Dangling Pointer in programming
Last Updated :
14 May, 2024
In programming especially in C and C++, pointers play a very important role in managing memory and improving performance. However incorrect use of pointers can lead to issues. One such issue is creation of dangling pointers. In this article, we will explore what dangling pointers are how they occur and how to prevent them.
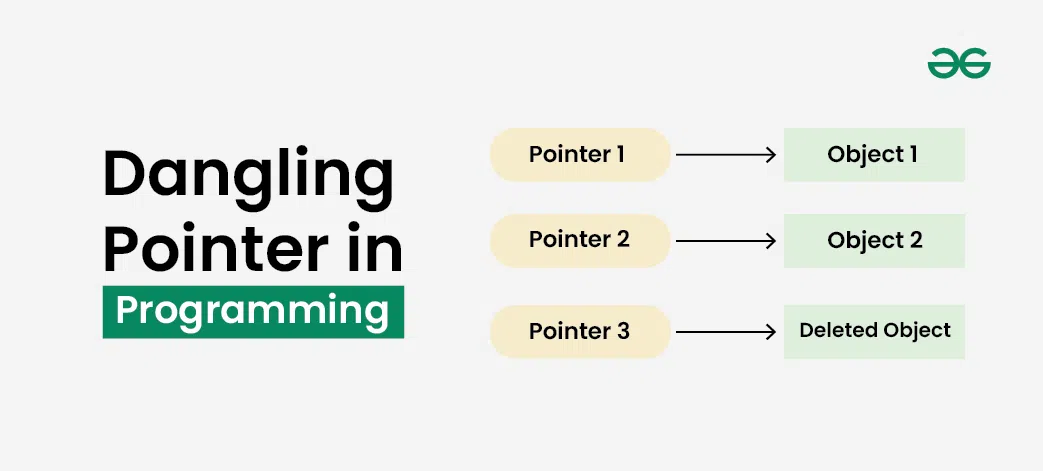
What is Dangling Pointer in Programming?
Dangling Pointer in programming refers to a pointer that doesn’t point to a valid memory location. This usually happens when an object is deleted or deallocated, without modifying the value of the pointer, so it still points to the memory location of the deallocated memory.
Example:
Dangling Pointer Example
int* ptr = new int(5);
delete ptr;
// ptr is now a dangling pointer
Dangling Pointer in C:
The below example demonstrates a simple program that creates a dangling pointer in C.
Example 1
#include <stdio.h>
int main()
{
// Allocating memory
int* ptr = malloc(5 * sizeof(int));
// Deallocating memory
free(ptr);
// ptr is now a dangling pointer
printf("ptr is now a dangling pointer");
return 0;
}
Example 2
#include <stdio.h>
int* ptr;
void gfgFnc()
{
// Allocating memory
int arr[] = { 1, 2, 3, 4, 5 };
ptr = arr;
// Memory gets deallocated automatically outside the
// function
}
int main()
{
gfgFnc();
// ptr is now a dangling pointer
printf("ptr is now a dangling pointer\n");
for (int i = 0; i < 5; i++)
printf("%d ", *(ptr + i));
return 0;
}
Outputptr is now a dangling pointer
Dangling Pointer in C++:
The below example demonstrates a simple program that creates a dangling pointer in C++.
Example 1
// C++ program demonstrating a dangling pointer
#include <iostream>
using namespace std;
int main()
{
// Allocating memory
int* ptr = new int(5);
// Deallocating memory
delete ptr;
// ptr is now a dangling pointer
cout << "ptr is now a dangling pointer" << endl;
return 0;
}
Example 2
#include <iostream>
using namespace std;
int* ptr;
void gfgFnc()
{
// Allocating memory
int arr[] = { 1, 2, 3, 4, 5 };
ptr = arr;
// Memory gets deallocated automatically outside the
// function
}
int main()
{
gfgFnc();
// ptr is now a dangling pointer
cout << "ptr is now a dangling pointer\n";
for (int i = 0; i < 5; i++)
cout << *(ptr + i) << " ";
return 0;
}
Outputptr is now a dangling pointer
Why is it important to handle Dangling Pointers?
Handling dangling pointers is important for maintaining the integrity and stability of a program. Dangling pointers occur when a pointer points to a memory location that has been deallocated or freed. If a program attempts to access or modify the memory through a dangling pointer, it can lead to unpredictable behavior, including crashes and data corruption.
By handling dangling pointers properly, we can improve the reliability and security of the program and prevent any unpredictable access to any memory location.
Preventing Dangling Pointers:
To prevent dangling pointers, always set pointers to NULL or nullptr after you have finished using them and have deallocated the memory they point to. This ensures that the pointers do not point to deallocated memory and become dangling pointers.
Share your thoughts in the comments
Please Login to comment...