As software systems naturally become more complex, the requirement for new programming languages that are able not only to get good performance but also to be safe and to be on a modern language level increases continuously.
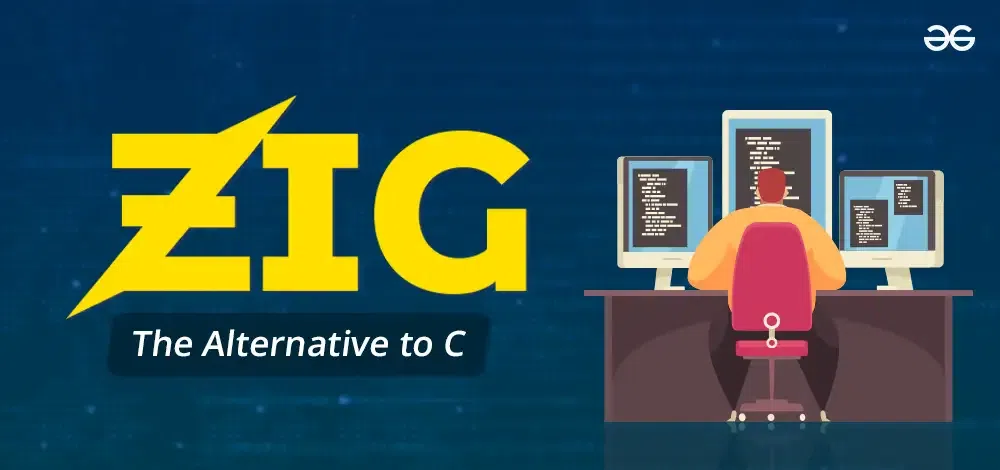
This landscape is where Zig resumes its place among venerable C programming language alternatives, meeting the criteria for fixing weaknesses and preserving strengths. The Zig platform was created by Andrew Kelley back in 2015. During this relatively short period, it has gained the impression and interest of all developers’ communities as an alternative to C.
What is Zig?
Zig is a multipurpose programming language tailored to ensure optimizing, robustness, and user-friendliness factors for different programming areas such as systems, embedded, and application-level software. Developed as an alternative to C, it ensures the latest and modern language features, safety mechanisms, and productivity tools comparable to C and C++ while keeping their efficiency alive. This is where the Zig language philosophy of simplicity, orthogonal design, and zero-cost abstractions come into focus to address the gap between low-level and high-level programming by enabling developers to write agile, effective, and maintainable code to serve a variety of application needs.
The Origin and Goals of Zig
Zig was developed in a bid to design a high-performance systems programming language that borrows the most valuable features from C and C++ while making improvements on their known areas of weakness and introducing novel ideas. Its primary goals include:
- Give clear, spelled-out, and acceptable code that can be deeply comprehended, learned, and integrated even more than C++.
- It has the potential to make the programming correct by mistake through explicit safety measures that identify program crashes, such as memory vulnerabilities and undefined behavior.
- Generating compact machine instructions (bytecodes) that are as fast as or faster than the original C or C++ language.
- Preserving the libraries’ conception with the existing C compiler allows developers to put together upcoming and coder language code that existed before.
- As for compatibility, no matter what platforms and architectures are used, the development can cope with them, which is also the reason for the creation of cross-platforms.
The official Zig documentation states that the language is designed to be “an ideal systems programming language for applications that push current hardware to its limits.”
Safety and Robustness: Analyze C’s Vulnerabilities
One of the most significant advantages of Zig over C is the consideration of reliability and stability. Unlike C, which is prone to memory vulnerabilities and undefined behavior, Zig incorporates modern language features that enhance safety and reliability:
- Automatic Memory Management: Zig includes ownership management in the model, which allows memory management not to be carried out manually as a source of bugs in C programs.
const allocator = std.heap.page_allocator;
const bytes = try allocator.alloc(u8, 1024);
defer allocator.free(bytes);
This example showcases Zig’s memory management mechanism, which reduces memory handling to simple and less likely sources of memory leaks and invalid pointer dereference.
- Bounds Checking: Arrays in Zig are bounds checked for the default, ensuring they do not go out of bounds and fixing the out-of-bounds access, which is a security weakness in C.
const arr = [4]u8{ 1, 2, 3, 4};
const invalid_access = arr[4]; // Compiler error: IndexOutOfBounds
On the other hand, Zig keeps those mistakes at compile time, helping users make their coding as secure as possible by avoiding common security vulnerabilities.
