Create a Text Narrator App using React-Native
Last Updated :
20 Nov, 2023
In this project, we’ll develop a Text Narrator application using React Native. The Text Narrator app is a valuable tool for improving accessibility. It allows users to input text, and the app will convert that text into audible speech. This can be incredibly helpful for individuals with visual impairments or reading difficulties, making it easier for them to consume written information.
Preview of final output: Let us have a look at how the final output will look like.
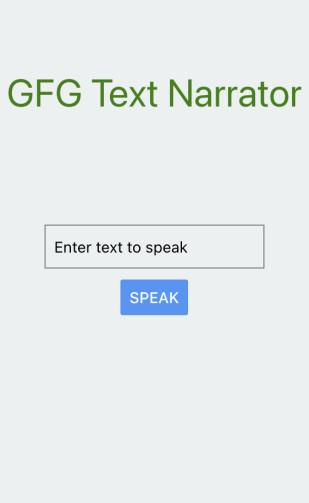
Prerequisites
Approach
- Text Input: Users can input the text they want to hear.
- Speech Feature: The app will use the `expo-speech` library to convert the text into audible speech.
- User-Friendly Interface: The app will have a simple and user-friendly design.
Steps to Create & Configure React Native App:
Step 1: Create a new React Native project.
npx react-native init TextEditorApp
Step2: Navigate to the project directory:
cd TextEditorApp
Step 3: Install ‘expo-speech‘
npm i expo-speech
Project Structure
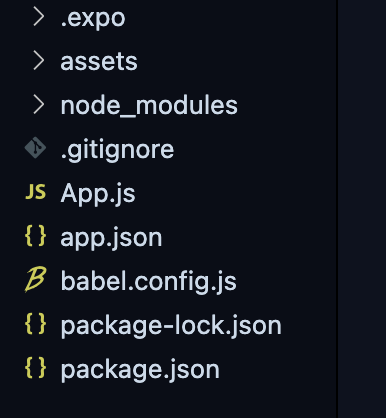
The updated dependencies in the package.json file will look like:
"dependencies": {
"react-native-paper": "4.9.2",
"@expo/vector-icons": "^13.0.0",
"expo-speech": "~11.1.1"
}
Example: Implementation of above approach in the App.js file.
Javascript
import * as React from 'react' ;
import { View, StyleSheet, Button, TextInput, Text } from 'react-native' ;
import * as Speech from 'expo-speech' ;
export default function App() {
const [textToSpeak, setTextToSpeak] = React.useState( '' );
const speak = () => {
Speech.speak(textToSpeak);
}
return (
<View style={styles.container}>
<Text style={styles.gfg}>GFG Text Narrator</Text>
<TextInput style={styles.input}
placeholder= "Enter text to speak"
onChangeText={setTextToSpeak}
value={textToSpeak} />
<Button title= "Speak" onPress={speak} />
</View>
)
}
const styles = StyleSheet.create(
{
container: {
height: "100%" ,
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
backgroundColor: '#ecf0f1' ,
padding: 8,
paddingTop: 80,
marginTop: 10
},
gfg: {
marginBottom: 100,
color: "green" ,
fontSize: 35,
},
input: {
height: 40,
width: 200,
borderColor: 'gray' ,
borderWidth: 1,
marginBottom: 10,
paddingHorizontal: 8,
},
});
|
Steps to Run Application:
Step 1: To run the app in an iOS simulator or on a connected iOS device:
npx react-native run-ios
Step 2 :To run the app in an Android emulator or on a connected Android device:
npx react-native run-android
Output
.gif)
Final output
Share your thoughts in the comments
Please Login to comment...