An array is a type of data structure used to store the collection of the same data type items held at contiguous memory locations. Arrays can be one-dimensional or multidimensional based on the number of directions in which the array can grow. In this article, we will study multidimensional arrays such as two-dimensional arrays and three-dimensional arrays.
What is Multidimensional Array in C++?
A multidimensional array is an array with more than one dimension. It is the homogeneous collection of items where each element is accessed using multiple indices.
Multidimensional Array Declaration
datatype arrayName[size1][size2]...[sizeN];
where,
- datatype: Type of data to be stored in the array.
- arrayName: Name of the array.
- size1, size2,…, sizeN: Size of each dimension.
Example:
Two dimensional array: int two_d[2][4];
Three dimensional array: int three_d[2][4][8];
Size of a Multidimensional Array
The size of an array is equal to the size of the data type multiplied by the total number of elements that can be stored in an array. We can calculate the total number of elements in an array by multiplying the size of each dimension of a multidimensional array.
For example:
int arr1[2][4];
- The array int arr1[2][4] can store total (2*4) = 8 elements.
- In C++ int data type takes 4 bytes and we have 8 elements in the array ‘arr1’ of the int type.
- Total size = 4*8 = 32 bytes.
int arr2[2][4][8];
- Array int arr2[2][4][8] can store total (2*4*8) = 64 elements.
- The Total size of ‘arr2‘ = 64*4 = 256 bytes.
To verify the above calculation we can use sizeof() method to find the size of an array.
C++
#include <iostream>
using namespace std;
int main()
{
int arr1[2][4];
int arr2[2][4][8];
cout << "Size of array arr1: " << sizeof (arr1)
<< " bytes" << endl;
cout << "Size of array arr2: " << sizeof (arr2)
<< " bytes" ;
return 0;
}
|
Output
Size of array arr1: 32 bytes
Size of array arr2: 256 bytes
The most widely used multidimensional arrays are:
- Two Dimensional Array
- Three Dimensional Array
Two Dimensional Array (or 2D Array)
A two-dimensional array in C++ is a collection of elements organized in rows and columns. It can be visualized as a table or a grid, where each element is accessed using two indices: one for the row and one for the column. Like a one-dimensional array, two-dimensional array indices also range from 0 to n-1 for both rows and columns.
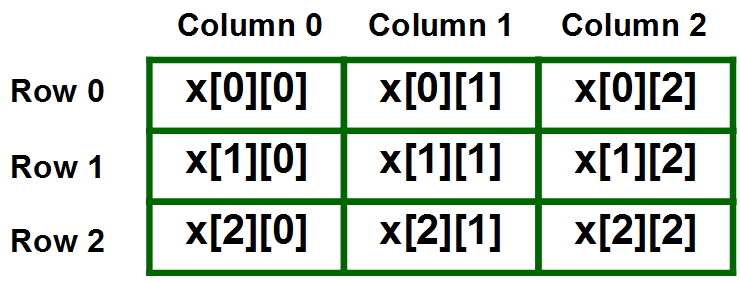
Syntax of 2D array
data_Type array_name[n][m];
Where,
- n: Number of rows.
- m: Number of columns.
We can declare a 2D array statically and dynamically. In static declaration, memory is allocated during compile time, and in dynamic memory is allocated during runtime. The above is the syntax for the static declaration of a 2D array. To know how to declare the 2d array dynamically, refer to this article.
Initialization of Two-Dimensional Arrays in C++
Different ways to initialize a 2D array are given below:
- Using Initializer List
- Using Loops
1. Initialize 2D array using the Initializer list
We can initialize a 2D array using an initializer list in two ways. Below is the first method of initializing a 2D array using an initializer list.
