Difference between modes a, a+, w, w+, and r+ in built-in open function?
Last Updated :
25 Sep, 2023
Understanding the file modes in Python’s open() function is essential for working with files effectively. Depending on your needs, you can choose between ‘a’, ‘a+’, ‘w’, ‘w+’, and ‘r+’ modes to read, write, or append data to files while handling files. In this article, we’ll explore these modes and their use cases.
Difference between modes a, a+, w, w+, and r+ in built-in open function?
Python’s built-in open() function is an essential tool for working with files. It allows you to specify how you want to interact with a file by using different modes. Understanding these modes – ‘a’, ‘a+’, ‘w’, ‘w+’, and ‘r+’ – is crucial for efficient file manipulation in Python.
Note – The ‘+’ symbol is often used to specify a mode that allows both reading and writing to a file.
Overview of File Modes
File modes define the purpose for which you want to open a file, whether it’s for reading, writing, or both. Let’s explore each mode in detail:
The test file used is mentioned below:-
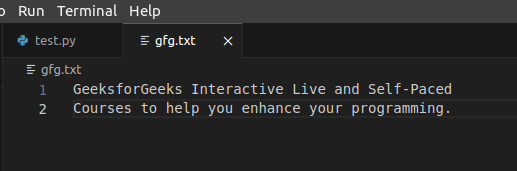
Difference between a and a+ in open()
The ‘r’ is for reading only, while ‘r+’ allows both reading and writing. It’s essential to be cautious when using ‘r+’ to avoid accidentally overwriting or appending data to the wrong part of the file.
Append Mode in Python with ‘a’
In ‘a’ mode, the file is opened for writing, positioned at the end if it exists, creates a new empty file if not, appends data without altering existing content, and disallows reading.
Python3
with open ( 'example.txt' , 'a' ) as file :
file .write( 'Appended text\n' )
|
Output:
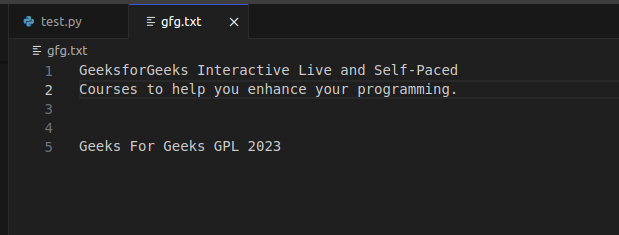
Append and Read Mode in Python with ‘a+’
The ‘a+’ mode opens the file for both reading and writing, positioning the file pointer at the end for writing in existing files and creating a new, empty file if it doesn’t exist.
Python3
with open ( 'example.txt' , 'a+' ) as file :
file .write( 'Appended text\n' )
file .seek( 0 )
content = file .read()
print (content)
|
Output:
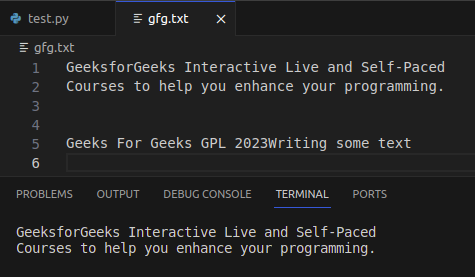
Difference between w and w+ in open()
The ‘w’ is for write-only mode, and ‘w+’ is for both write and read mode. Use ‘w+’ if you need to both write and read data from the file, while ‘w’ is for writing data and overwriting the file’s contents.
Write a file in Python with ‘w’
It opens the file for writing. If the file exists, it truncates (clears) its content. If the file doesn’t exist, Python creates a new, empty file. Reading from the file is not allowed in this mode.
Python3
with open ( 'example.txt' , 'w' ) as file :
file .write( 'This will overwrite existing content' )
|
Output:
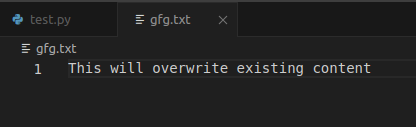
Write and Read Mode in Python with ‘w+’
In ‘w+’ mode, the file is opened for both reading and writing, existing content is cleared, a new empty file is created if it doesn’t exist, and the file pointer is positioned at the beginning.
Python3
with open ( 'example.txt' , 'w+' ) as file :
file .write( 'This will overwrite existing content' )
file .seek( 0 )
content = file .read()
print (content)
|
Output:
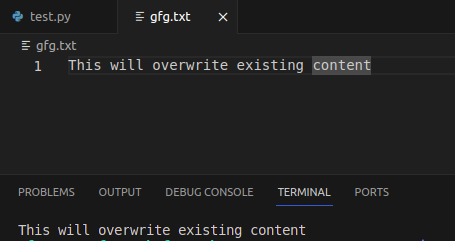
Difference between r and r+ in open()
The ‘r’ is for reading only, and ‘r+’ is for both reading and writing. Be cautious when using ‘r+’ as it can potentially overwrite or modify the existing content of the file.
Read mode in Python with ‘r’
This code segment opens a file named ‘file_r.txt’ in ‘read’ mode (‘r’). It then reads the content of the file using the .read() method and stores it in the variable content. The ‘r’ mode is used for reading files, and it will raise a FileNotFoundError if the file does not exist.
Python3
with open ( 'example.txt' , 'r' ) as file_r:
content = file_r.read()
print ( 'Content in "r":' , content)
|
Output:
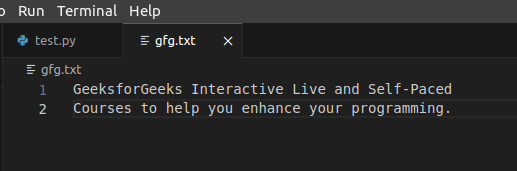
Read and Write Mode in Python with ‘r+’
In ‘r+’ mode, the file is opened for both reading and writing without truncation, and the file pointer is positioned at the beginning. If the file doesn’t exist, a FileNotFoundError is raised.
Python3
with open ( 'example.txt' , 'r+' ) as file :
content = file .read()
print (content)
file .write( 'Appending new content' )
|
Output:
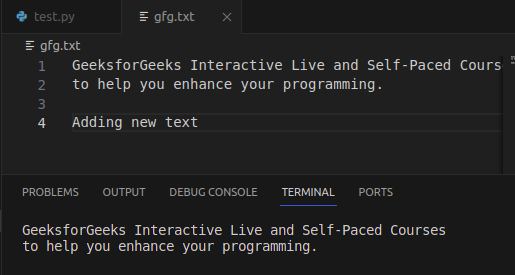
Share your thoughts in the comments
Please Login to comment...