8086 program to convert an 8 bit BCD number into hexadecimal number
Last Updated :
22 May, 2018
Problem – Write an assembly language program in 8086 microprocessor to convert an 8 bit BCD number into hexadecimal number.
Example –
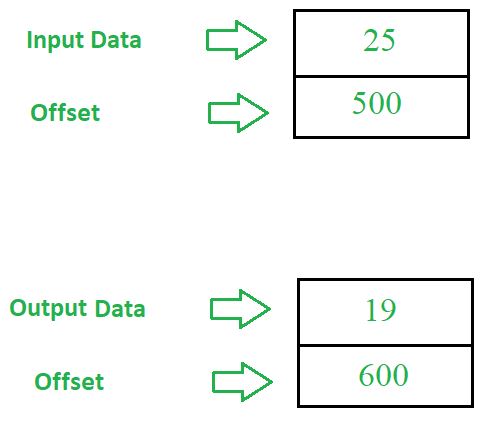
Assumption –
Initial value of segment register is 00000.
Calculation of physical memory address:
Memory Address = Segment Register * 10(H) + Offset
where Segment Register is 00000 (Assumption) and Offset is given in the program.
Algorithm –
- Assign value 500 in SI and 600 in DI.
- Move the contents of [SI] in BL.
- Use AND instruction to calculate AND between 0F and contents of BL.
- Move the contents of [SI] in AL.
- Use AND instruction to calculate AND between F0 and contents of AL.
- Move 04 in CL.
- Use ROR instruction on AL.
- Move 0A in DL.
- Use MUL instruction to multiply AL with DL.
- Use ADD instruction to add AL with BL.
- Move the contents of AL in [DI].
- Halt the program.
Program –
MEMORY ADDRESS |
MNEMONICS |
COMMENT |
0400 |
MOV SI, 500 |
SI <- 500 |
0403 |
MOV DI, 600 |
DI <- 600 |
0406 |
MOV BL, [SI] |
BL <- [SI] |
0408 |
AND BL, 0F |
BL = BL AND 0F |
040A |
MOV AL, [SI] |
AL <- [SI] |
040C |
AND AL, F0 |
BL = AL AND F0 |
040E |
MOV CL, 04 |
CL = 04 |
0410 |
ROR AL, CL |
Rotate AL |
0412 |
MOV DL, 0A |
DL = 0A |
0414 |
MUL DL |
AX = AL * DL |
0416 |
ADD AL, BL |
AL = AL + BL |
0418 |
MOV [DI], AL |
[DI] <- AL |
041A |
HLT |
End of Program |
Explanation – Registers used SI, DI, AL, BL, CL, DL.
- MOV SI,500 is used to move offset 500 to Starting Index(SI)
- MOV DI,600 is used to move offset 600 to Destination Index(DI)
- MOV BL,[SI] is used to move the contents of [SI] to BL
- AND BL,0F is used to mask the higher order nibble from BL
- MOV AL,[SI] is used to move the contents of [SI] to AL
- AND AL,F0 is used to mask the lower order nibble from BL
- MOV CL,04 is used to move 04 to CL
- ROR AL,CL is used to reverse the contents of AL
- MOV DL,0A is used to move 0A to DL
- MUL DL is used to multiply contents of AL with DL
- ADD AL,BL is used to add contents of AL and BL
- MOV [DI],AL is used to move the contents of AL to [DI]
- HLT stops executing the program and halts any further execution
Share your thoughts in the comments
Please Login to comment...