Command-Line Option and Argument Parsing using argparse in Python
Last Updated :
24 Nov, 2023
Command line arguments are those values that are passed during the calling of the program along with the calling statement. Usually, python uses sys.argv
array to deal with such arguments but here we describe how it can be made more resourceful and user-friendly by employing argparse
module.
Python Argparse Module
The ‘argparse'
module in Python helps create a program in a command-line-environment in a way that appears not only easy to code but also improves interaction. The argparse
module also automatically generates help and usage messages and issues errors when users give the program invalid arguments.
Steps for Using Argparse
Creating a Parser: Importing argparse module is the first way of dealing with the concept. After you’ve imported it you have to create a parser or an ArgumentParser object that will store all the necessary information that has to be passed from the python command-line.
Syntax: class argparse.ArgumentParser(prog=None, usage=None, description=None, epilog=None, parents=[], formatter_class=argparse.HelpFormatter, prefix_chars=’-‘, fromfile_prefix_chars=None, argument_default=None, conflict_handler=’error’, add_help=True, allow_abbrev=True) Parameters:
- prog– name of the program (default=sys.argv[0])
- usage– string describes the program usage(default: generated from arguments added to the parser)
- description– text to display before the argument help(default: none)
- epilog– text to display after the argument help (default: none)
- parents– list of ArgumentParser objects whose arguments should also be included
- formatter_class– class for customizing the help output
- prefix_chars– set of characters that prefix optional arguments (default: ‘-‘)
- fromfile_prefix_chars– set of characters that prefix files from which additional arguments should be read (default: None)
- argument_default– global default value for arguments (default: None)
- conflict_handler– strategy for resolving conflicting optionals (usually unnecessary)
- add_help– Add a -h/–help option to the parser (default: True)
- allow_abbrev– Allows long options to be abbreviated if the abbreviation is unambiguous. (default: True)
Adding Arguments: Next step is to fill the ArgumentParser with the information about the arguments of the program. This implies a call to the add_argument()
method. These information tell ArgumentParser how to take arguments from the command-line and turn them into objects.
Syntax: ArgumentParser.add_argument(name or flags…[, action][, nargs][, const][, default][, type][, choices][, required][, help][, metavar][, dest]) Parameters:
- name or flags– either a name or list of option string
- action– basic type of action to be taken when this argument is encountered at the command line
- nargs– number of command-line arguments that should be consumed
- const– constant value required by some action and nargs selections
- default– value produced if the arguments are absent from the command line
- type– type to which the command line arguments should be converted.
- choices – A container of the allowable values for the argument
- required – Whether or not the command-line option may be omitted (optionals only)
- help– brief description of what the argument does
- metavar – A name for the argument in usage messages
- dest – The name of the attribute to be added to the object returned by parse_args()
Parsing Arguments: The information gathered in the step 2 is stored and used when arguments are parsed through parse_args(). The data is initially stored in sys.argv array in a string format. Calling parse_args() with the command-line data first converts them into the required data type and then invokes the appropriate action to produce a result.
Syntax: ArgumentParser.parse_args(args=None, namespace=None) Parameter:
- args – List of strings to parse. The default is taken from sys.argv.
- namespace – An object to take the attributes. The default is a new empty Namespace object
Application of Argparse in Python
In most cases, this means a simple Namespace object will be built up from attributes parsed out of the command line:
Namespace(accumulate=, integers=[ 2, 8, -7, 41 ])
These were the root concepts you need to be familiar with to deal with argparse. The following are some examples to support this application.
Example 1: To find the sum of command-line arguments using argparse
This code employs the ‘argparse'
module to create a command-line interface for processing integers. It defines two command-line arguments: ‘integers’ to accept multiple integer values, and ‘accumulate’ to perform a sum operation on those integers. When the script is executed with integer values as arguments, it parses and calculates the sum of those integers, displaying the result. It provides a convenient way to perform integer accumulation through the command line.
Python3
import argparse
parser = argparse.ArgumentParser(description = 'Process some integers.' )
parser.add_argument( 'integers' , metavar = 'N' ,
type = int , nargs = '+' ,
help = 'an integer for the accumulator' )
parser.add_argument(dest = 'accumulate' ,
action = 'store_const' ,
const = sum ,
help = 'sum the integers' )
args = parser.parse_args()
print (args.accumulate(args.integers))
|
Output:
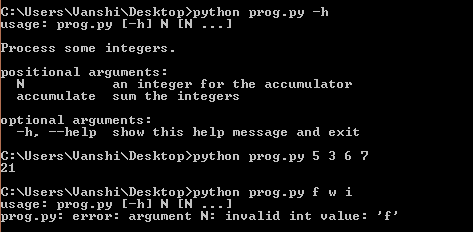
Example 2: Program to arrange the integer inputs in ascending order
This code utilizes the ‘argparse'
module to create a command-line interface for sorting integers in ascending order. It defines two command-line arguments: ‘integers’ to accept multiple integer values and ‘accumulate’ to arrange these integers using the ‘sorted'
function. When the script is executed with integer values as arguments, it parses and displays the sorted integers in ascending order, offering a convenient way to perform integer sorting through the command line.
Python3
import argparse
parser = argparse.ArgumentParser(description = 'sort some integers.' )
parser.add_argument( 'integers' ,
metavar = 'N' ,
type = int ,
nargs = '+' ,
help = 'an integer for the accumulator' )
parser.add_argument(dest = 'accumulate' ,
action = 'store_const' ,
const = sorted ,
help = 'arranges the integers in ascending order' )
args = parser.parse_args()
print (args.accumulate(args.integers))
|
Output:
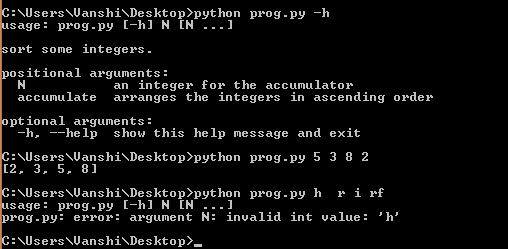
Example 3: To find the average of the entered command line numerical arguments
This code utilizes the ‘argparse'
module to create a command-line interface for calculating the average of floating-point numbers. It defines two command-line arguments: ‘integers’ to accept multiple floating-point values and ‘sum’ and ‘len’ to perform sum and length calculations. The script calculates the average by dividing the sum by the number of provided values and prints the result. It offers a straightforward way to compute the average of floating-point numbers through the command line.
Python3
import argparse
parser = argparse.ArgumentParser(description = 'sort some integers.' )
parser.add_argument( 'integers' ,
metavar = 'N' ,
type = float ,
nargs = '+' ,
help = 'an integer for the accumulator' )
parser.add_argument( 'sum' ,
action = 'store_const' ,
const = sum )
parser.add_argument( 'len' ,
action = 'store_const' ,
const = len )
args = parser.parse_args()
add = args. sum (args.integers)
length = args. len (args.integers)
average = add / length
print (average)
|
Output:
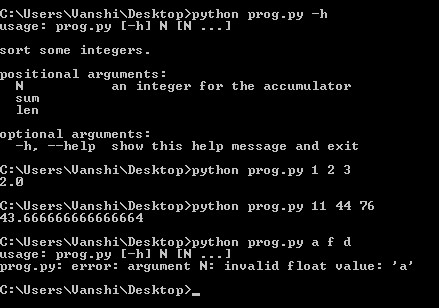
Share your thoughts in the comments
Please Login to comment...