Add a Search Bar to a ColumnView with Python
Last Updated :
19 Apr, 2024
Adding a search bar to a ColumnView in Python can enhance the user experience by allowing the users to easily search and filter the items displayed in the view. In this article, we will see how we can add a search bar to a column view with Python.
Add a Search Bar to a ColumnView with Python
Below are some of the approaches by which we can add a search bar to a ColumnView with Python:
- Using PyQt5
- Using Tkinter
Add a Search Bar to a ColumnView Using PyQt5
In this example, the Python code below uses PyQt5 to create an application with a search bar and a column view. The GFG
class sets up the window layout, including connections for filtering items based on the search bar input. The code initializes the app, creates the window, and runs the event loop.
Python3
import sys
from PyQt5.QtWidgets import QApplication, QColumnView, QFileSystemModel, QVBoxLayout, QLineEdit, QWidget
class GFG(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('Search ColumnView')
layout = QVBoxLayout(self)
# Create search bar
self.search_bar = QLineEdit(self)
self.search_bar.textChanged.connect(self.filter_items)
layout.addWidget(self.search_bar)
# Create ColumnView
self.column_view = QColumnView(self)
model = QFileSystemModel()
model.setRootPath('')
self.column_view.setModel(model)
layout.addWidget(self.column_view)
self.setLayout(layout)
def filter_items(self):
search_query = self.search_bar.text()
# Filter items based on search query
if __name__ == '__main__':
app = QApplication(sys.argv)
window = GFG()
window.show()
sys.exit(app.exec_())
Output:
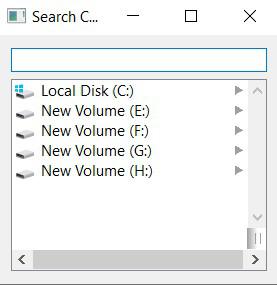
Add a Search Bar to a ColumnView Using Tkinter
In this example, we are creating a tkinter application with a search bar and a column view. The GFG
class sets up the window layout, populating the column view with dummy data. It provides a method filter_items
for filtering items based on the search query, but the filtering logic is not yet implemented.
Python3
import tkinter as tk
from tkinter import ttk
class GFG(tk.Tk):
def __init__(self):
super().__init__()
self.title('Search ColumnView')
# Create search bar
self.search_var = tk.StringVar()
self.search_var.trace('w', self.filter_items)
self.search_entry = ttk.Entry(self, textvariable=self.search_var)
self.search_entry.pack()
# Create ColumnView
self.column_view = ttk.Treeview(self)
# Populate ColumnView with items
# Replace this with your own data population logic
for i in range(10):
self.column_view.insert('', 'end', text=f'Item {i}')
self.column_view.pack()
def filter_items(self, *args):
search_query = self.search_var.get()
if __name__ == '__main__':
app = GFG()
app.mainloop()
Output:
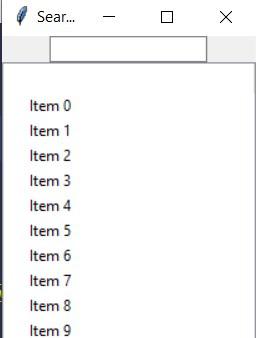
Share your thoughts in the comments
Please Login to comment...