Delete all the even nodes of a Circular Linked List
Last Updated :
06 Sep, 2022
Given a circular singly linked list containing N nodes, the task is to delete all the even nodes from the list.
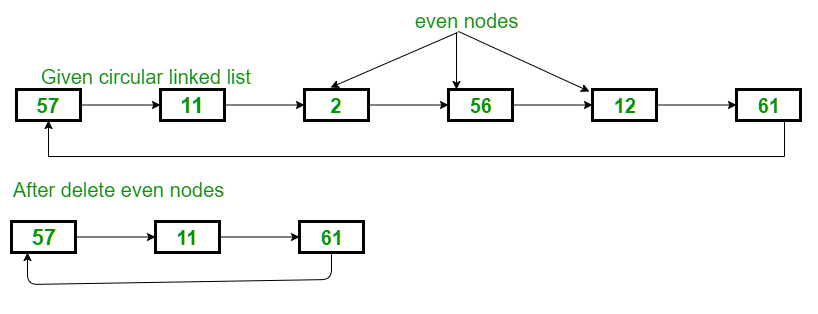
Examples:
Input : 57->11->2->56->12->61
Output : List after deletion : 57 -> 11 -> 61
Input : 9->11->32->6->13->20
Output : List after deletion : 9 -> 11 -> 13
The idea is to traverse the nodes of the circular singly linked list one by one and get the pointer of the nodes having even data. Delete those nodes by following the approach used in this post.
Below is the implementation of the above idea:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
struct Node* next;
};
void push( struct Node** head_ref, int data)
{
struct Node* ptr1 = ( struct Node*) malloc ( sizeof ( struct Node));
struct Node* temp = *head_ref;
ptr1->data = data;
ptr1->next = *head_ref;
if (*head_ref != NULL) {
while (temp->next != *head_ref)
temp = temp->next;
temp->next = ptr1;
}
else
ptr1->next = ptr1;
*head_ref = ptr1;
}
void deleteNode(Node* head_ref, Node* del)
{
struct Node* temp = head_ref;
if (head_ref == del)
head_ref = del->next;
while (temp->next != del) {
temp = temp->next;
}
temp->next = del->next;
free (del);
return ;
}
void deleteEvenNodes(Node* head)
{
struct Node* ptr = head;
struct Node* next;
do {
next = ptr->next;
if (ptr->data % 2 == 0)
deleteNode(head, ptr);
ptr = next;
} while (ptr != head);
}
void printList( struct Node* head)
{
struct Node* temp = head;
if (head != NULL) {
do {
printf ( "%d " , temp->data);
temp = temp->next;
} while (temp != head);
}
}
int main()
{
struct Node* head = NULL;
push(&head, 61);
push(&head, 12);
push(&head, 56);
push(&head, 2);
push(&head, 11);
push(&head, 57);
cout << "\nList after deletion : " ;
deleteEvenNodes(head);
printList(head);
return 0;
}
|
Java
class GFG
{
static class Node
{
int data;
Node next;
};
static Node push(Node head_ref, int data)
{
Node ptr1 = new Node();
Node temp = head_ref;
ptr1.data = data;
ptr1.next = head_ref;
if (head_ref != null )
{
while (temp.next != head_ref)
temp = temp.next;
temp.next = ptr1;
return head_ref;
}
else
ptr1.next = ptr1;
head_ref = ptr1;
return head_ref;
}
static Node deleteNode(Node head_ref, Node del)
{
Node temp = head_ref;
if (head_ref == del)
head_ref = del.next;
while (temp.next != del)
{
temp = temp.next;
}
temp.next = del.next;
return head_ref;
}
static Node deleteEvenNodes(Node head)
{
Node ptr = head;
Node next;
do
{
if (ptr.data % 2 == 0 )
deleteNode(head, ptr);
next = ptr.next;
ptr = next;
}
while (ptr != head);
return head;
}
static void printList(Node head)
{
Node temp = head;
if (head != null )
{
do
{
System.out.printf( "%d " , temp.data);
temp = temp.next;
}
while (temp != head);
}
}
public static void main(String args[])
{
Node head = null ;
head=push(head, 61 );
head=push(head, 12 );
head=push(head, 56 );
head=push(head, 2 );
head=push(head, 11 );
head=push(head, 57 );
System.out.println( "\nList after deletion : " );
head=deleteEvenNodes(head);
printList(head);
}
}
|
Python3
import math
class Node:
def __init__( self , data):
self .data = data
self . next = None
def push(head_ref, data):
ptr1 = Node(data)
temp = head_ref
ptr1.data = data
ptr1. next = head_ref
if (head_ref ! = None ):
while (temp. next ! = head_ref):
temp = temp. next
temp. next = ptr1
else :
ptr1. next = ptr1
head_ref = ptr1
return head_ref
def deleteNode(head_ref, delete):
