Creating and Using Serializers – Django REST Framework
Last Updated :
10 Sep, 2021
In Django REST Framework the very concept of Serializing is to convert DB data to a datatype that can be used by javascript. Serializers allow complex data such as querysets and model instances to be converted to native Python datatypes that can then be easily rendered into JSON, XML or other content types. Serializers also provide deserialization, allowing parsed data to be converted back into complex types, after first validating the incoming data. The serializers in REST framework work very similarly to Django’s Form and ModelForm classes.
To check how to setup Django RESt Framework and create a API visit – How to Create a basic API using Django Rest Framework ?
Creating a basic Serializer
To create a basic serializer one needs to import serializers class from rest_framework and define fields for a serializer just like creating a form or model in Django.
Example
Python3
from rest_framework import serializers
class CommentSerializer(serializers.Serializer):
email = serializers.EmailField()
content = serializers.CharField(max_length = 200 )
created = serializers.DateTimeField()
|
This way one can declare serializer for any particular entity or object based on fields required. Serializers can be used to serialize as well as deserialize the data.
Using Serializer to serialize data
One can now use CommentSerializer to serialize a comment, or list of comments. Again, using the Serializer class looks a lot like using a Form class. Let’s create a Comment class first to create a object of type comment that can be understood by our serializer.
Python3
from datetime import datetime
class Comment( object ):
def __init__( self , email, content, created = None ):
self .email = email
self .content = content
self .created = created or datetime.now()
comment = Comment(email = 'leila@example.com' , content = 'foo bar' )
|
Now that our object is ready, let’s try serializing this comment object. Run following command,
Python manage.py shell
Now run the following code
# import comment serializer
>>> from apis.serializers import CommentSerializer
# import datetime for date and time
>>> from datetime import datetime
# create a object
>>> class Comment(object):
... def __init__(self, email, content, created=None):
... self.email = email
... self.content = content
... self.created = created or datetime.now()
...
# create a comment object
>>> comment = Comment(email='leila@example.com', content='foo bar')
# serialize the data
>>> serializer = CommentSerializer(comment)
# print serialized data
>>> serializer.data
Now let’s check output for this,
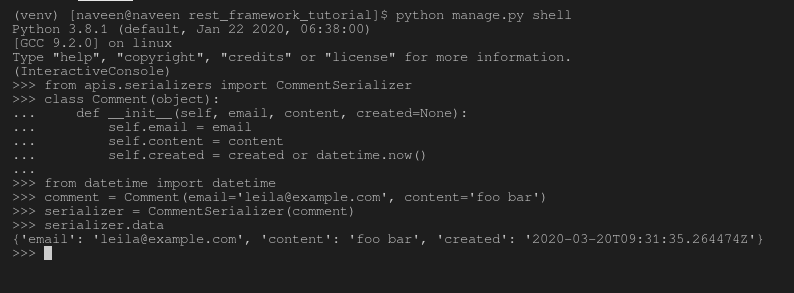
We can convert this data to JSON or XML format using Python’s inbuilt functions or rest framework’s parsers.
Python3
from rest_framework.renderers import JSONRenderer
json = JSONRenderer().render(serializer.data)
|
Using Serializer to deserialize data
Deserialization is similar to Serialization. It means to convert the data from JSON format to a given data type. First we parse a stream into Python native datatypes… (define which datatype to deserialize to….)
First we need to convert this json data back to data that can be understood by the serializer for deserializing,
Python3
import io
from rest_framework.parsers import JSONParser
stream = io.BytesIO(json)
data = JSONParser().parse(stream)
|
and Now let’s deserialize the data back to its original state
Python3
serializer = CommentSerializer(data = data)
serializer.is_valid()
serializer.validated_data
|
Let’s check output and if data has been deserialized –

Share your thoughts in the comments
Please Login to comment...