C Program for Quadratic Equation Roots
Last Updated :
14 Jul, 2023
In this article, we will learn to write a C program to find the roots of the quadratic equation.
Quadratic Equation is polynomial equations that have a degree of two, which implies that the highest power of the function is two. The quadratic equation is represented by ax2 + bx + c where a, b, and c are real numbers and constants, and a ≠0. The root of the quadratic equations is a value of x that satisfies the equation.
How to Find Quadratic Equation Roots?
The Discriminant is the quantity that is used to determine the nature of roots:
Discriminant(D) = b2 - 4ac;
Based on the nature of the roots, we can use the given formula to find the roots of the quadratic equation.
1. If D > 0, Roots are real and different
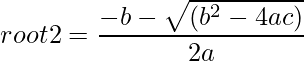
2. If D = 0, Roots are real and the same
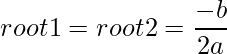
3.If D < 0, Roots are complex

Program to Find Roots of a Quadratic Equation
C
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
void findRoots( int a, int b, int c)
{
if (a == 0) {
printf ( "Invalid" );
return ;
}
int d = b * b - 4 * a * c;
double sqrt_val = sqrt ( abs (d));
if (d > 0) {
printf ( "Roots are real and different\n" );
printf ( "%f\n%f" , ( double )(-b + sqrt_val) / (2 * a),
( double )(-b - sqrt_val) / (2 * a));
}
else if (d == 0) {
printf ( "Roots are real and same\n" );
printf ( "%f" , -( double )b / (2 * a));
}
else
{
printf ( "Roots are complex\n" );
printf ( "%f + i%f\n%f - i%f" , -( double )b / (2 * a),
sqrt_val / (2 * a), -( double )b / (2 * a),
sqrt_val / (2 * a));
}
}
int main()
{
int a = 1, b = -7, c = 12;
findRoots(a, b, c);
return 0;
}
|
Output
Roots are real and different
4.000000
3.000000
Complexity Analysis
Time Complexity: O(log(D)), where D is the discriminant of the given quadratic equation.
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...