How to Convert a HashSet to JSON in Java?
Last Updated :
09 Feb, 2024
In Java, a HashSet is an implementation of the Set interface that uses a hash table to store elements. It allows fast lookups and does not allow duplicate elements. Elements in a HashSet are unordered and can be of any object type. In this article, we will see how to convert a HashSet to JSON in Java.
Steps to convert a HashSet to JSON in Java
Below are the steps and implementation of converting a HashSet to JSON in Java with Jackson.
Step 1: Create a Maven Project
Open any preferred IDE and Create a new Maven project. Here, we are using IntelliJ IDEA. So, we can do this by selecting File -> New -> Project.. -> Maven and following the wizard.
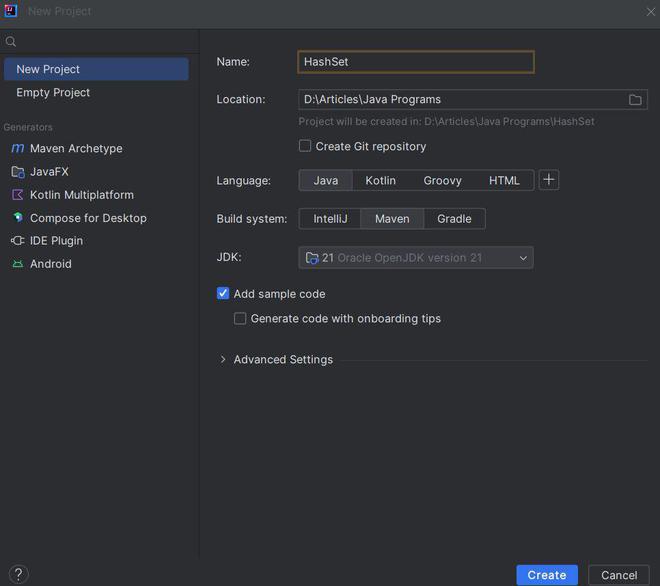
Project Structure:
Below is the project structure that we have created.
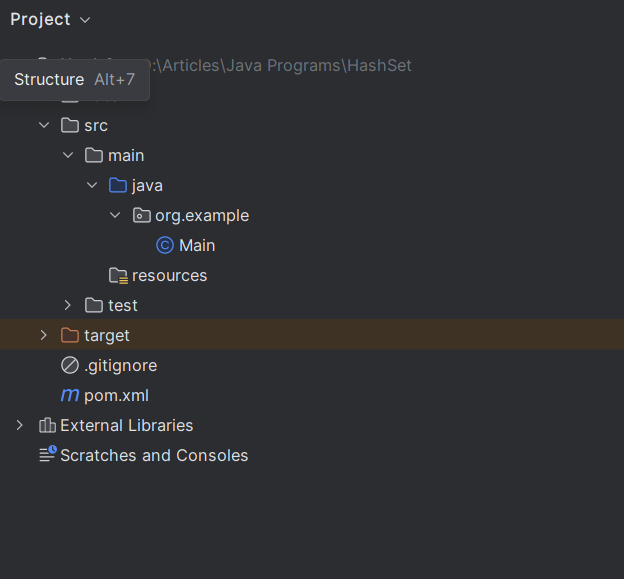
Step 2: Add Jackson Dependency to the pom.xml
Now, we will add Jackson dependency to pom.xml file.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >org.example</ groupId >
< artifactId >HashSet</ artifactId >
< version >1.0-SNAPSHOT</ version >
< dependencies >
< dependency >
< groupId >com.fasterxml.jackson.core</ groupId >
< artifactId >jackson-databind</ artifactId >
< version >2.13.0</ version >
</ dependency >
</ dependencies >
< properties >
< maven.compiler.source >21</ maven.compiler.source >
< maven.compiler.target >21</ maven.compiler.target >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
</ properties >
</ project >
|
Step 3: Add logic of Conversion of a HashSet to JSON String
Below is the implementation to convert a HashSet to JSON with Jackson.
Java
package org.example;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
import java.util.HashSet;
public class Main {
public static void main(String[] args)
{
HashSet<String> courses = new HashSet<>();
courses.add( "Java" );
courses.add( "Python" );
courses.add( "C++" );
ObjectMapper mapper = new ObjectMapper();
try {
ObjectWriter writer = mapper.writerWithDefaultPrettyPrinter();
String jsonData = writer.writeValueAsString(courses);
System.out.println(jsonData);
}
catch (JsonProcessingException e) {
e.printStackTrace();
}
}
}
|
Explanation of the above code:
- It creates a HashSet of courses – Java, Python, C++
- Jackson ObjectMapper is used to convert Java objects to JSON.
- ObjectWriter is used to format the JSON with proper indentation.
- HashSet is converted to a JSON string using ObjectWriter.
- The JSON string is printed to the output.
Output:
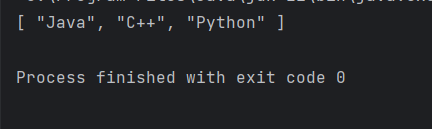
Share your thoughts in the comments
Please Login to comment...