Create an Autoplay Carousel using HTML CSS and JavaScript
Last Updated :
28 Sep, 2023
Creating an autoplay carousel using HTML, CSS, and JavaScript is a great way to showcase multiple images or content in a visually appealing way. In this article, we’ll walk through the steps to create such a carousel. We’ll also provide a live example and instructions on how to run the application.
In this article, we will create an autoplay carousel that displays colorful content slides. The carousel will automatically transition between slides at regular intervals.
Prerequisites:
Approach
- HTML: Make a “carousel” container with “carousel-item” elements for content.
- CSS: Style container dimensions, background, and item appearance.
- JavaScript: Manage “active” class, set an interval for auto-rotation (e.g., every 3.5 seconds), and switch items. You can also customize content and adjust the interval for desired behavior. Test and refine as needed.
Example: This example describes the basic implementation of the the autoplay carousel using HTML, CSS, and JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Autoplay Carousel</ title >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< div class = "carousel" >
< div class = "carousel-item" >
< div class = "slide-image"
style="background-image:
</ div >
</ div >
< div class = "carousel-item" >
< div class = "slide-image"
style="background-image:
</ div >
</ div >
< div class = "carousel-item" >
< div class = "slide-image"
style="background-image:
</ div >
</ div >
< div class = "carousel-item" >
< div class = "slide-image"
style="background-image:
</ div >
</ div >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
* {
box-sizing: border-box;
}
body {
display : flex;
justify- content : center ;
align-items: center ;
}
.carousel {
position : relative ;
width : 270px ;
height : 160px ;
overflow : hidden ;
background-color : #cdcdcd ;
}
.carousel-item .slide-image {
width : 270px ;
height : 160px ;
background- size : cover;
background-repeat : no-repeat ;
}
.carousel-item {
position : absolute ;
width : 100% ;
height : 270px ;
border : none ;
top : 0 ;
left : 100% ;
}
.carousel-item.active {
left : 0 ;
transition: all 0.3 s ease-out;
}
.carousel-item div {
height : 100% ;
}
. red {
background-color : red ;
}
. green {
background-color : green ;
}
.yellow {
background-color : yellow;
}
.violet {
background-color : violet;
}
|
Javascript
window.onload = function () {
let slides =
document.getElementsByClassName( 'carousel-item' );
function addActive(slide) {
slide.classList.add( 'active' );
}
function removeActive(slide) {
slide.classList.remove( 'active' );
}
addActive(slides[0]);
setInterval( function () {
for (let i = 0; i < slides.length; i++) {
if (i + 1 == slides.length) {
addActive(slides[0]);
setTimeout(removeActive, 350, slides[i]);
break ;
}
if (slides[i].classList.contains( 'active' )) {
setTimeout(removeActive, 350, slides[i]);
addActive(slides[i + 1]);
break ;
}
}
}, 1500);
};
|
Output:
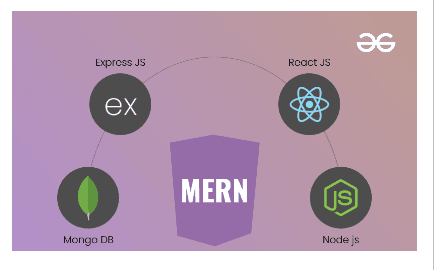
Output
Share your thoughts in the comments
Please Login to comment...