Creating Unique Files with Timestamps in Java
Last Updated :
02 Feb, 2024
Create a file with a specific timestamp in its name in Java using the java.nio.file package along with the java.text and java.util packages for formatting the timestamp.
Difference between SimpleDateFormat and DateTimeFormatter
SimpleDateFormat and DateTimeFormatter are both classes in Java used for formatting and parsing date and time values, but they have some key differences:
- Thread Safety:
- SimpleDateFormat is not thread-safe. If multiple threads are trying to format or parse dates simultaneously using the same instance of SimpleDateFormat, it can lead to unexpected results.
- DateTimeFormatter is designed to be thread-safe. Instances of DateTimeFormatter can be safely shared among multiple threads.
- Immutability:
- SimpleDateFormat is mutable, meaning its state can be modified after creation. This can lead to issues in a multithreaded environment.
- DateTimeFormatter is immutable. Once you create an instance, its state cannot be changed. This makes it safe to use in concurrent scenarios.
- Pattern Letters:
- SimpleDateFormat uses pattern letters that are not always intuitive (e.g., ‘D’ for day of the year, ‘F’ for day of the week in month).
- DateTimeFormatter uses a more comprehensive and consistent set of pattern letters based on the ISO 8601 standard. For example, ‘M’ is always used for month, ‘d’ for day of the month, and ‘E’ for day of the week.
- API Design:
- SimpleDateFormat is part of the older java.text package.
- DateTimeFormatter is part of the newer java.time.format package introduced in Java 8 as part of the java.time API.
- Handling of Locale:
- Both classes allow you to specify a locale, but the way they handle it can differ.
- SimpleDateFormat relies heavily on the default locale of the JVM unless explicitly set.
- DateTimeFormatter allows you to explicitly set the desired locale or use the default locale.
In summary, if you are working with Java 8 or newer, it’s generally recommended to use DateTimeFormatter due to its thread safety, immutability, and improved API design. If you are working with older versions of Java, you may need to use SimpleDateFormat but be cautious about its lack of thread safety.
Example of Creating a File with a Specific Timestamp in its Name
1. Using SimpleDateFormat for Timestamp
Below is the implementation of creating a File with specific Timestamp in its Name:
Java
import java.nio.file.Path;
import java.nio.file.Paths;
import java.text.SimpleDateFormat;
import java.util.Date;
public class CreateFileWithTimestampExample1 {
public static void main(String[] args) {
String directoryPath = "/path/to/directory/" ;
SimpleDateFormat dateFormat = new SimpleDateFormat( "yyyyMMdd_HHmmss" );
String timestamp = dateFormat.format( new Date());
String fileName = "file_" + timestamp + ".txt" ;
String fullPath = directoryPath + fileName;
Path filePath = Paths.get(fullPath);
try {
filePath.toFile().createNewFile();
System.out.println( "File created: " + filePath);
} catch (Exception e) {
e.printStackTrace();
}
}
}
|
Output

Final Result after Execution of Program:
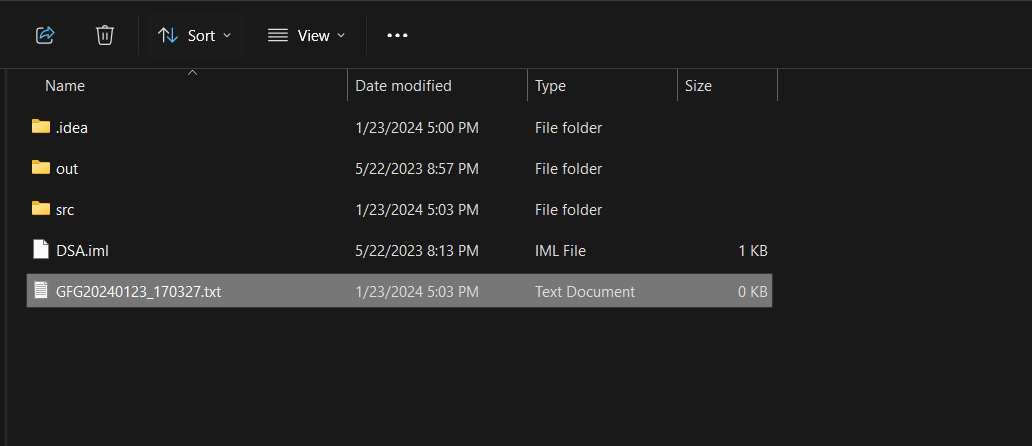
Explaination of the above Program:
This Java program generates a new file with a timestamp incorporated into its name as mentioned below:
- It begins by defining the desired directory path and obtaining the current timestamp using SimpleDateFormat.
- The program then constructs the filename by combining “file_”, the timestamp, and “.txt”.
- It creates the full path by concatenating the directory path and filename.
- Finally, it attempts to create the file using Paths and File classes, printing a success message or handling any exceptions that may arise.
Note: Here, GFG20240123_170327.txt is the filename and rest is the Path where file is created.
2. Using DateTimeFormatter for Timestamp
Below is the implementation of using DateTimeFormatter for Timestamp:
Java
import java.nio.file.Path;
import java.nio.file.Paths;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class CreateFileWithTimestampExample2 {
public static void main(String[] args) {
String directoryPath = "/path/to/directory/" ;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern( "yyyyMMdd_HHmmss" );
String timestamp = LocalDateTime.now().format(formatter);
String fileName = "file_" + timestamp + ".txt" ;
String fullPath = directoryPath + fileName;
Path filePath = Paths.get(fullPath);
try {
filePath.toFile().createNewFile();
System.out.println( "File created: " + filePath);
} catch (Exception e) {
e.printStackTrace();
}
}
}
|
Output: will be same as the above method.
Explaination of the above Program:
- Directory Path:Â It setsÂ
directoryPath
 to store the desired file location.
- Timestamp Generation:Â It employsÂ
DateTimeFormatter
 to format the current date and time, creating a string like “yyyyMMdd_HHmmss” using LocalDateTime.now().format(formatter)
.
- Filename Construction: It combines “file_”, the timestamp, and “.txt” to create a unique filename.
- Full Path Composition:Â It joins the directory path and filename to form a complete file path.
- File Creation Attempt:Â It usesÂ
Paths
 and File
 classes to try creating the file, printing a success message or handling exceptions.
- Key Difference:Â It leveragesÂ
LocalDateTime
 and DateTimeFormatter
 for contemporary date-time handling, offering potential advantages in terms of functionality and readability.
Note: Replace “/path/to/directory/” with the actual path where you want to create the file. These examples use different approaches to format the timestamp, either with SimpleDateFormat (Example 1) or DateTimeFormatter (Example 2). Choose the one that suits your preference or the version of Java you are using (since DateTimeFormatter is introduced in Java 8).
Share your thoughts in the comments
Please Login to comment...