First Method: The below array has 2 rows and 4 columns. The elements are filled in a way that the first 4 elements are filled in the first row and the next 4 elements are filled in the second row.
int arr[2][4] = {0, 1, 2, 3, 4, 5, 6, 7};
Second Method: The below way is the cleaner way to initialize a 2D array the nested list represents the elements in a row and the number of elements inside it is equal to the number of columns in a 2D array. The number of nested lists represents the number of columns.
int x[2][4] = {{0, 1, 2, 3}, {4, 5, 6, 7}};
2. Initialization of 2D array using Loops
We can also initialize 2D array using loops. To initialize 2D array we have to use two nested loops and nested loops are equal to the dimension. For example, to initialize a 3D array we have to use three nested loops. Let’s see an example.
Example: In the below example we have initializes the 2D array with 1. The outer loop is used to track rows “i=0” means the first row because of 0 indexing similarly “j=0” means the first column and combining this x [0][0] represents the first cell of the 2D array.
int x[2][4];
for(int i = 0; i < 2; i++){
for(int j = 0; j < 4; j++){
x[i][j] = 1;
}
}
Accessing Elements of Two-Dimensional Arrays in C++
We can access the elements of a 2-dimensional array using row and column indices. It is similar to matrix element position but the only difference is that here indexing starts from 0.
Syntax:
array_name[i][j];
where,
- i: Index of row.
- j: Index of the column.
Example: Below is the index of elements of the second row and third column.
int x[1][2];
Let’s understand this using code by printing elements of a 2D array.
Example of 2D Array
C++
#include <iostream>
using namespace std;
int main()
{
int count = 1;
int array1[3][4];
for ( int i = 0; i < 3; i++) {
for ( int j = 0; j < 4; j++) {
array1[i][j] = count;
count++;
}
}
for ( int i = 0; i < 3; i++) {
for ( int j = 0; j < 4; j++) {
cout << array1[i][j] << " " ;
}
cout << endl;
}
return 0;
}
|
Output
1 2 3 4
5 6 7 8
9 10 11 12
Explanation: In the above code we have initialized the count by ‘1’ and declared a 2D array with 3 rows and 4 columns after that we initialized the array with the value of count and increment value of count in every iteration of the loop. Then we are printing the 2D array using a nested loop and we can see in the below output that there are 3 rows and 4 columns.
Time Complexity: O(n*m)
Space Complexity:O(n*m)
where n is the number of rows and m is the number of columns.
Three-Dimensional Array in C++
The 3D array is a data structure that stores elements in a three-dimensional cuboid-like structure. It can be visualized as a collection of multiple two-dimensional arrays stacked on top of each other. Each element in a 3D array is identified by its three indices: the row index, column index, and depth index.
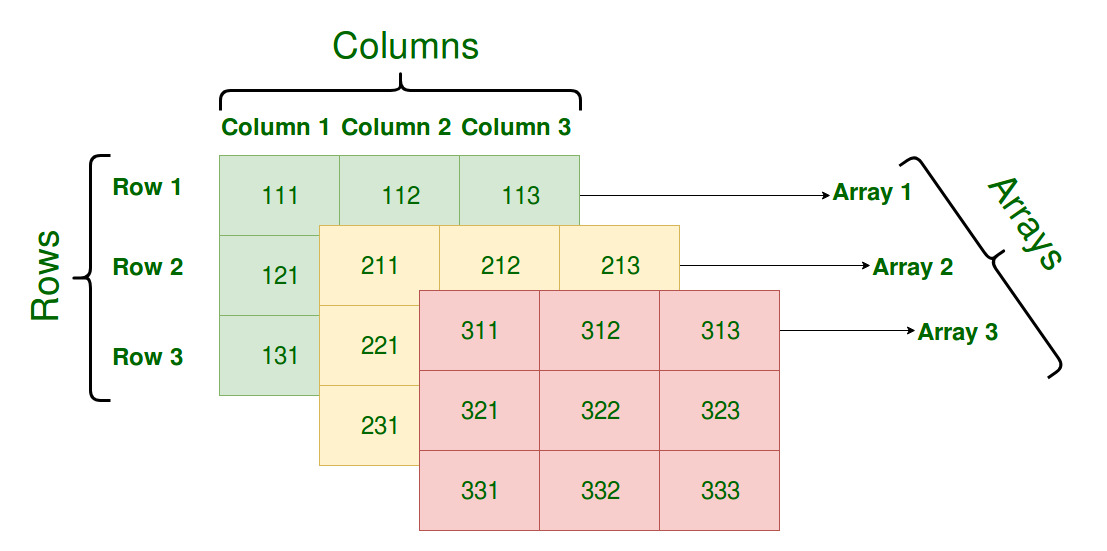
Declaration of Three-Dimensional Array in C++
To declare a 3D array in C++, we need to specify its third dimension along with 2D dimensions.