- Null Safety: Zig obstructs the possibility of null pointer dereferences using null in an explicit sense; this is a typical problem that can lead to crashing apps written in C.
const maybe_null: ?*MyStruct = undefined;
switch (maybe_null) {
ptr: {
// The value is guaranteed to be non-null here
const value = ptr.*); // Dereference the pointer
// ... do something with the value
},
null => {
// Handle the null case
},
}
This example demonstrates that Zig demonstrates null safety, which requires explicitly handling null values and prevents unwanted dereferences of a null pointer.
Thanks to these safety features of Zig, which caught standard C and C++ programming deficiencies, the Zig language can safeguard the whole host of bugs seen in C and C++ without compromising performance.
Performance and low-level control
In contrast to the main characteristics of the language, which are safety and production at large, the language of Zig still has some features similar to C for high-performance and low-level applications, especially for systems programming. With the help of modern compiler technologies and optimizations, Zig correctly balances high performance and low overhead, which allows a developer to interact with low-level hardware and even do the manual memory allocation if needed.
// Allocate memory for one u8
const mem = try std.heap.alloc(u8, 1);
defer std.heap.free(mem);
// Use the allocated memory address
const ptr = mem;
In addition to this, Zig’s ability to run compile-time code execution, which enables the calculations and optimization to be carried out before the actual execution, gives rise to a possibility for performance improvement and lowers overhead at run time.
const values = [4]u8{ 1, 2, 3, 4};
const sum = comptime: {
var result: u8 = 0;
for (values) |value| {
result += value;
}
break:compile result;
};
In this case, the total value of the values array is computed at compile time, thereby allowing the compiler to perform optimizations and eliminate the extra computation throughputs at run time.
Simplicity, Readability, and Modern Features
Zig has advantages like focusing on simplicity and readability, and it holds its place above C and C++. Zig aims for a simpler and more approachable syntax compared to C++, achieved through language design choices such as:
- There are no header files and no preprocessor directives.
- No default memory management process is required because it is an automatic task.
- There is no need to bring up any makefiles or linker scripts instead.
- There is no distinction between declarations and definitions.
Such design choices make the zig code more accessible for developers to read and decipher, improving the code quality and contributing to the developers’ learning and maintenance of zig code.
Furthermore, Zig uses new packages to ensure that code is readable and maintainable, e.g., error unions and error sets. This approach is more expressive and safer than traditional error codes or global variables.
const Error = error{
OutOfMemory,
FileNotFound,
};
fn readFile(path: []const u8) Error! []u8 {
Implementation goes here.
}
fn main()! void {
const contents = readFile("data.txt"). catch |err| {
Handle the error.
return err;
};
// Use contents
}
Thus, the readFile function returns an error union: Error![]u8 to the caller of the function using the catch syntax, which is both explicit and safer to handle errors compared to other error-handling mechanisms in C.
Compatibility with C and Cross-Platform Support
Another strong point of Zig is that it is fully compatible with C libraries. This compatibility allows for the extensive use of the already existing code written in C and gives Zig’s features a chance to brighten Ziger’s life. Zig is created to run with C, so developers are able to use C libraries without problems and reuse their C projects along with Zig code.
It is central to almost all development engineers who often want to interface with archaic virtual memories created or older libraries like C.
In addition, Zig offers multi-platform support, meaning it can run on various systems and architectures, including Linux and Windows, among many others. Zig’s flexibility proves it is a good choice for embedded systems development when multiple hardware platforms appear.
Here’s an example of Zig code targeting an embedded device, demonstrating its potential for low-level system programming:
const std = @import("std");
const gpio = @import("gpio.zig");
pub fn main()! void {
const led = try gpio.openPin(17, output);
defer led.deinit();
while (true) {
led.set();
std.time.sleep(1000000000); // 1 second
led.clear();
std.time.sleep(1000000000);
}
}
Zig controls a GPIO( General Purpose Input Output) pin on an embedded device in this code snippet, showcasing its suitability for low-level system programming tasks.
Tooling, Ecosystem, and Community Support
Zig, being a new language, has the advantage of having a great deal of interest and contributions from developers. Zig has a package manager (zig build), a build system, and complete documentation (Zig Language Documentation).
With these tools, which streamline the software development lifecycle and promote code sharing, you may speed up software development and improve its quality. This is a problem with the particular case of C, which relies on an increasing level of programmers’ manual interventions.
Besides, Zig’s community, consisting of people who are exploring the possibilities of using it, has been providing feedback on its future features and the idea in general. The more evident messages are the increased conversations on sites like Hacker News and Reddit, where viewers can adjust their perspectives on Zig in a way they never thought existed.
One commenter on Hacker News highlighted Zig’s potential for improving code quality:
“”I hope that projects like Zig will get more attention and help in improving the quality of the code base for systems programming by providing a modern alternative to C.””
