BigIntegerMath Class | Guava | Java
Last Updated :
28 Aug, 2018
BigIntegerMath is used to perform mathematical operations on BigInteger values. Basic standalone math functions are divided into the classes IntMath, LongMath, DoubleMath, and BigIntegerMath based on the primary numeric type involved. These classes have parallel structure, but each supports only the relevant subset of functions. Similar functionality for int and for long can be found in IntMath and LongMath respectively.
Declaration : The declaration for com.google.common.math.BigIntegerMath class is :
@GwtCompatible(emulated = true)
public final class BigIntegerMath
extends Object
Below table shows the methods provided by BigIntegerMath Class of Guava :
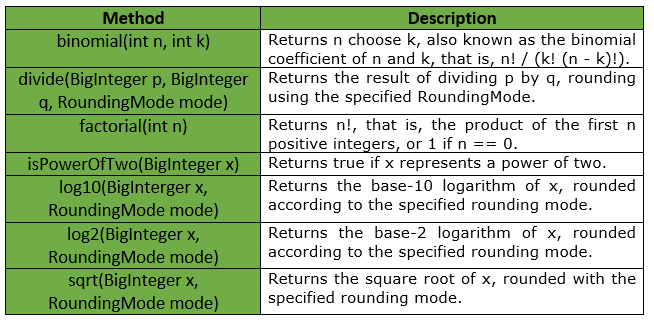
Exceptions :
- log2 : IllegalArgumentException if x <= 0
- log10 : IllegalArgumentException if x <= 0
- sqrt : IllegalArgumentException if x < 0
- divide : ArithmeticException if q == 0, or if mode == UNNECESSARY and a is not an integer multiple of b
- factorial : IllegalArgumentException if n < 0
- binomial : IllegalArgumentException if n < 0, k n
Example 1 :
import java.math.*;
import com.google.common.math.BigIntegerMath;
class GFG {
public static void main(String args[])
{
GFG obj = new GFG();
obj.examples();
}
private void examples()
{
try {
System.out.println(BigIntegerMath.divide(BigInteger.TEN,
new BigInteger( "3" ),
RoundingMode.UNNECESSARY));
}
catch (ArithmeticException ex) {
System.out.println( "Error Message is : " +
ex.getMessage());
}
}
}
|
Output:
Error Message is : Rounding necessary
Example 2 :
import java.math.*;
import com.google.common.math.BigIntegerMath;
class GFG {
public static void main(String args[])
{
GFG obj = new GFG();
obj.examples();
}
private void examples()
{
System.out.println(BigIntegerMath.divide(BigInteger.TEN,
new BigInteger( "5" ),
RoundingMode.UNNECESSARY));
System.out.println( "Log10 is : " +
BigIntegerMath.log10( new BigInteger( "1000" ),
RoundingMode.HALF_EVEN));
System.out.println( "factorial is : " +
BigIntegerMath.factorial( 7 ));
System.out.println( "Log2 is : " +
BigIntegerMath.log2( new BigInteger( "8" ),
RoundingMode.HALF_EVEN));
System.out.println( "sqrt is : " +
BigIntegerMath.sqrt(BigInteger.
TEN.multiply(BigInteger.TEN),
RoundingMode.HALF_EVEN));
}
}
|
Output:
2
Log10 is : 3
factorial is : 5040
Log2 is : 3
sqrt is : 10
Reference : Google Guava
Share your thoughts in the comments
Please Login to comment...