it looks or how well the architecture its infrastructure are.
According to Eric Evans, When we are developing software our focus should not be primarily on technology, rather it should be primarily on business. Remember,
It is not the customer’s job to know what they want” – Steve Jobs
Strategic Design in Domain-Driven Design(DDD)
Strategic Design in Domain-Driven Design (DDD) focuses on defining the overall architecture and structure of a software system in a way that aligns with the problem domain. It addresses high-level concerns such as how to organize domain concepts, how to partition the system into manageable parts, and how to establish clear boundaries between different components.
Let us see some key concepts within Strategic Design in Domain-Driven Design(DDD)
1. Bounded Contexts
- A Bounded Context represents a specific area within the overall problem domain where a particular model or language applies consistently.
- Different parts of a system may have different meanings for the same terms, and a Bounded Context defines explicit boundaries within which those terms have specific meanings.
- This allows teams to develop models that are tailored to specific contexts without introducing confusion or inconsistencies.
- Bounded Contexts help manage complexity by breaking down a large, complex domain into smaller, more manageable parts.
2. Context Mapping
- Context Mapping is the process of defining the relationships and interactions between different Bounded Contexts.
- It involves identifying areas of overlap or integration between contexts and establishing communication channels and agreements between them.
- Context Mapping helps ensure that different parts of the system can collaborate effectively while still maintaining clear boundaries between them.
- There are various patterns and techniques for Context Mapping, such as Partnership, Shared Kernel, and Customer-Supplier.
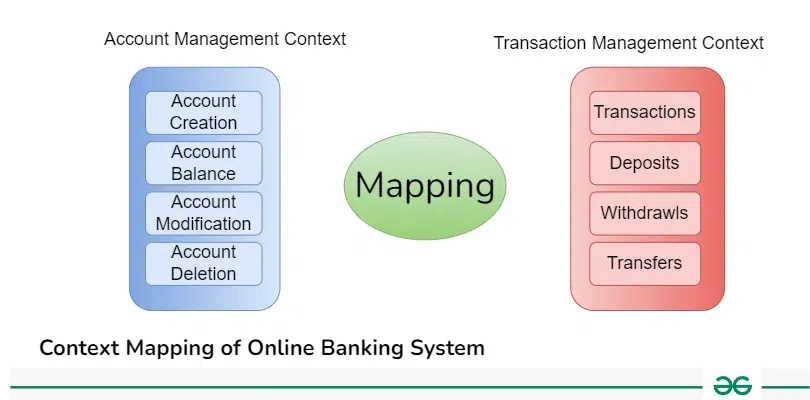
3. Strategic Patterns
- Strategic Patterns are general guidelines or principles for organizing the architecture of a software system in a way that aligns with the problem domain.
- These patterns help address common challenges in designing complex systems and provide proven approaches for structuring the system effectively.
- Examples of strategic patterns include Aggregates, Domain Events, and Anti-Corruption Layer.
- These patterns provide solutions to recurring problems in domain-driven design and help ensure that the architecture of the system reflects the underlying domain concepts accurately.
4. Shared Kernel
- Shared Kernel is a strategic pattern that involves identifying areas of commonality between different Bounded Contexts and establishing a shared subset of the domain model that is used by multiple contexts.
- This shared subset, or kernel, helps facilitate collaboration and integration between contexts while still allowing each context to maintain its own distinct model.
- Shared Kernel should be used judiciously, as it introduces dependencies between contexts and can lead to coupling if not managed carefully.
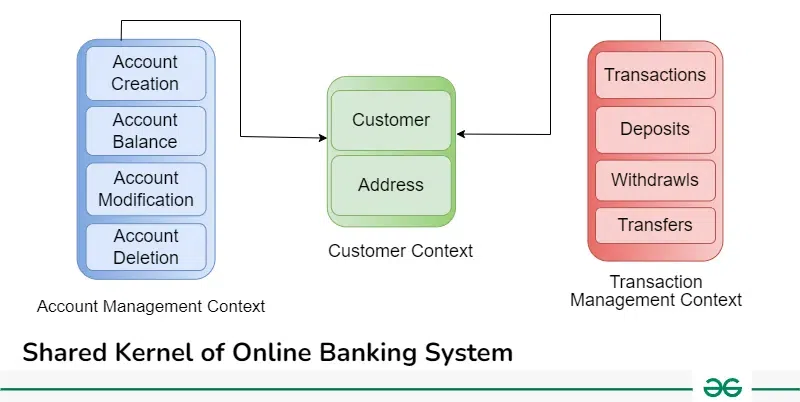
5. Anti-Corruption Layer (ACL)
- The Anti-Corruption Layer is another strategic pattern that helps protect a system from the influence of external or legacy systems that use different models or languages.
