What is the Event Driven Programming Paradigm ?
Last Updated :
02 Feb, 2024
Event-driven programming is a paradigm where the execution of a program is determined by events such as user actions or messages. Programs respond to events with predefined actions, allowing for asynchronous and responsive behavior, often seen in GUI applications and distributed systems.
Advantages of Event-Driven Programming Paradigm
- Enables asynchronous processing, optimizing resource utilization and responsiveness, crucial for real-time applications and user interfaces.
- Encourages modular code design, simplifying maintenance and scalability by separating concerns and promoting code reusability.
- Enhances user experience by responding promptly to user inputs, delivering a smoother and more interactive interface.
- Facilitates easier integration of new features or modifications, promoting adaptability to changing requirements in dynamic environments.
- Components communicate through events, reducing dependencies and enhancing system flexibility, making it easier to maintain and modify.
Disadvantages of Event-Driven Programming Paradigm
- Event-driven systems can be challenging to debug due to their asynchronous nature, making it harder to trace errors.
- Concurrent events may introduce race conditions, leading to unpredictable behavior and making debugging and synchronization complex.
- Event-driven systems may lead to inversion of control, making code harder to follow and understand for developers unfamiliar with the design.
- A series of interconnected events can lead to cascading effects, making it harder to predict the outcome and manage the system state.
- Continuous listening for events can consume system resources, leading to potential inefficiencies in resource utilization and impacting overall system performance.
Event-Driven Architecture (EDA)
Event-driven architecture (EDA) responds to actions like button clicks. In this paradigm, events, such as “buttonClick,” trigger predefined actions. The user interacts with the interface, and the system, following the Event-Driven Programming model, responds dynamically to ensure a responsive and engaging user experience.
Example: To demonstrate basic event handling through a JavaScript EventDispatcher, prompting an alert using Event-Driven Architecture.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Event-Driven Architecture Example</ title >
< style >
body {
margin: 0 auto;
}
.container {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
h1 {
color: green;
}
#myButton {
width: 102px;
height: 40px;
background: #6583b9;
border: none;
color: white;
border-radius: 5px;
font-size: 16px;
}
#myButton:hover {
scale: 1.03;
background: skyblue;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 >GeeksforGeeks</ h1 >
< button id = "myButton" >Click me!</ button >
</ div >
< script >
class Event {
constructor(name) {
this.name = name;
}
}
class EventDispatcher {
constructor() {
this.listeners = {};
}
addListener(eventName, callback) {
if (!this.listeners[eventName]) {
this.listeners[eventName] = [];
}
this.listeners[eventName].push(callback);
}
dispatchEvent(event) {
const eventName = event.name;
if (this.listeners[eventName]) {
this.listeners[eventName]
.forEach(callback => callback(event));
}
}
}
const eventDispatcher = new EventDispatcher();
eventDispatcher.addListener("buttonClick", (event) => {
console.log(`Button Clicked! (${event.name})`);
alert("Button Clicked!");
});
document.getElementById("myButton")
.addEventListener("click", () => {
const buttonClickEvent = new Event("buttonClick");
eventDispatcher.dispatchEvent(buttonClickEvent);
});
</ script >
</ body >
</ html >
|
Output:
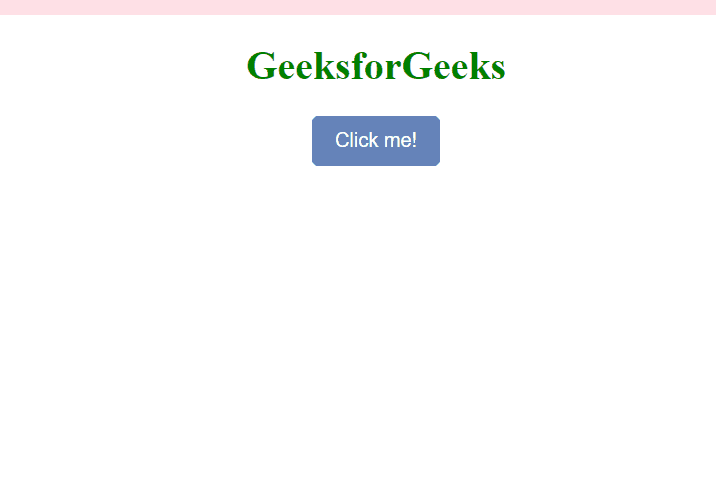
Callback Functions in event-driven programming paradigm
In the event-driven programming paradigm, we are using callback functions. This entails defining functions to handle specific events, such as button clicks. By registering these callbacks with event listeners, the program responds dynamically to user actions, embodying the essence of event-driven architecture for interactive and responsive applications.
Example: To demonstrate the core principles of event-driven programming for interactive user experiences using callback functions.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
\ initial-scale = 1 .0">
< title >Event-Driven Architecture Example</ title >
< style >
body {
margin: 0 auto;
}
.container {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
h1 {
color: green;
}
#myButton {
width: 102px;
height: 40px;
background: #6583b9;
border: none;
color: white;
border-radius: 5px;
font-size: 16px;
}
#myButton:hover {
scale: 1.03;
background: skyblue;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 >GeeksforGeeks</ h1 >
< button id = "myButton" >Click me!</ button >
</ div >
< script >
// Callback function to handle button click event
function handleButtonClick() {
console.log("Button clicked!");
alert("Button clicked!");
}
// Adding an event listener to the button
document.getElementById("myButton")
.addEventListener("click", handleButtonClick);
</ script >
</ body >
</ html >
|
Output:
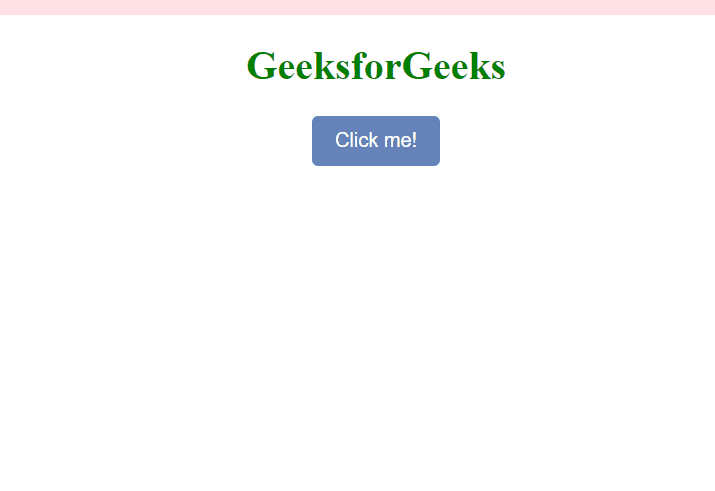
Share your thoughts in the comments
Please Login to comment...