temp = head_ref
if (head_ref = = delete):
head_ref = delete. next
while (temp. next ! = delete):
temp = temp. next
temp. next = delete. next
def deleteEvenNodes(head):
ptr = head
next = None
next = ptr. next
ptr = next
while (ptr ! = head):
if (ptr.data % 2 = = 0 ):
deleteNode(head, ptr)
next = ptr. next
ptr = next
return head
def printList(head):
temp = head
if (head ! = None ):
print (temp.data, end = " " )
temp = temp. next
while (temp ! = head):
print (temp.data, end = " " )
temp = temp. next
if __name__ = = '__main__' :
head = None
head = push(head, 61 )
head = push(head, 12 )
head = push(head, 56 )
head = push(head, 2 )
head = push(head, 11 )
head = push(head, 57 )
print ( "List after deletion : " , end = "")
head = deleteEvenNodes(head)
printList(head)
|
C#
using System;
class GFG
{
public class Node
{
public int data;
public Node next;
};
static Node push(Node head_ref, int data)
{
Node ptr1 = new Node();
Node temp = head_ref;
ptr1.data = data;
ptr1.next = head_ref;
if (head_ref != null )
{
while (temp.next != head_ref)
temp = temp.next;
temp.next = ptr1;
return head_ref;
}
else
ptr1.next = ptr1;
head_ref = ptr1;
return head_ref;
}
static Node deleteNode(Node head_ref, Node del)
{
Node temp = head_ref;
if (head_ref == del)
head_ref = del.next;
while (temp.next != del)
{
temp = temp.next;
}
temp.next = del.next;
return head_ref;
}
static Node deleteEvenNodes(Node head)
{
Node ptr = head;
Node next;
do
{
if (ptr.data % 2 == 0)
deleteNode(head, ptr);
next = ptr.next;
ptr = next;
}
while (ptr != head);
return head;
}
static void printList(Node head)
{
Node temp = head;
if (head != null )
{
do
{
Console.Write( "{0} " , temp.data);
temp = temp.next;
}
while (temp != head);
}
}
public static void Main(String []args)
{
Node head = null ;
head=push(head, 61);
head=push(head, 12);
head=push(head, 56);
head=push(head, 2);
head=push(head, 11);
head=push(head, 57);
Console.WriteLine( "\nList after deletion : " );
head=deleteEvenNodes(head);
printList(head);
}
}
|
Javascript
<script>
class Node {
constructor() {
this .data = 0;
this .next = null ;
}
}
function push(head_ref , data) {
var ptr1 = new Node();
var temp = head_ref;
ptr1.data = data;
ptr1.next = head_ref;
if (head_ref != null ) {
while (temp.next != head_ref)
temp = temp.next;
temp.next = ptr1;
return head_ref;
} else
ptr1.next = ptr1;
head_ref = ptr1;
return head_ref;
}
function deleteNode(head_ref, del) {
var temp = head_ref;
if (head_ref == del)
head_ref = del.next;
while (temp.next != del) {
temp = temp.next;
}
temp.next = del.next;
return head_ref;
}
function deleteEvenNodes(head) {
var ptr = head;
var next;
do {
if (ptr.data % 2 == 0)
deleteNode(head, ptr);
next = ptr.next;
ptr = next;
} while (ptr != head);
return head;
}
function printList(head) {
var temp = head;
if (head != null ) {
do {
document.write( temp.data+ " " );
temp = temp.next;
} while (temp != head);
}
}
var head = null ;
head = push(head, 61);
head = push(head, 12);
head = push(head, 56);
head = push(head, 2);
head = push(head, 11);
head = push(head, 57);
document.write( "\nList after deletion : " );
head = deleteEvenNodes(head);
printList(head);
</script>
|
Output
List after deletion : 57 11 61
Complexity Analysis:
- Time Complexity: O(N^2)
- As to delete each node we need O(N) time and we have to process every node.
- Auxiliary Space: O(1)
- As constant extra space is used
Share your thoughts in the comments
Please Login to comment...