- An ACL acts as a translation layer between the external system and the core domain model, transforming data and messages as needed to ensure compatibility.
- This allows the core domain model to remain pure and focused on the problem domain, while still integrating with external systems as necessary.
6. Ubiquitous Language
Ubiquitous Language refers to a shared vocabulary or language that is used consistently and universally across all stakeholders involved in the development of a software system. This language consists of terms, phrases, and concepts that accurately represent domain knowledge and concepts.
Some of the key principles of Ubiquitous Language are:
- Shared Understanding: The primary goal of Ubiquitous Language is to establish a shared understanding of the problem domain among all members of the development team, including developers, domain experts, business analysts, and stakeholders. By using a common language, everyone involved can communicate more effectively and accurately convey domain concepts and requirements.
- Consistency and Clarity: Ubiquitous Language promotes consistency and clarity in communication by using precise and unambiguous terminology. Each term or phrase in the language should have a clear and agreed-upon meaning,
- Alignment with Business Concepts: The language used in DDD should closely align with the terminology and concepts used in the business domain. It should reflect the way domain experts think and talk about the problem domain, ensuring that the software accurately represents real-world concepts and processes.
- Evolutionary Nature: Ubiquitous Language is not static but evolves over time as the team gains a deeper understanding of the domain and as requirements change. It should adapt to reflect new insights, discoveries, or changes in business priorities, ensuring that the language remains relevant and up-to-date throughout the development process.
Tactical Design Patterns in Domain-Driven Design (DDD)
In Domain-Driven Design (DDD), tactical design patterns are specific strategies or techniques used to structure and organize the domain model within a software system. These patterns help developers effectively capture the complexity of the domain, while also promoting maintainability, flexibility, and scalability.
Let us see some of the key tactical design patterns in DDD:
1. Entity
An entity is a domain object that has a distinct identity and lifecycle. Entities are characterized by their unique identifiers and mutable state. They encapsulate behavior and data related to a specific concept within the domain.
For example, in a banking application, a BankAccount
entity might have properties like account number, balance, and owner, along with methods to deposit, withdraw, or transfer funds.
2. Value Object
A value object is a domain object that represents a conceptually immutable value. Unlike entities, value objects do not have a distinct identity and are typically used to represent attributes or properties of entities. Value objects are equality-comparable based on their properties, rather than their identity.
For example, a Money
value object might represent a specific amount of currency, encapsulating properties like currency type and amount.
3. Aggregate
- An aggregate is a cluster of domain objects that are treated as a single unit for the purpose of data consistency and transactional integrity.
- Aggregates consist of one or more entities and value objects, with one entity designated as the aggregate root.
- The aggregate root serves as the entry point for accessing and modifying the aggregate’s internal state.
- Aggregates enforce consistency boundaries within the domain model, ensuring that changes to related objects are made atomically.
For example, in an e-commerce system, an Order
aggregate might consist of entities like OrderItem
and Customer
, with the Order
entity serving as the aggregate root.
4. Repository
- A repository is a mechanism for abstracting data access and persistence logic from the domain model.
- Repositories provide a standardized interface for querying and storing domain objects, hiding the details of how data is retrieved or stored. Repositories encapsulate the logic for translating between domain objects and underlying data storage mechanisms, such as databases or external services.
- By decoupling the domain model from data access concerns, repositories enable greater flexibility and maintainability.
For example, a CustomerRepository
might provide methods for querying and storing Customer
entities.
- A factory is a creational pattern used to encapsulate the logic for creating instances of complex domain objects. Factories abstract the process of object instantiation, allowing clients to create objects without needing to know the details of their construction.
- Factories are particularly useful for creating objects that require complex initialization logic or involve multiple steps.
For example, a ProductFactory
might be responsible for creating instances of Product
entities with default configurations.
6. Service
- A service is a domain object that represents a behavior or operation that does not naturally belong to any specific entity or value object.
