Building RESTful APIs with FastAPI
Last Updated :
04 Nov, 2023
FastAPI is a Python web framework that makes it easy to build APIs quickly and efficiently. There is Another famous framework for doing the same is Flask, which is also Python-based. In this article, we will focus on creating a RESTful API using FastAPI. In this article, we will learn how to create a basic ToDo List API using FastAPI. FastAPI is a Python web framework known for its simplicity and speed, making it a great choice for beginners.
Setting up the Project
Before we start, make sure you have Python installed on your computer. You’ll also need to install FastAPI and Uvicorn, For creating API using FastAPI we need fastAPI, also for running the server we also need a uvicorn.
We can install them by running the below command
pip install fastapi
pip install uvicorn
Setting Up FastAPI Application
- Creating a Directory/Folder: We start by creating a new directory/folder for our project. we can name it something like “todoAPI”.
- Creating a Python File: Inside your project directory/folder, create a Python file named main.py. This will be the main entry point for our FastAPI application.
So our project structure is now set for writing the actual code to create API using fastAPI.
Creating FastAPI App
Here we have created the FastAPI app.
Python3
from fastapi import FastAPI
app = FastAPI()
|
Defining ToDo Model
Let’s define a simple ToDo model that represents each task. here we simple define Task model which has 2 field title and discription both are of type string.
Python3
from pydantic import BaseModel
class Task(BaseModel):
title: str
description: str = ""
|
Creating Endpoints for ToDo List
In this step we will create diff – diff endpoints for managing our ToDo list. Here we have to make sure we follow the syntax for creating endpoint for diff diff request type such as GET and POST request.
Here we use simple python list tasks as our data structure to store data.
Endpoint 1: Add a Task in ToDo List
Here in this endpoint whenever end user do POST request on ‘/tasks/‘ we will add that task in tasks list and simply return the msg “msg”: “task added succesfully” as response
Python3
@app .post( "/tasks/" )
def create_task(task: Task):
tasks.append(task)
return { "msg" : "task added succesfully" }
|
Endpoint 2: Get All Tasks
Here we add an endpoint to retrieve all taske by sending GET request to ‘/tasks/‘
Python3
@app .get( "/tasks/" , response_model = List [Task])
def get_tasks():
return tasks
|
Endpoint 3: Get a Specific Task
We create an endpoint to retrieve a specific task by it’s index in the list, here we user send GET request to ‘/tasks/indexOfTask‘ we send that task details in response to the user.
Python3
@app .get( "/tasks/{task_id}" , response_model = Task)
def get_task(task_id: int ):
if 0 < = task_id < len (tasks):
return tasks[task_id]
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
|
Here we had also added HTTPException because when user send some specific task ID and that task is not in our dataBase we send an error message that “Task not found“.
Endpoint 4: Update a Task
In this endpint we had Implement an endponint to update a task specific task with it’s task_id. here not that user is sending a PUT request to endpoint “/tasks/{task_id}“
Python3
@app .put( "/tasks/{task_id}" , response_model = Task)
def update_task(task_id: int , updated_task: Task):
if 0 < = task_id < len (tasks):
tasks[task_id] = updated_task
return updated_task
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
|
Endpoint 5: Delete a specific Task
In this endpoint we will implement the deletion functionality, by task_id user can delete a specific task from the Tasks list.
Python3
@app .delete( "/tasks/{task_id}" , response_model = Task)
def delete_task(task_id: int ):
if 0 < = task_id < len (tasks):
deleted_task = tasks.pop(task_id)
return deleted_task
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
|
Complete Code
The provided code is a FastAPI application for managing tasks with CRUD operations:
- Create a new task with a title and description.
- Retrieve a list of all tasks.
- Get a single task by its ID.
- Update an existing task by its ID.
- Delete a task by its ID.
The application uses an in-memory list as a database to store tasks. It runs on http://127.0.0.1:8000. You can interact with it using API client tools or by sending HTTP requests to the defined endpoints.
