C# – Reading Lines From a File Until the End of File is Reached
Last Updated :
27 Dec, 2021
Given a file, now our task is to read lines from the file until the end of the file using C#. Before reading the lines from a file we must have some data, so first we need an empty file in our path and then insert lines in our file and then we read lines from a file. So to do this task we use two basic operations that are reading and writing. The file becomes a stream when we open the file for writing and reading, here the stream means a sequence of bytes that is used for communication. So to our task, we use:
Path: For reading a file from any source we have to need the location/path. A Path is a string that includes a file path in a system.
@"c:\folder\file_name.txt"
We will check if the file exists in the path or not by using File.Exists(path) method
StreamWriter: StreamWriter is used to write a stream of data/lines to a file.
StreamWriter sw = new StreamWriter(myfilepath)
StreamReader: StreamReader is used to read a stream of data/lines from a file.
StreamReader sr = new StreamReader(myfilepath)
Peek: Used to read the data/lines from the file till the end of the file.
StreamReaderObject.Peek()
So all are placed in try() block to catch the exceptions that occur.
Example: Consider the path and file are:
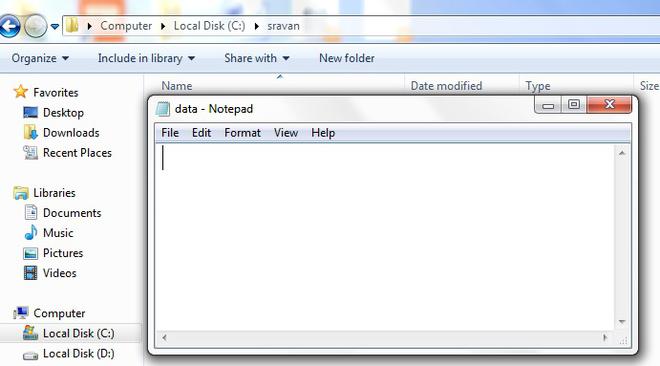
C#
using System;
using System.IO;
class GFG{
public static void Main()
{
string myfilepath = @"c:\sravan\data.txt" ;
try
{
if (File.Exists(path))
{
File.Delete(path);
}
using (StreamWriter sw = new StreamWriter(myfilepath))
{
sw.WriteLine( "hello" );
sw.WriteLine( "geeks for geeks" );
sw.WriteLine( "welcome to c#" );
}
using (StreamReader sr = new StreamReader(myfilepath))
{
while (sr.Peek() >= 0)
{
Console.WriteLine(sr.ReadLine());
}
}
}
catch (Exception e)
{
Console.WriteLine( "The process failed: {0}" , e.ToString());
}
Console.Read();
}
|
Output:
hello
geeks for geeks
welcome to c#
See the file data is inserted:
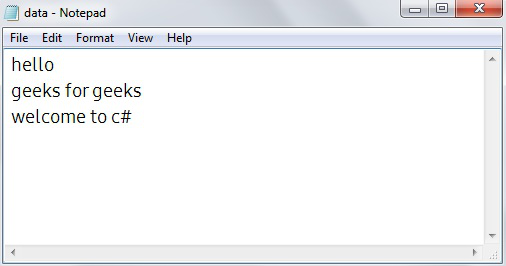
Share your thoughts in the comments
Please Login to comment...