Creating and updating PowerPoint Presentations in Python using python – pptx
Last Updated :
18 Aug, 2020
python-pptx is library used to create/edit a PowerPoint (.pptx) files. This won’t work on MS office 2003 and previous versions. We can add shapes, paragraphs, texts and slides and much more thing using this library.
Installation: Open the command prompt on your system and write given below command:
pip install python-pptx
Let’s see some of its usage:
Example 1: Creating new PowerPoint file with title and subtitle slide.
Python3
from pptx import Presentation
root = Presentation()
first_slide_layout = root.slide_layouts[ 0 ]
slide = root.slides.add_slide(first_slide_layout)
slide.shapes.title.text = " Created By python-pptx"
slide.placeholders[ 1 ].text = " This is 2nd way"
root.save( "Output.pptx" )
print ( "done" )
|
Output:
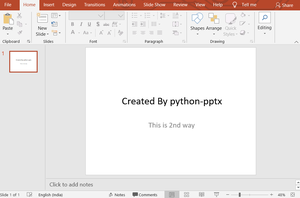
Example 2: Adding Text-Box in PowerPoint.
Python3
from pptx import Presentation
from pptx.util import Inches, Pt
ppt = Presentation()
blank_slide_layout = ppt.slide_layouts[ 6 ]
slide = ppt.slides.add_slide(blank_slide_layout)
left = top = width = height = Inches( 1 )
txBox = slide.shapes.add_textbox(left, top,
width, height)
tf = txBox.text_frame
tf.text = "This is text inside a textbox"
p = tf.add_paragraph()
p.text = "This is a second paragraph that's bold and italic"
p.font.bold = True
p.font.italic = True
p = tf.add_paragraph()
p.text = "This is a third paragraph that's big "
p.font.size = Pt( 40 )
ppt.save( 'test_2.pptx' )
print ( "done" )
|
Output:
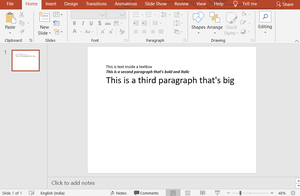
Example 3: PowerPoint (.pptx) file to Text (.txt) file conversion.
Python3
from pptx import Presentation
ppt = Presentation( "sample.pptx" )
File_to_write_data = open ( "File_To_Extract_ppt.txt" , "w" )
for slide in ppt.slides:
for shape in slide.shapes:
if not shape.has_text_frame:
continue
for paragraph in shape.text_frame.paragraphs:
for run in paragraph.runs:
File_to_write_data.write(run.text)
File_to_write_data.close()
print ( "Done" )
|
Output:
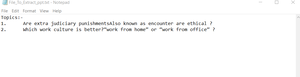
Example 4: Inserting image into the PowerPoint file.
Python3
from pptx import Presentation
from pptx.util import Inches
img_path = 'bg_bg.png'
ppt = Presentation()
blank_slide_layout = ppt.slide_layouts[ 6 ]
slide = ppt.slides.add_slide(blank_slide_layout)
left = top = Inches( 1 )
pic = slide.shapes.add_picture(img_path,
left, top)
left = Inches( 1 )
height = Inches( 1 )
pic = slide.shapes.add_picture(img_path, left,
top, height = height)
ppt.save( 'test_4.pptx' )
print ( "Done" )
|
Output:
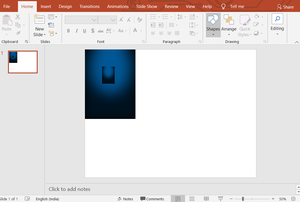
Example 5: Adding Charts to the PowerPoint file.
Python3
from pptx import Presentation
from pptx.chart.data import CategoryChartData
from pptx.enum.chart import XL_CHART_TYPE
from pptx.util import Inches
ppt = Presentation()
slide = ppt.slides.add_slide(ppt.slide_layouts[ 6 ])
chart_data = CategoryChartData()
chart_data.categories = [ 'East' , 'West' , 'Midwest' ]
chart_data.add_series( 'Series 1' ,
( int ( input ( "Enter Value:" )),
int ( input ( "Enter Value:" )),
int ( input ( "Enter Value:" ))))
x, y, cx, cy = Inches( 2 ), Inches( 2 ), Inches( 6 ), Inches( 4.5 )
slide.shapes.add_chart( XL_CHART_TYPE.COLUMN_CLUSTERED, x,
y, cx, cy, chart_data )
ppt.save( 'chart-Tutorial.pptx' )
print ( "done" )
|
Output:
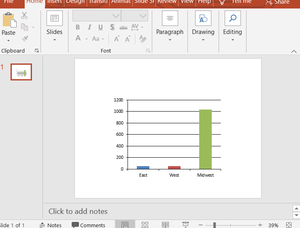
Example 6: Adding tables to the PowerPoint file.
Python3
from pptx import Presentation
from pptx.util import Inches
ppt = Presentation()
slide = ppt.slides.add_slide(ppt.slide_layouts[ 6 ])
x, y, cx, cy = Inches( 2 ), Inches( 2 ), Inches( 4 ), Inches( 1.5 )
shape = slide.shapes.add_table( 3 , 4 , x,
y, cx, cy)
ppt.save( "Tabel_Tutorial.pptx" )
print ( "done" )
|
Output:
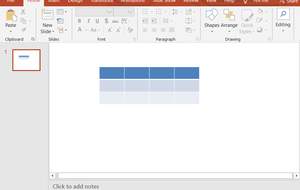
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...