C# Program For Reading Data From Stream and Cast Data to Chars
Last Updated :
01 Nov, 2021
Given data, now our task is to read data from the stream and cast data to chars in C#. So to do this task we use the following class and methods:
- FileStream: It is a class that is used to read and write files. So, to manipulate files using FileStream, you need to create an object of FileStream class.
Syntax:
Stream object = new FileStream(path, FileMode.Open)
Where path is the location of your file – @”c:\A\data.txt” and FileMode is the mode of the file like reading and writing.
- ReadByte(): This method is used to read the data from the file Byte by Byte. This method will throw NotSupportException when the current stream did not support reading. If the current stream is closed then this method will throw ObjectDisposedException.
Syntax:
FileStream_object.ReadByte()
Example:
Let us consider a file named “file.txt” is present in the A folder of C drive like as shown in the below image:
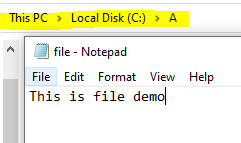
Now we read data from stream and cast data to chars. So to this follow the following approach.
Approach
- Read the file named “file.txt” using FileStream with the help of specified path.
- Read the data in file byte by byte using ReadByte() method until it reaches end of file.
while ((obj = s.ReadByte()) != -1)
{
// Convert the data into chars and display
Console.Write("{0} ", (char)obj);
}
- Display file data into chars using Casting to char.
(char)obj
C#
using System;
using System.IO;
public sealed class GFG{
public static void Main()
{
using (Stream s = new FileStream( @"c:\A\file.txt" , FileMode.Open))
{
int obj;
while ((obj = s.ReadByte()) != -1)
{
Console.Write( "{0} " , ( char )obj);
}
Console.ReadLine();
}
}
}
|
Output:
T H I S I S F I L E D E M O
Share your thoughts in the comments
Please Login to comment...