C++ Program to Print Christmas Tree Using Pyramid
Last Updated :
24 Oct, 2023
This program prints a Christmas tree with a specified height and width. It consists of two main parts: the upper part that forms the tree’s triangular shape and the lower part that represents the tree trunk.
Approach to Print Christmas Tree
Christmas tree creation is a complex task but breaking the tree into parts can make it a bit easy. The parts broken are mentioned below:
Printing Upper Part (Pyramids):
- A loop iterates through each row of the pyramid.
- Inner loops are used to manage spaces and asterisks for each row.
- The first inner loop handles printing spaces before the asterisks to center them.
- The second inner loop prints the asterisks in a pyramid pattern.
Printing Lower Part (Branch):
- Another loop is used to print the lower part of the tree, which represents the tree trunk.
- Similar to the upper part, inner loops are used to manage spaces and print asterisks.
Program to Print Christmas Tree Using Pyramid
Below is the implementation of the topic mentioned above:
C++
#include <iostream>
using namespace std;
class PrintChristmasTree {
public :
static const int height = 5;
static int main()
{
int width = 5;
int space = width * 5;
int x = 1;
for ( int a = 1; a <= height; a++) {
for ( int i = x; i <= width; i++) {
for ( int j = space; j >= i; j--)
cout << " " ;
for ( int k = 1; k <= i; k++)
cout << "* " ;
cout << endl;
}
x = x + 2;
width = width + 2;
}
for ( int i = 1; i <= 4; i++) {
for ( int j = space - 3; j >= 1; j--)
cout << " " ;
for ( int k = 1; k <= 4; k++)
cout << "* " ;
cout << endl;
}
return 0;
}
};
int main()
{
PrintChristmasTree::main();
return 0;
}
|
Output:
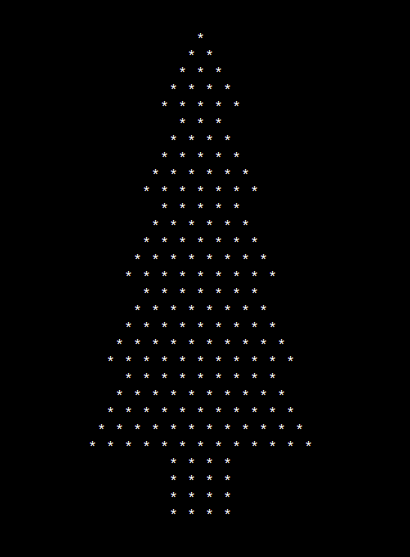
Complexity of the Above Method:
Time complexity: O(h*w*w) for given height h and width w
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...