- Services encapsulate domain logic that operates on multiple objects or orchestrates interactions between objects.
- Services are typically stateless and focus on performing specific tasks or enforcing domain rules.
For example, an OrderService
might provide methods for processing orders, applying discounts, and calculating shipping costs.
Benefits of Domain-Driven Design(DDD)
- Shared Understanding:
- It encourages collaboration between domain experts, developers, and stakeholders.
- By encouraging a shared understanding of the problem domain through the ubiquitous language, teams can communicate more effectively and ensure that the software accurately reflects the needs and requirements of the business.
- Focus on Core Domain:
- It helps teams identify and prioritize the core domain of the application—the areas of the system that provide the most value to the business. By focusing development efforts on the core domain, teams can deliver functionality that directly addresses business objectives and differentiates the software from competitors.
- Resilience to Change:
- It emphasizes designing software systems that are resilient to change by modeling the domain in a way that reflects the inherent complexities and uncertainties of the problem domain.
- By embracing change as a natural part of software development, teams can respond more effectively to evolving business needs and market conditions.
- Clear Separation of Concerns:
- DDD encourages a clear separation of concerns between domain logic, infrastructure concerns, and user interface concerns. By isolating domain logic from technical details and infrastructure concerns, teams can maintain a clean and focused domain model that is independent of specific implementation details or technological choices.
- Improved Testability:
- It promotes the use of domain objects with well-defined boundaries and behaviors, making it easier to write better and focused tests that verify the correctness of domain logic.
- By designing software systems with testability in mind, teams can ensure that changes to the codebase are safe and predictable, reducing the risk of introducing regressions or unintended side effects.
- Support for Complex Business Rules:
- It provides patterns and techniques for modeling and implementing complex business rules and workflows within the domain model.
- By representing business rules explicitly in the domain model, teams can ensure that the software accurately reflects the intricacies of the business domain and enforces domain-specific constraints and requirements.
- Alignment with Business Goals:
- Ultimately, It aims to align software development efforts with the strategic goals and objectives of the business. By focusing on understanding and modeling the problem domain, teams can deliver software solutions that directly support business objectives, drive innovation, and create value for stakeholders and end-users.
Challenges of Domain-Driven Design (DDD)
- Complexity:
- DDD can introduce complexity, especially in large and complex domains.
- Modeling intricate business domains accurately requires a deep understanding of the domain and may involve dealing with ambiguity and uncertainty. Managing this complexity effectively requires careful planning, collaboration, and expertise.
- Ubiquitous Language Adoption:
- Establishing and maintaining a ubiquitous language—a shared vocabulary that accurately represents domain concepts—can be challenging. It requires collaboration between developers and domain experts to identify and agree upon domain terms and meanings.
- Achieving consensus on the ubiquitous language may require overcoming communication barriers and reconciling differences in terminology and perspectives.
- Bounded Context Alignment:
- In large and complex domains, different parts of the domain may have distinct models and bounded contexts. Aligning these bounded contexts and ensuring consistency between them can be challenging.
- It requires clear communication, collaboration, and coordination between teams working on different parts of the domain to avoid inconsistencies and conflicts.
- Technical Complexity:
- Implementing DDD principles and patterns effectively may require adopting new technologies, frameworks, and architectural approaches. Integrating DDD with existing systems or legacy codebases can be complex and may require refactoring or redesigning existing code to align with DDD principles.
- Technical challenges such as performance, scalability, and maintainability must be carefully addressed to ensure the success of DDD adoption.
- Resistance to Change:
- Introducing DDD may encounter resistance from team members who are accustomed to traditional development approaches or who perceive DDD as overly complex or impractical.
- Overcoming resistance to change requires effective communication, education, and leadership to demonstrate the benefits of DDD and address concerns and skepticism.
- Over-Engineering:
- There is a risk of over-engineering when applying DDD, where teams focus too much on modeling complex domain concepts and introducing unnecessary abstractions or complexity. Striking the right balance between simplicity and expressiveness is crucial to avoid over-complicating the design and implementation.
Use-Cases of Domain-Driven Design (DDD)
- Finance and Banking:
- In the finance sector, DDD can be used to model complex financial instruments, transactions, and regulatory requirements. By accurately representing domain concepts such as accounts, transactions, and portfolios, DDD helps ensure the integrity and consistency of financial systems. It also enables better risk management, compliance, and reporting.
