Difference Between var, val, and def Keywords in Scala
Last Updated :
05 Apr, 2024
Scala is a general-purpose, high-level, multi-paradigm programming language. It is a pure object-oriented programming language that also provides support to the functional programming approach. Scala programs can convert to bytecodes and can run on the JVM (Java Virtual Machine). Scala stands for Scalable language. It also provides Javascript runtimes. Scala is highly influenced by Java and some other programming languages like Lisp, Haskell, Pizza, etc. This article focuses on discussing the differences between var, val, and def keywords in Scala.
var Keyword
‘var’ is used to declare mutable variables. Once it is defined, the value of a variable declared with ‘var’ can be reassigned.
Syntax:
var variableName: DataType = value
Example:
Scala
object Main {
def main(args: Array[String]): Unit = {
var age: Int = 25
println(age)
// Reassignment allowed
age = 30
println(age)
}
Output:
After running above it initializes age with the value 25, prints it, then reassigns age to 30 and prints it again. So, the output will be 25 followed by 30.
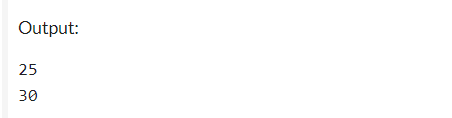
var-keyword-output
val Keyword
Scala’s ‘val’ is similar to a final variable in Java or constants in other languages. It’s also worth remembering that the variable type cannot change in Scala.
‘val’ is used to declare immutable variables. Once it is defined, the value of a variable declared with ‘val’ cannot be changed.
Syntax:
val variableName: DataType = value
Example:
Scala
object Main {
def main(args: Array[String]): Unit = {
val name: String = "Alice"
println(name)
// name = "Bob" // Error: Reassignment to val is not allowed
}
}
Output:
It declares a val named name with the value “Alice”, prints it, and demonstrates that reassignment to val is not allowed by commenting out the reassignment line. When you run this code, it will print Alice as the output.
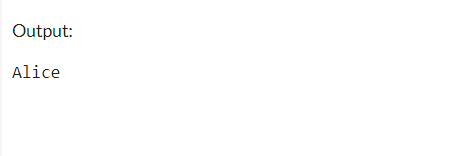
val-keyword-output
def Keyword
‘def’ keyword is used to define methods (functions). Methods defined with ‘def’ are evaluated each time they are called.
Syntax:
def methodName(parameterList): ReturnType = { methodBody }Note
Example:
C++
object Main {
def add(x: Int, y: Int): Int = {
x + y
}
def main(args: Array[String]): Unit = {
val result = add(3, 5)
println("Result: " + result) // Output: Result: 8
}
}
Output:
The above code defines a method add(x: Int, y: Int): Int that returns the sum of two integers, wrapped within an object Main with a main method for execution. When run, it calculates add(3, 5) and prints the result.
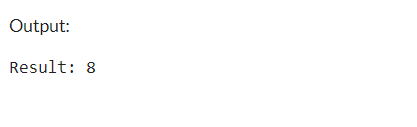
def-keyword-output
Note:
Unlike ‘val’ and ‘var’ , ‘def’ is used for defining functions rather than variables. Also, unlike ‘val’ and ‘var’, ‘def’ doesn’t store a value but rather defines an action to be performed.
Difference between var, val and def Keywords
Features
| ‘var’ Keyword
| ‘val’ Keyword
| ‘def’ Keyword
|
---|
Mutability
| Mutable
| Immutable
| Not Applicable
|
---|
Reassignment
| Allowed
| Not Allowed
| Not Applicable
|
---|
Usage
| For declaring
variables
| For declaring
constants
| For declaring
methods
|
---|
Syntax
| var name: Type = value
| val name: Type = value
| def methodName(params): ReturnType = { body }
|
---|
Memory Allocation
| Allocated at declaration
| Allocated at declaration
| Allocated at invocation
|
---|
Performance
| May incur overhead
| Minimal overhead
| May incur overhead
|
---|
Execution Time
| At each assignment
| Once at declaration
| At each invocation
|
---|
Lazy Evaluation
| Not supported
| Not supported
| Supported with lazy ‘val’
|
---|
Example
| var x: Int = 5
| val y: String = “Hello”
| def add(x: Int, y: Int): Int = { x + y }
|
---|
Conclusion
In short, the ‘val ‘and ‘var’ are evaluated when defined, while ‘def’ is evaluated on call. Also, ‘val’ defines a constant, a fixed value that cannot be modified once declared and assigned while ‘var’ defines a variable, which can be modified or reassigned.
Share your thoughts in the comments
Please Login to comment...