Arithmetic Operators in Solidity
Last Updated :
21 Mar, 2023
Arithmetic operators are used to perform arithmetic or mathematical operations. Solidity has the following types of arithmetic operators:
- Addition: The addition operator takes two operands and results in a sum of these operands. It is denoted by +.
- Subtraction: The subtraction operator takes two operands and results in a difference between these operands. It is denoted by -.
- Multiplication: The multiplication operator takes two operands and results in a product of these operands. It is denoted by *.
- Division: The division operator takes two operands and results in a quotient after the division of these operands. It is denoted by /.
- Modulus: The modulus operator takes two operands and results in the remainder after the division of these operands. It is denoted by %.
- Increment: The increment operator takes one operand and increments the operand by one. It is denoted by ++.
- Decrement: The decrement operator takes one operand and decrements the operand by one. It is denoted –.
Operator |
Denotation |
Description |
Addition |
+
|
It results in the sum of two operands. |
Subtraction |
–
|
It results in the difference between the two operands. |
Multiplication |
*
|
It results in the product of two operands. |
Division |
/
|
It results in the quotient of the division of two operands. |
Modulus |
%
|
It results in the remainder of the division of two operands. |
Increment |
++
|
It increments the operand by one. |
Decrement |
—
|
It decrements the operand by one. |
Below is the Solidity program to implement Arithmetic operators:
Solidity
pragma solidity ^0.5.0;
contract Arithmetic {
function arithop(uint a, uint b) public pure returns (uint, uint,
uint, uint,
uint, uint,
uint) {
uint sum = a + b;
uint sub = a - b;
uint mul = a * b;
uint div = a / b;
uint mod = a % b;
uint inc = ++a;
uint dec = --b;
return (sum, sub, mul, div , mod, inc, dec);
}
}
|
Output:
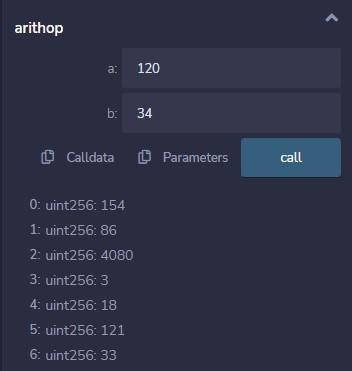
Â
Share your thoughts in the comments
Please Login to comment...