Can you embed JavaScript expressions within JSX?
Last Updated :
04 Feb, 2024
Yes! In web development, combining JavaScript with JSX opens up dynamic possibilities for creating interactive UI components. In this article, you will learn the step-by-step process of integration of JavaScript expressions within JSX.
Prerequisites:
What is JSX and How it works?
JSX, or JavaScript XML, is a syntax extension. it essentially allows us to write HTML-like syntax within JavaScript. For example:
const element = <h1>Hello, world!</h1>;
Here, <h1>Hello, world!</h1> looks like HTML but it’s actually JSX. When this code is transpiled, it gets converted into regular JavaScript function calls that create React elements.
We will use the following approached to embed JavaScript expressions within JSX:
1. Embedding JavaScript Variables
JavaScript variables can be directly embedded within JSX using curly braces {}. For example:
const name = "John";
const element = <h1>Hello, {name}!</h1>;
Here, {name} is replaced with the value of the name variable when rendered.
2: Utilizing JavaScript Functions
JavaScript functions can be used to generate dynamic content within JSX. For example:
function greet(name) {
return <h1>Hello, {name}!</h1>;
}
const element = greet("Geek");
The greet function returns a JSX element containing a personalized greeting.
3: Conditional Rendering
Conditional rendering allows for displaying different components based on conditions. Example:
const isLoggedIn = true;
const element = (
<div>
{isLoggedIn ? <p>Welcome, Geek!</p> : <p>Please log in</p>}
</div>
);
Here, the message changes based on the value of the isLoggedIn variable.
Steps to Create Application (Install Required Modules):
Step 1: Create a React Vite application and Navigate to the project directory the following command:
npm init vite@latest my-jsx-project --template react
cd my-jsx-project
Step 2: Install Packages:
npm install
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
Project Structure:
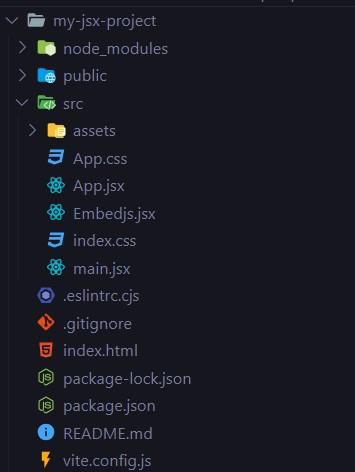
Project Strucutre
Step 3: Create a simple React component Embedjs.jsx in src folder, to see embedding JavaScript expressions within JSX, and change the following existing files:
Javascript
import React from 'react' ;
function Greeting() {
const name = "Geek" ;
function generateGreeting(name) {
return <h2 style={{ color: 'green' }}>
Hello, {name}!</h2>;
}
const isLoggedIn = true ;
return (
<div style={{
fontFamily: 'Arial, sans-serif' ,
textAlign: 'center'
}}>
{ }
<div style={{ marginBottom: '20px' }}>
<h3>Approach 1: Embedding JavaScript variables</h3>
<h2 style={{ color: 'green' }}>
Hello, {name}!</h2>
</div>
{ }
<div style={{ marginBottom: '20px' }}>
<h3>Approach 2: Using JavaScript functions</h3>
{generateGreeting( "Geekina" )}
</div>
{ }
<div>
<h3>Approach 3: Conditional rendering</h3>
{isLoggedIn ? <h2 style={{ color: 'green' }}>
Welcome, User!</h2> : <h2>Please log in </h2>}
</div>
</div>
);
}
export default Greeting;
|
Javascript
import { useState } from 'react'
import reactLogo from './assets/react.svg'
import viteLogo from '/vite.svg'
import './App.css'
import Embedjs from './Embedjs'
function App() {
const [count, setCount] = useState(0)
return (
<>
<Embedjs />
</>
)
}
export default App
|
Step 4: Start Your Application using the following command:
npm run dev
Output:
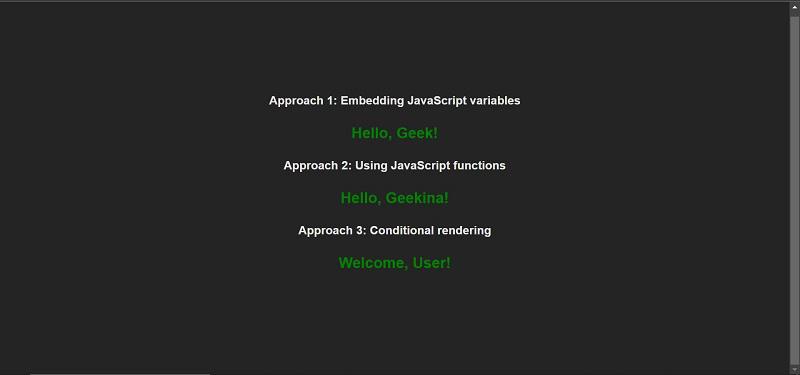
Final Output
Share your thoughts in the comments
Please Login to comment...