Syntax:
dataType arrayName[d][r];
- dataType: Type of data to be stored in each element.
- arrayName: Name of the array
- d: Number of 2D arrays or Depth of array.
- r: Number of rows in each 2D array.
- c: Number of columns in each 2D array.
Example:
int array[3][5][2];
Initialization of Three-Dimensional Array in C++
To initialize the 3D array in C++, we follow the same methods we have used to initialize the 2D array. In 3D array, we have one more dimension so we have to add one more nested list of elements.
A 3D array in C can be initialized by using:
- Initializer List
- Loops
Initialization of 3D Array using Initializer List
Method 1: In this method, we have to write the total number of elements inside curly braces, and each item is placed at its position according to the dimension given.
int x[3][5][2] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
10, 11, 12, 13, 14, 15, 16, 17, 18, 19,
20, 21, 22, 23, 24, 25, 26, 27, 28, 30};
Method 2 (Better): In this method, we have partitioned the elements using nested lists and it is easy to read.
int x[3][5][2] = {
{ {0, 1}, {2, 3}, {4, 5}, {6, 7}, {8, 9} },
{ {10, 11}, {12, 13}, {14, 15}, {16, 17}, {18, 19} },
{ {20, 21}, {22, 23}, {24, 25}, {26, 27}, {28, 30} },
};
Initialization of 3D Array using Loops
This method is the same as initializing a 2D array using loops with one more nested loop for the third dimension.
int x[3][5][2];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 5; j++) {
for (int k = 0; k < 2; k++) {
x[i][j][k] = (some_value);
}
}
}
Accessing elements in Three-Dimensional Array in C++
Accessing elements in 3D arrays is as simple as accessing elements in 2D arrays. Here what we have to do extra work is adding one more nested loop to keep track of the third dimension.
C++
#include <iostream>
using namespace std;
int main()
{
int count = 0;
int x[2][2][3];
for ( int i = 0; i < 2; i++) {
for ( int j = 0; j < 2; j++) {
for ( int k = 0; k < 3; k++) {
x[i][j][k] = count;
count++;
}
}
}
for ( int i = 0; i < 2; i++) {
for ( int j = 0; j < 2; j++) {
for ( int k = 0; k < 3; k++) {
printf ( "x[%d][%d][%d] = %d \n" , i, j, k,
x[i][j][k]);
count++;
}
}
}
return 0;
}
|
Output
x[0][0][0] = 0
x[0][0][1] = 1
x[0][0][2] = 2
x[0][1][0] = 3
x[0][1][1] = 4
x[0][1][2] = 5
x[1][0][0] = 6
x[1][0][1] = 7
x[1][0][2] = 8
x[1][1][0] = 9
x[1][1][1] = 10
x[1][1][2] = 11
Explanation: In the above code, we have initialized the 3D array using the loop as explained above with the number from 0 to 7 using the count variable and then accessing the elements using the same loop used for initializing the 3D array. The only difference is that instead of assigning an element at a particular position say “x[0][0][1]=1” we are printing the element stored at that location as seen in the below output.
Share your thoughts in the comments
Please Login to comment...