C++ Dereferencing
Last Updated :
21 Jan, 2023
Prerequisites:
Pointers are symbolic representations of addresses. They enable programs to simulate call-by-reference as well as to create and manipulate dynamic data structures. Iterating over elements in arrays or other data structures is one of the main use of pointers. Pointers and references work simultaneously together, where the pointer uses references to store the address of another variable.
Syntax:
datatype *var_name;
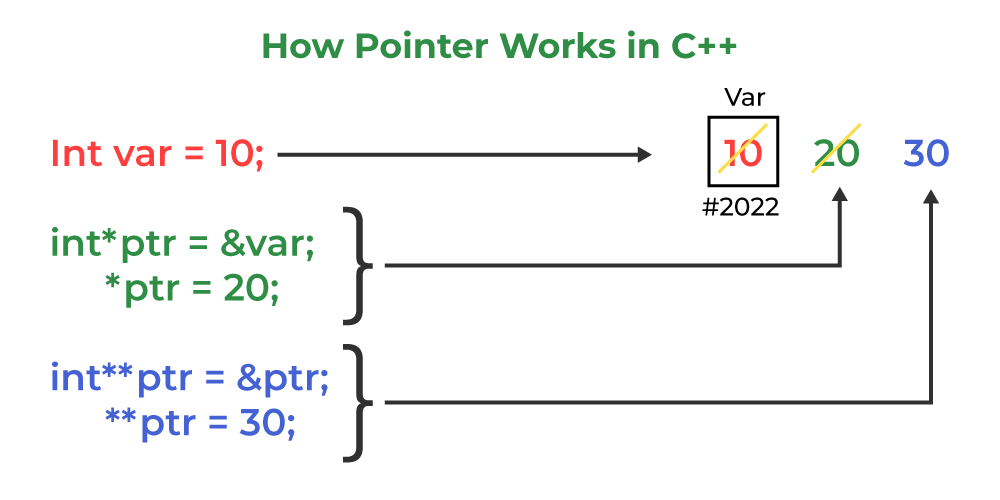
Pointer Working shown in the Image
Dereference Operator
Dereferencing is the method where we are using a pointer to access the element whose address is being stored. We use the * operator to get the value of the variable from its address.
Example:
int a;
// Referencing
int *ptr=&a;
// Dereferencing
int b=*ptr;
Below is the implementation of the above example:
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int a = 10, b = 20;
int * pt;
pt = &a;
cout << "The address where a is stored is: " << pt
<< endl;
cout << "The value stored at the address by "
"dereferencing the pointer is: "
<< *pt << endl;
}
|
Output
The address where a is stored is: 0x7ffdcae26a0c
The value stored at the address by dereferencing the pointer is: 10
Example : We Can Directly assign value to a pointer using Dereference :
C++
#include <iostream>
using namespace std;
int main() {
int *p;
*p = 80;
cout << *p<<endl;
return 0;
}
|
In Above code we directly assign value to pointer p using asterisk (*) and using dereference we print its pointed value that is 80.
Changing the value of the Pointer
There are 2 methods to change the value of pointers
- Change the address of the stored variable
- Change the value in the stored variable
When do you want to assign another address to the pointer?
One of the functionalities of the pointer is that it can store some other variable address. If we want to change the address to another variable in that case we can just reference the variable to another variable.
Example:
C++
#include <iostream>
using namespace std;
int main()
{
int a = 10, b = 20;
int * pt;
pt = &a;
cout << "The address where a is stored is: " << pt
<< endl;
cout << "The value stored at the address by "
"dereferencing the pointer is: "
<< *pt << endl;
pt = &b;
cout << "Pointer is now pointing at: " << pt << endl;
cout << "New value the pointer is pointing to is: "
<< *pt << endl;
return 0;
}
|
Output
The address where a is stored is: 0x7ffe14972128
The value stored at the address by dereferencing the pointer is: 10
Pointer is now pointing at: 0x7ffe1497212c
New value the pointer is pointing to is: 20
When you want to change the value of the variable by dereference operator?
In a few conditions, we can change the value of the actual variable when we need it rather than referencing another variable. In this, case we can just the dereferenced value to make actual changes.
Example:
int a=5;
// Referencing
int *ptr=&a;
// Dereferencing and changing value
*ptr=6;
Below is the implementation of the above example:
C++
#include <iostream>
using namespace std;
int main()
{
int a = 5, b = 6;
int * pt;
pt = &a;
cout << "The address where a is stored is: " << pt
<< endl;
cout << "The value stored at the address by "
"dereferencing the pointer is: "
<< *pt << endl;
*pt = b;
cout << "Pointer is still pointing at: " << pt << endl;
cout << "The new value stored at the address by "
"dereferencing the pointer is: "
<< *pt << endl;
cout << "Now the value of a is: " << a << endl;
return 0;
}
|
Output
The address where a is stored is: 0x7ffc6d36859c
The value stored at the address by dereferencing the pointer is: 5
Pointer is still pointing at: 0x7ffc6d36859c
The new value stored at the address by dereferencing the pointer is: 6
Now the value of a is: 6
Using Array with Pointers
An array is the collection of elements with similar data types, also the memory blocks where elements are continuous. We can use ptr to point to the elements of the array. Let’s check how:
Example:
int arr=[1,2,3,4,5];
// Referencing with array
int *ptr=arr;
// Dereferencing the array
// 1 will be printed
cout<< *ptr <<" ";
Below is the implementation of the above example:
C++
#include <iostream>
using namespace std;
int main()
{
int arr[] = { 1, 2, 3, 4, 5 };
int * ptr = arr;
cout << "Elements of array:" ;
for ( int i = 0; i < 5; i++) {
cout << *(ptr + i) << " " ;
}
cout << endl;
return 0;
}
|
Output
Elements of array:1 2 3 4 5
Share your thoughts in the comments
Please Login to comment...