Backbone.js Underscore Methods (9) Model
Last Updated :
13 Jul, 2022
Backbone.js Underscore Methods Model is the 9 Underscore models which we can use with Backbone models. These are the proxies to underscore.js to provide 9 object functions on Backbone.Model. These functions are keys, values, pairs, inverts, pick, omit, chain and isEmpty.
Syntax:
Backbone.Model.method_Name( );
Parameters: Different function accepts different parameters.
Example 1: In this example, we will see keys, values, and pairs functions. These function does not require any parameters.
HTML
<!DOCTYPE html>
< html >
< head >
< title >BackboneJS Model underscore methods</ title >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >BackboneJS Model Underscore methods</ h3 >
< script type = "text/javascript" >
var Geek = Backbone.Model.extend();
var Geek1 = new Geek({
id: "1001e",
Name: "cody",
});
var Geek2 = new Geek({
id: "1002e",
Name: "Geeky",
});
var Geek3 = new Geek({
id: "1003e",
Name: "zetshu",
});
// Keys() function
document.write(`All the keys of ${Geek1.get('Name')} : `);
document.write(JSON.stringify(Geek1.keys()), '< br >< br >');
// values() function
document.write(`All the values of ${Geek2.get('Name')} : `);
document.write(JSON.stringify(Geek2.values()), '< br >< br >');
// pairs() function
document.write(`All the keys and values of ${Geek3.get('Name')} : `);
document.write(JSON.stringify(Geek3.pairs()), '< br >< br >');
</ script >
</ body >
</ html >
|
Output:
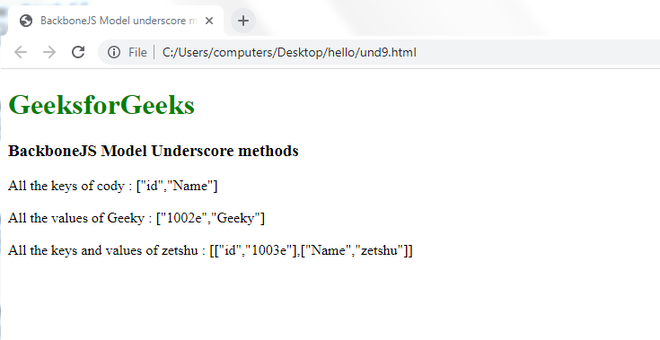
Backbone.js Underscore 9 method Model
Example 2: In this example, we will see the invert, chain, and isEmpty function. three of these function does not take any parameters.
HTML
<!DOCTYPE html>
< html >
< head >
< title >BackboneJS Model underscore methods</ title >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >BackboneJS Model Underscore methods</ h3 >
< script type = "text/javascript" >
var Geek = Backbone.Model.extend();
var Geek4 = new Geek({
id: "1004e",
Name: "itachi",
});
var Geek5 = new Geek({
id: "1005e",
Name: "tobi",
});
var Geek6 = new Geek({
id: "1006e",
Name: "lufy",
});
// invert() function
document.write(`Invert All the keys and values of ${Geek4.get('Name')} : `);
document.write(JSON.stringify(Geek4.invert()), '< br >< br >')
// chain() function
document.write(`Chain keys and values of ${Geek5.get('Name')} : `);
document.write(Geek5.chain().map(function (l, g)
{ return g + " is " + l + " "; }), '< br >< br >');
// isEmpty() function
document.write(`Checking ${Geek6.get('Name')} is empty : `);
document.write(Geek6.isEmpty())
</ script >
</ body >
</ html >
|
Output:
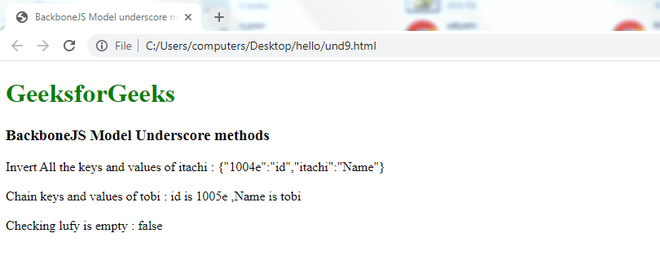
invert() , chain() , and isEmpty()
Example 3: In this example, we will see omit and pick method. Two of these function takes the attribute’s name as a parameter on which you want to perform the function.
HTML
<!DOCTYPE html>
< html >
< head >
< title >BackboneJS Model underscore methods</ title >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
< script src =
type = "text/javascript" >
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >BackboneJS Model Underscore methods</ h3 >
< script type = "text/javascript" >
var Geek = Backbone.Model.extend();
var Geek1 = new Geek({
id: "1001e",
Name: "cody",
});
var Geek2 = new Geek({
id: "1002e",
Name: "Geeky",
});
// pick() function
document.write(`Pick id attribute of ${Geek1.get('Name')} : `);
document.write(JSON.stringify(Geek1.pick('id')), '< br >< br >');
// omit() function
document.write(`Omit Name attribute of ${Geek2.get('Name')} : `);
document.write(JSON.stringify(Geek2.omit('Name')), '< br >< br >');
</ script >
</ body >
</ html >
|
Output:
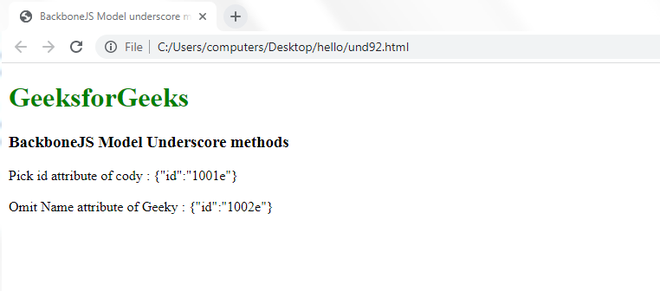
pick() and omit()
Reference: https://backbonejs.org/#Model-Underscore-Methods
Share your thoughts in the comments
Please Login to comment...