- E-commerce and Retail:
- E-commerce platforms often deal with complex domain concepts such as product catalogs, inventory management, pricing, and customer orders. DDD can help model these concepts effectively, enabling features such as personalized recommendations, dynamic pricing, and streamlined order processing.
- Healthcare and Life Sciences:
- In healthcare, DDD can be used to model patient records, medical diagnoses, treatment plans, and healthcare workflows. By accurately representing domain concepts such as patient demographics, medical histories, and clinical protocols, DDD enables the development of robust electronic health record (EHR) systems, medical imaging platforms, and telemedicine applications.
- Insurance:
- Insurance companies manage diverse products, policies, claims, and underwriting processes. DDD can help model these complex domain concepts, enabling features such as policy management, claims processing, risk assessment, and actuarial analysis.
- Real Estate and Property Management:
- Real estate and property management involve handling diverse properties, leases, tenants, maintenance requests, and financial transactions. DDD can help model these domain concepts effectively, enabling features such as property listings, lease management, tenant portals, and asset tracking.
Real-world Example of Domain-Driven Design (DDD)
Problem Statement
Lets say, we are developing a ride-hailing application called “RideX.” The system allows users to request rides, drivers to accept ride requests, and facilitates the coordination of rides between users and drivers.
Ubiquitous Language
- User: Refers to individuals who request rides via the RideX platform.
- Driver: Refers to individuals who provide rides to users via the RideX platform.
- Ride Request: Represents a user’s request for a ride, specifying details such as pickup location, destination, and ride preferences.
- Ride: Represents a single instance of a ride, including details such as pickup and drop-off locations, fare, and duration.
- Ride Status: Represents the current status of a ride, such as “Requested,” “Accepted,” “In Progress,” or “Completed.”
Bounded Contexts
- Ride Management Context: Responsible for managing the lifecycle of rides, including ride requests, ride assignments to drivers, and ride status updates.
- User Management Context: Handles user authentication, registration, and user-specific features such as ride history and payment methods.
- Driver Management Context: Manages driver authentication, registration, availability status, and driver-specific features such as earnings and ratings.
Entities and Value Objects
- User Entity: Represents a registered user of the RideX platform, with properties like user ID, email, password, and payment information.
- Driver Entity: Represents a registered driver on the RideX platform, with properties like driver ID, vehicle details, and driver status.
- Ride Request Entity: Represents a user’s request for a ride, including properties like request ID, pickup location, destination, and ride preferences.
- Ride Entity: Represents a single instance of a ride, including properties like ride ID, pickup and drop-off locations, fare, and ride status.
- Location Value Object: Represents a geographical location, with properties like latitude and longitude.
Aggregates
- Ride Aggregate: Consists of the Ride Entity as the aggregate root, along with related entities such as Ride Request, User, and Driver entities. The Ride Aggregate encapsulates the logic for managing the lifecycle of a ride, including handling ride requests, assigning drivers, and updating ride status.
Repository
- Ride Repository: Provides methods for querying and storing ride-related entities, such as retrieving ride details, updating ride status, and storing ride-related data in the database.
Services
- Ride Assignment Service: Responsible for assigning available drivers to ride requests based on factors such as driver availability, proximity to pickup location, and user preferences.
- Payment Service: Handles payment processing for completed rides, calculating fares, processing payments, and updating user and driver payment information.
Domain Events
- RideRequestedEvent: Represents an event triggered when a user requests a ride, containing information such as the ride request details and user ID.
- RideAcceptedEvent: Represents an event triggered when a driver accepts a ride request, containing information such as the ride ID, driver ID, and pickup location.
Example Scenario
- User Requesting a Ride: A user requests a ride by providing their pickup location, destination, and ride preferences. RideX creates a new ride request entity and triggers a RideRequestedEvent.
- Driver Accepting a Ride: A driver accepts a ride request from the RideX platform. RideX updates the ride status to “Accepted,” assigns the driver to the ride, and triggers a RideAcceptedEvent.
- Ride In Progress: The user and driver coordinate the ride, with the ride status transitioning from “Accepted” to “In Progress” once the driver reaches the pickup location.
- Ride Completion: After reaching the destination, the ride status is updated to “Completed.” RideX calculates the fare, processes the payment, and updates the user and driver payment information accordingly.
Share your thoughts in the comments
Please Login to comment...