Python3
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List
import uvicorn
app = FastAPI()
class Task(BaseModel):
title: str
description: str = ""
tasks = []
@app .post( "/tasks/" )
def create_task(task: Task):
tasks.append(task)
return { "msg" : "task added succesfully" }
@app .get( "/tasks/" , response_model = List [Task])
def get_tasks():
return tasks
@app .get( "/tasks/{task_id}" , response_model = Task)
def get_task(task_id: int ):
if 0 < = task_id < len (tasks):
return tasks[task_id]
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
@app .put( "/tasks/{task_id}" , response_model = Task)
def update_task(task_id: int , updated_task: Task):
if 0 < = task_id < len (tasks):
tasks[task_id] = updated_task
return updated_task
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
@app .delete( "/tasks/{task_id}" , response_model = Task)
def delete_task(task_id: int ):
if 0 < = task_id < len (tasks):
deleted_task = tasks.pop(task_id)
return deleted_task
else :
raise HTTPException(status_code = 404 , detail = "Task not found" )
if __name__ = = "__main__" :
uvicorn.run(app, host = "127.0.0.1" , port = 8000 )
|
Deployement of the Project
Most of the developer use postman as tool to test an API we will also use the same. Here are some sample API requests we can make to test our created API endpoints:
Creating a Task
POST http://localhost:8000/tasks/
Body: {"title": "title of task", "description": "description of task"}
Get All Tasks:
