Create a User Polls Project using HTML CSS & JavaScript
Last Updated :
28 Dec, 2023
Creating a user polls project has various valuable purposes, including gathering feedback, engaging users, personalizing content, fostering community, conducting research, A/B testing, and improving the user experience. Clear objectives are crucial, and in this example, we focus on collecting data on users’ preferences for programming. In this article, we will explore how to implement a user polls project using HTML, CSS, and JavaScript.
Prerequisites:
Preview Image:
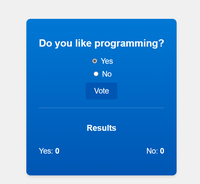
Output Image
Approach
We have a clear set of observations to guide the thorough implementation of this project. Let’s begin by creating the basic HTML structure based on the project description we have discussed above.
- There are two radio buttons for user input.
- At the top, there is a paragraph presenting the question.
- There is a button with the value attribute set to ‘vote.’
- At the bottom, there are results displayed for ‘Yes’ and ‘No,’ along with their respective counts.
- We can notice that the background color is distinct.
- The position is centered, and text elements are aligned to the center.
- In this project, when we click the ‘Vote’ button, the values of ‘Yes’ and ‘No’ should either increase or remain the same, depending on the user’s choice.
- To achieve this, we will utilize the getElementById method in JavaScript, which will allow us to increment the counters for ‘Yes’ and ‘No’ accordingly.
- Subsequently, the updated counts will be reflected in the output.
Example: Below is the basic implementation of the above approach.
Javascript
document.addEventListener( "DOMContentLoaded" , function () {
const pollForm =
document.getElementById( "poll-form" );
const yesCount =
document.getElementById( "yes-count" );
const noCount =
document.getElementById( "no-count" );
let yesVotes = 0;
let noVotes = 0;
pollForm.addEventListener( "submit" , function (e) {
e.preventDefault();
const formData = new FormData(pollForm);
const userVote = formData.get( "vote" );
if (userVote === "yes" ) {
yesVotes++;
} else if (userVote === "no" ) {
noVotes++;
}
updateResults();
});
function updateResults() {
yesCount.textContent = yesVotes;
noCount.textContent = noVotes;
}
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >User Polls</ title >
< link rel = "stylesheet" href = "./style.css" >
</ head >
< body >
< div class = "container" >
< h1 class = "poll-question" >
Do you like programming?
</ h1 >
< form id = "poll-form" class = "poll-form" >
< label >
< input type = "radio"
name = "vote"
value = "yes" > Yes
</ label >
< label >
< input type = "radio"
name = "vote"
value = "no" > No
</ label >
< button type = "submit"
class = "vote-button" >
Vote
</ button >
</ form >
< div id = "results" class = "results" >
< h2 class = "results-title" >
Results
</ h2 >
< div class = "results-count" >
< p >Yes:
< span id = "yes-count"
class = "count" >0
</ span >
</ p >
< p >No:
< span id = "no-count"
class = "count" >0
</ span >
</ p >
</ div >
</ div >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
background-color : #f0f0f0 ;
margin : 0 ;
padding : 0 ;
display : flex;
justify- content : center ;
align-items: center ;
min-height : 100 vh;
}
.container {
background : linear-gradient(to bottom , #0078d4 , #0056b3 );
border-radius: 10px ;
box-shadow: 0 4px 6px rgba( 0 , 0 , 0 , 0.1 );
color : #fff ;
text-align : center ;
padding : 30px ;
}
.poll-question {
font-size : 24px ;
margin-bottom : 20px ;
}
.poll-form label {
display : block ;
margin-bottom : 10px ;
font-size : 18px ;
color : #fff ;
}
.vote-button {
background-color : #0056b3 ;
color : #fff ;
border : none ;
padding : 10px 20px ;
cursor : pointer ;
font-size : 18px ;
border-radius: 5px ;
transition: background-color 0.3 s ease-in-out;
}
.vote-button:hover {
background-color : #0078d4 ;
}
.results {
margin-top : 20px ;
padding-top : 20px ;
border-top : 1px solid rgba( 255 , 255 , 255 , 0.3 );
}
.results-title {
font-size : 20px ;
}
.results-count {
display : flex;
justify- content : space-between;
font-size : 18px ;
margin-top : 10px ;
}
.count {
font-weight : bold ;
}
|
Output:
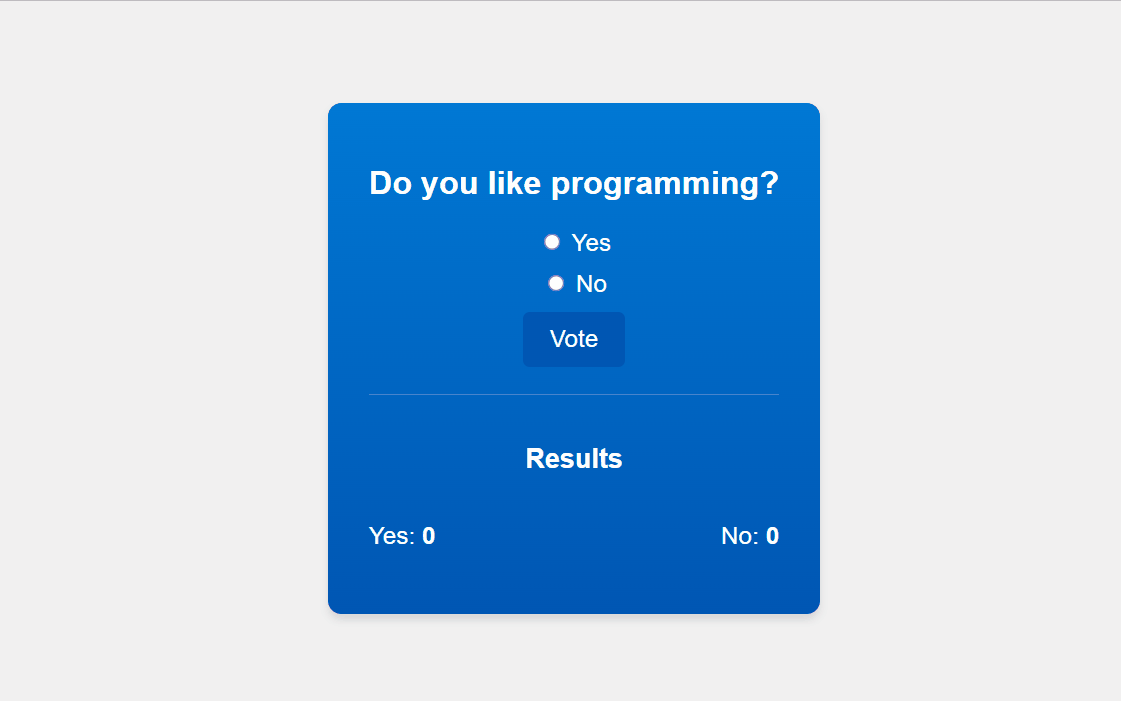
Output
Share your thoughts in the comments
Please Login to comment...