Backbone.js preinitialize View
Last Updated :
15 Mar, 2023
Backbone.js preinitialize function is a special function that can be defined in a Backbone View. It is called when the View is created, but before any initialization logic is performed. This function is typically used to perform any setup or initialization that needs to be done before the View is fully initialized.
Syntax:
var View = Backbone.View.extend({
preinitialize: function() {
// preinitialization code here
}
});
Parameters: The preinitialize function does not take any parameters.
Example 1: In this example, we define a new Backbone View called MyView and add a preinitialize function that sets up some default options for the view. The preinitialize function takes an options argument that can be used to override the default options. We then define a render function that outputs some text to the document with styles based on the options passed to the view. Finally, we create two instances of the MyView object, one with custom options and one with the default options, and call their render functions to display the text.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Backbone.js preinitialize View Example
</ title >
< script src =
</ script >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >Backbone.js preinitialize View</ h3 >
< script type = "text/javascript" >
// Define a new Backbone View
// with a preinitialize function
var MyView = Backbone.View.extend({
preinitialize: function (options) {
this.options = _.extend({
color: 'blue',
fontSize: '16px'
}, options);
},
render: function () {
document.write
('< p style="color:'
+ this.options.color
+ ';font-size:'
+ this.options.fontSize
+ ';">This text is styled'
+ ' using the options passed'
+ ' to the view.</ p >');
}
});
// Create a new instance of the MyView
// object with custom options
var myView1 = new MyView({
color: 'green',
fontSize: '20px'
});
// Create a new instance of the MyView
// object with default options
var myView2 = new MyView();
// Call the render function of the
// MyView objects
myView1.render();
myView2.render();
</ script >
</ body >
</ html >
|
Output:
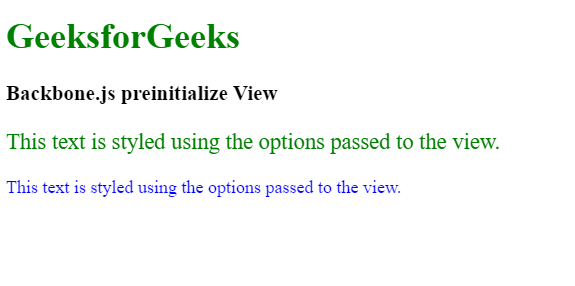
Backbone.js preinitialize View
Example 2: In this example, we define a new Backbone View called MyView and add a preinitialize function that sets up an event listener for a button click. We use the _.extend function to merge the default this.events object with the custom events object, which specifies that we want to listen for a click on the #my-button element and call the handleButtonClick function when it is clicked. The handleButtonClick function simply displays an alert message to the user. Finally, we create an instance of the MyView object and pass it the el option to specify that it should be attached to the body element of the document. When the button is clicked, the handleButtonClick function is called and the alert message is displayed.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Backbone.js preinitialize View
</ title >
< script src =
</ script >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >Backbone.js preinitialize View</ h3 >
< button id = "my-button" >Click me!</ button >
< script type = "text/javascript" >
// Define a new Backbone View with
// a preinitialize function
var MyView = Backbone.View.extend({
preinitialize: function () {
this.events = _.extend({
'click #my-button': 'handleButtonClick'
}, this.events);
},
initialize: function () {
// Initialization code here
this.render();
},
handleButtonClick: function () {
alert('Button clicked!');
}
});
// Create a new instance of the MyView object
var myView = new MyView({
el: 'body'
});
</ script >
</ body >
</ html >
|
Output:
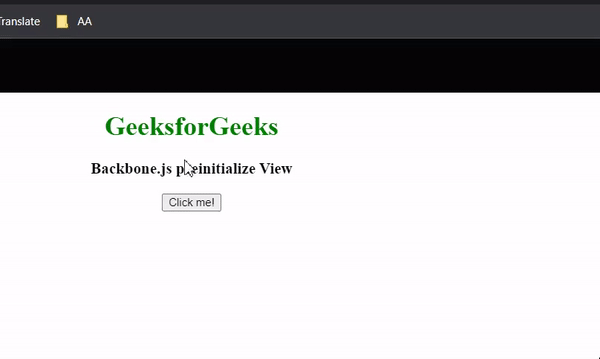
Backbone.js preinitialize View
Reference: https://backbonejs.org/#View-preinitialize
Share your thoughts in the comments
Please Login to comment...