When rendering a list and what is a key and it’s purpose ?
Last Updated :
08 Dec, 2023
Whenever we render a list in React using the map method which is mostly preferred, we need to provide the keys. If we do not provide a key during rendering we get a warning in our react application. To remove that warning and optimize our code we provide a key.
Prerequisites:
Keys are something that helps react to identify which items have changed, are added, or are removed. Keys should be given to the elements inside the array to give the elements a stable identity while they are rendered.
Purpose of Keys:
- To provide a unique identity to each list element from lists.
- To remove the warning or error that reacts if the key is not used.
- It is used to identify which items have changed, updated, or deleted from the Lists.
- It is useful when a user alters the list.
- It helps react to determine which component to be re-rendered.
Steps to create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app example
Step 2: After creating your project folder i.e. example, move to it using the following command:
cd example
Project Structure:
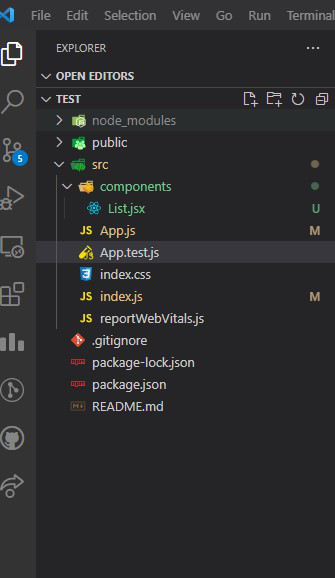
Folder Structure
Example 1: Basic example of rendering a list. Write down the following code in App.js, and List.jsx file.
Javascript
import React from 'react' ;
import List from './components/List' ;
const numbers = [1, 2, 3, 4, 5];
function App() {
return (
<div className= "App" >
<List numbers={numbers} />
</div>
);
}
export default App;
|
Javascript
import React from "react" ;
function List(props) {
const numbers = props.numbers;
const listItems = numbers.map((number,index) =>
<li>{number}</li>);
return (
<>
<div>List of numbers</div>
<ul>{listItems}</ul>
</>
);
}
export default List;
|
Step to run the application: Run the following command from the root directory of the project.
npm start
Output:
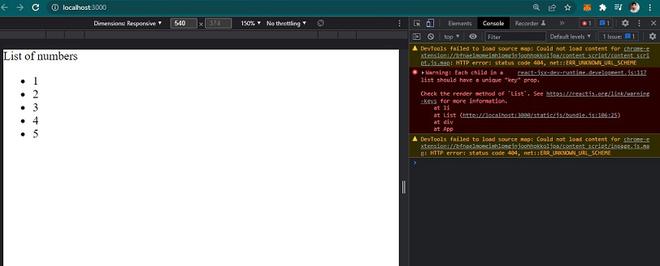
List without key
Example 2: Using key attributes in the list
List.jsx
import React from "react" ;
function List(props) {
const numbers = props.numbers;
const listItems = numbers.map((number,index) =>
<li key={index}>{number}</li>);
return (
<>
<div>List of numbers</div>
<ul>{listItems}</ul>
</>
);
}
export default List;
|
Output:
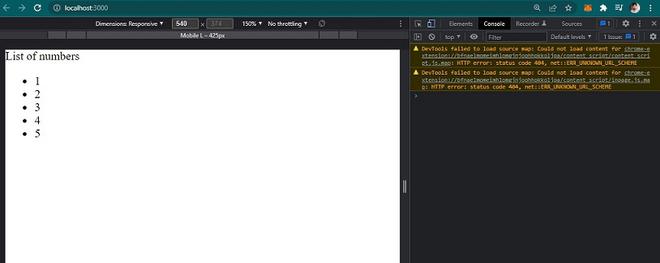
List with key
Share your thoughts in the comments
Please Login to comment...