GET http://localhost:8000/tasks/
Get a Specific Task (replace {task_id} with the task index):
GET http://localhost:8000/tasks/{task_id}
Update a Task (replace {task_id} with the task index):
PUT http://localhost:8000/tasks/{task_id}
Body: {"title": "updated title"}
Delete a Task (replace {task_id} with the task index):
DELETE http://localhost:8000/tasks/{task_id}
Output:
Adding the tasks 0:
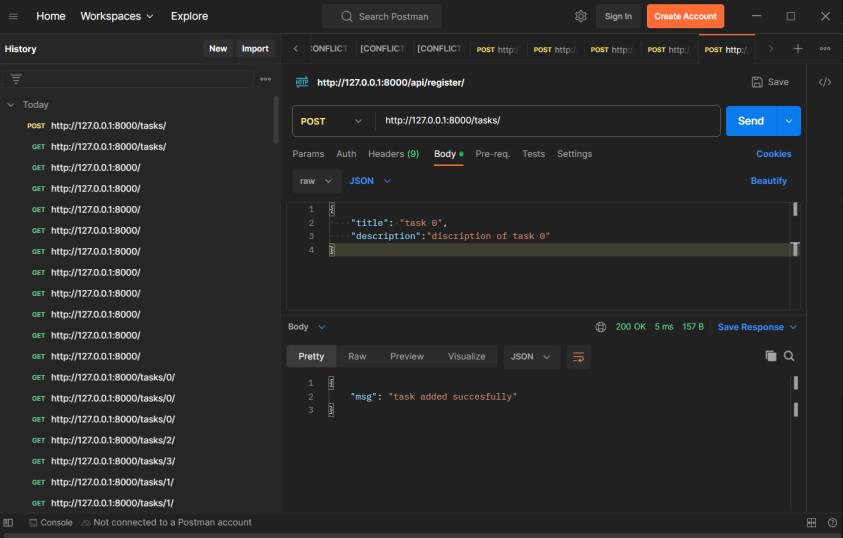
adding task 0 with POST request
Adding the tasks 1:
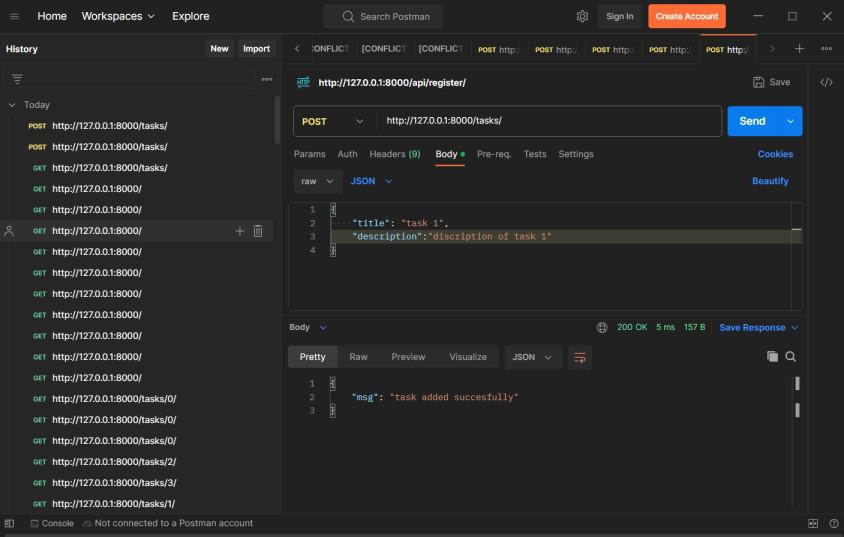
adding task 1 using POST request
Adding the tasks 2:
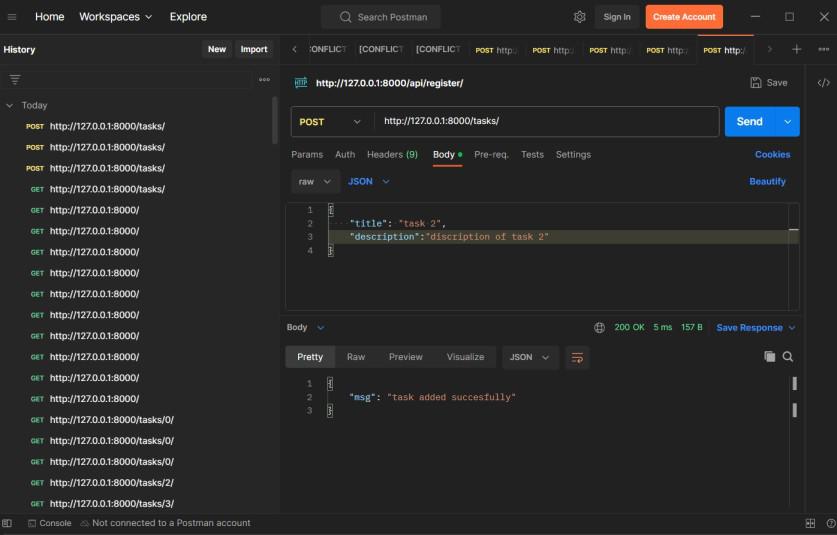
adding task 2 with POST request
Accessing all the tasks
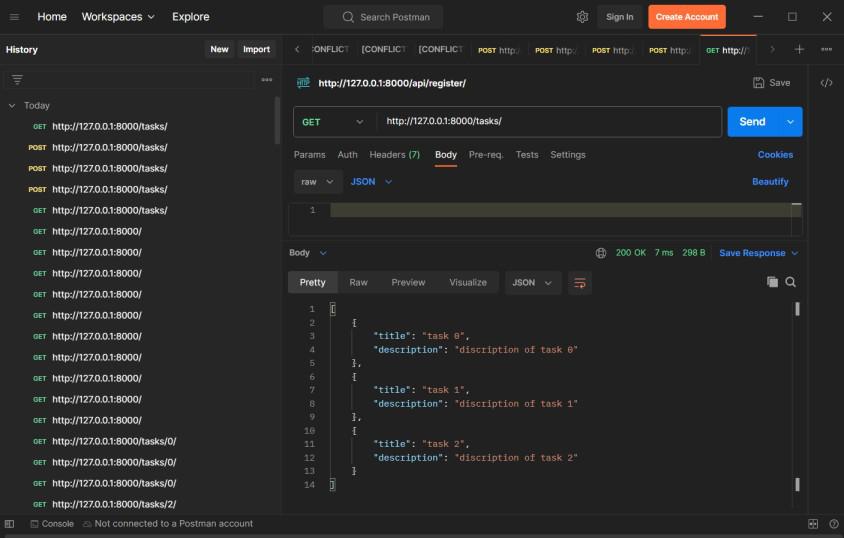