Another commenter praised Zig’s simplicity and potential for teaching low-level programming concepts:
Looking at it from another angle, Zig seems to be the best language for teaching low-level programming concepts without exposing students to the complexity of C++.
The following comments highlight the rapidly expanding interest and affiliation of the developer’s community, which will eventually be one of the vital elements for long-lasting performance and Zig adoption.
Potential Use Cases and Applications
Given its unique combination of features, Zig presents a compelling choice for a wide range of applications, particularly in the realm of systems programming and performance-critical domains.
- Operating Systems and Kernels: The kernel-level features like the low-level control, performance, and safety of the Zig together make it easily implemented in intelligent operating systems, where reliability and efficiency are the most important things.
- Device Drivers and Embedded Systems: The given example confirms the cross-platform features and ability to develop low-level device drivers and embedded systems applications. It is no wonder that Zig is able to target different architectures, which require interaction and optimizations at the hardware level.
- Game Development: Zig’s performance utility and capability of close optimization make it a good fit for gaming, where it can be used for critical parts or lower-level engine functionalities.
- Scientific Computing and High-Performance Applications: Zig’s emphasis on stable and accurate code generation and its ability to interact directly with low-level hardware do make it a good option for mathematics, physical simulations, and other performance-demanding applications.
- Systems Programming and Low-Level Libraries: The open-source Zig programming language is a potential alternative for low-level library development, utilities, and system-programming applications that need a mixture of performance and reliability. Zig is fully compatible with the C libraries and has robust safety features, which makes it a good option for developing libraries, writing utilities, and other low-level essential applications.
While there are many suggested use cases, the general adoption of the language and its success in these areas depend on things like ecosystem maturity, community support, and real-world benchmark performance.’
Challenges and Future Outlook
Despite its promising features and potential, Zig faces several challenges that could impact its widespread adoption and success:
- Ecosystem Maturity: As a novel language, you should also know that the Zig ecosystem and, therefore, the availability of third-party libraries and tools are less developed than languages like C and C++. Thus, we will need to bridge this gap to attract more developers and establish a base of diverse apps.
- Mindshare and Adoption: In sectors where C++ and C predominate, persuading developers and organizations to shift to the new language simultaneously can be very difficult. Like any startup, getting Zig’s early adoption will be crucial to success, and we will need to overcome this inertia and convince people of the benefits that Zig offers.
- Performance Benchmarking: Although Zig claims to have better performance in comparison to a range of workloads and utilities, it will necessitate a completely independent and extensive evaluation, which needs to be performance benchmarked and compared with those of C, C++, and other languages to validate these claims and identify potential areas that require more attention.
- Community and Corporate Support: The sustainable future of Zig is stated when its community grows in numbers and in its contribution and influence, on top of getting corporate and public sector support. By keeping an ecosystem of developers, community members, and market partners active, we provide proper conditions for our project’s reach and growth.
However, even though Zig is undergoing difficulties because it has just come out, the evidence of growing interest and enthusiasm that the developers show about the language indicates that the language will likely succeed and carve out its niche within future systems programming languages. As Zig progresses in its march towards maturity, Zig may have a compelling go-to feature set of safety, productivity, and language construction that is modern at the expense of other choices for programming.
Conclusion
Zig is a compelling alternative to C, offering a unique blend of safety, performance, and modern language features. By addressing common pain points in C and C++ while preserving low-level control, Zig positions itself as a valuable tool for systems programming, embedded development, and performance-critical applications.
Incorporating robust safety mechanisms like automatic memory management and null safety, Zig aims to eliminate entire classes of bugs prevalent in C/C++ code without compromising performance. Its simplicity, innovative features, and focus on code maintainability further enhance developer productivity. The main difficulties still need to be solved in developing and adopting the ecosystem. However, community involvement is a sign that Zig already has a chance of creating a niche in system-oriented programming languages.
FAQs on Zig – The Alternative of C
Is Zig production-ready?
Yes, Zig reached production-ready status with version 0.11.0, released in February 2023. However, its ecosystem is still actively developing and maturing.
How does Zig’s performance compare to C and C++?
Zig aims to match or exceed the performance of C and C++, leveraging modern compiler optimizations and low-level control.
Can Zig replace C for systems programming?
Zig is designed to potentially replace C in systems programming tasks, offering safety and modern features while retaining C’s performance.
Is Zig harder to learn than C?
Many developers find Zig easier to learn than C or C++, thanks to its simpler syntax and lack of unnecessary complexity.
What platforms and architectures does Zig support?
Zig supports a wide range of platforms, including Windows, macOS, Linux, ARM, x86, RISC-V, and more, enabling cross-platform development.
Share your thoughts in the comments
Please Login to comment...