accessing all tasks by GET request
Accessing specific tasks with id
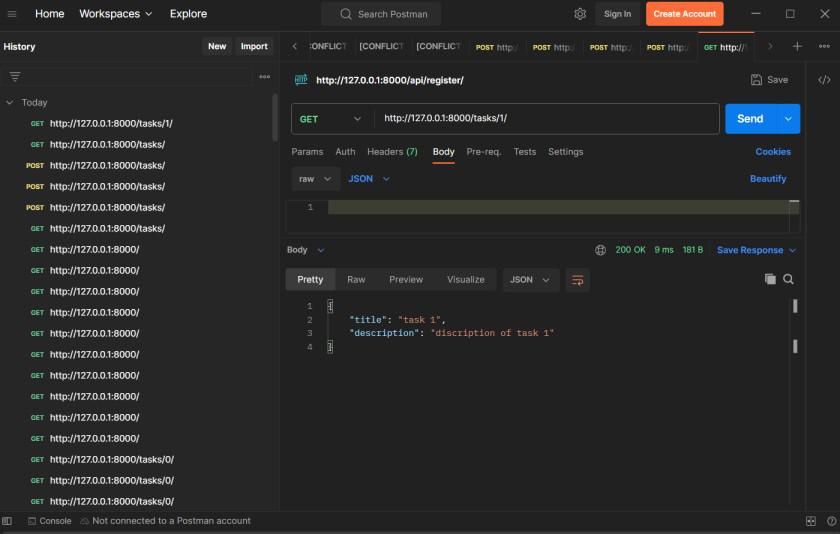
accessing specific task with task_id=1 and GET request
Updating the tasks
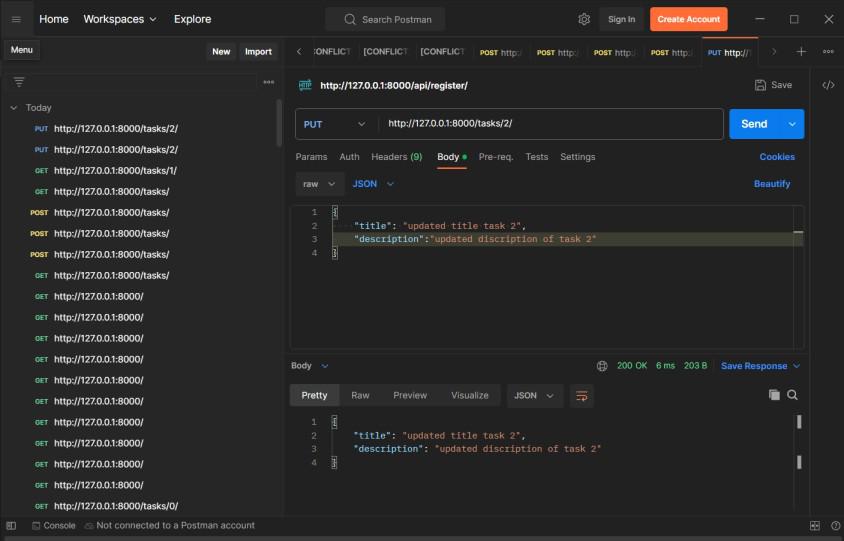
updating task where task_id=2 using PUT request
Accessing the updated tasks
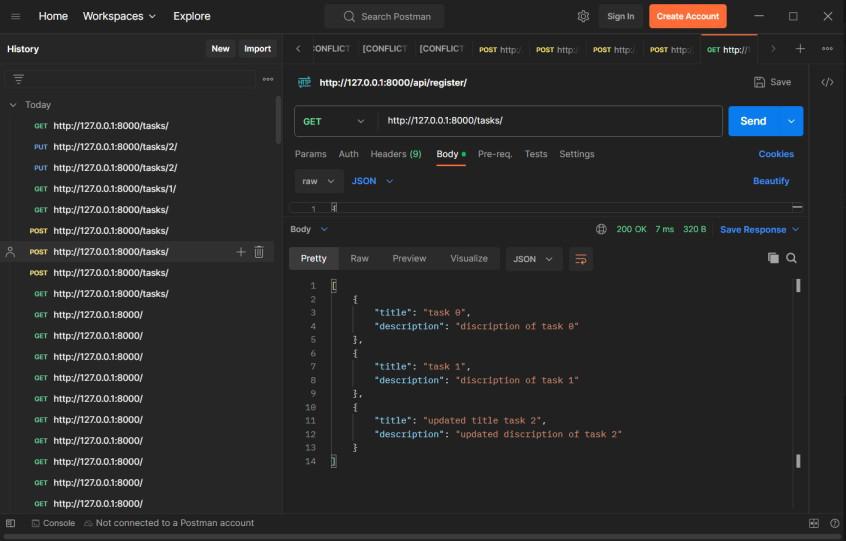
accessing all updated task after updating task where task_id=2 using GET request
Deleting the tasks
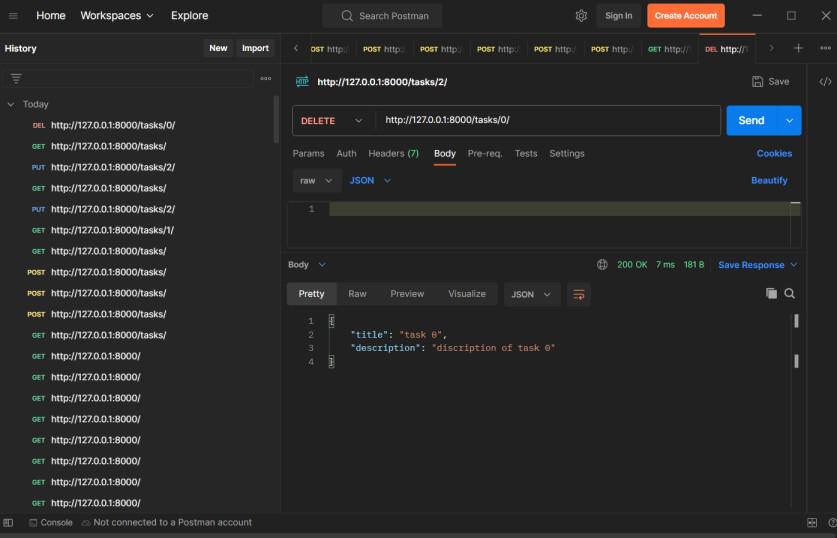
deleting task where task_id=0 using DELETE request
Accesing the task after deleting
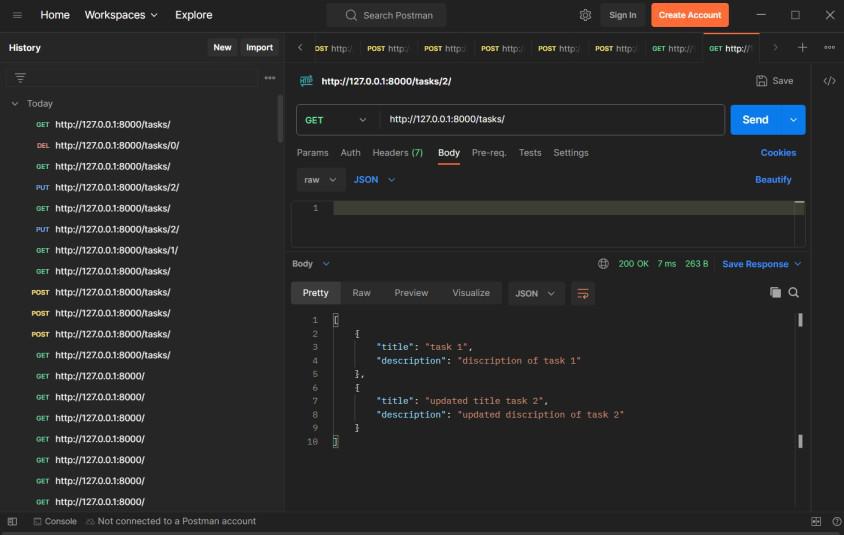
Accessing all task after deleting task where task_id=0 using GET request
Share your thoughts in the comments
Please Login to comment...