What is the Equivalent of $(document).ready in JavaScript ?
Last Updated :
14 Dec, 2023
The jQuery function “$(document).ready()” makes sure that code is only performed when the DOM has completely loaded, preventing untimely modification. It enables event binding, safe DOM element interaction, and the execution of DOM-dependent code. ensures code executes when it should, preventing mistakes caused by inaccessible parts.
When using jQuery is out of option and we want to get the same result without using jQuery, then we can use two approaches:
Approach 1: Using the “DOMContentLoaded” event
In this approach, we use the DOMContentLoaded event and in this, we can use it with both the window and the document. Without waiting for stylesheets, graphics, or subframes to fully load, the DOMContentLoaded event fires once the basic HTML content has loaded and been parsed. So, it gives us the same type of functionality that is provided by $(document).ready() with jQuery.
Syntax:
document.addEventListener("DOMContentLoaded", function() {
// Code to be executed when the DOM is ready
});
// Or we can also use this event in this way
window.addEventListener("DOMContentLoaded", function() {
// Code to be executed when the DOM is ready
});
Example: This example demonstrates how we can implement the first approach which is using the DOMContentLoaded event with a document and with a window. Here, it is demonstrated how when we add the DOMContentLoaded event with the document, the script is executed when the DOM is loaded.
HTML
<!DOCTYPE html>
< html >
< head >
< script >
//When the DOMContentLoaded event is not
// used, the script is like this
// This will not be executed
document
.addEventListener("DOMContentLoaded",
function () {
// Code to be executed when the DOM is ready
let heading = document
.getElementById("myHeading");
heading.textContent = "DOM is ready!";
});
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
$(document).ready equivalent without jQuery
</ h3 >
< h1 id = "myHeading" >
Waiting for DOM...
</ h1 >
</ body >
</ html >
|
Output:
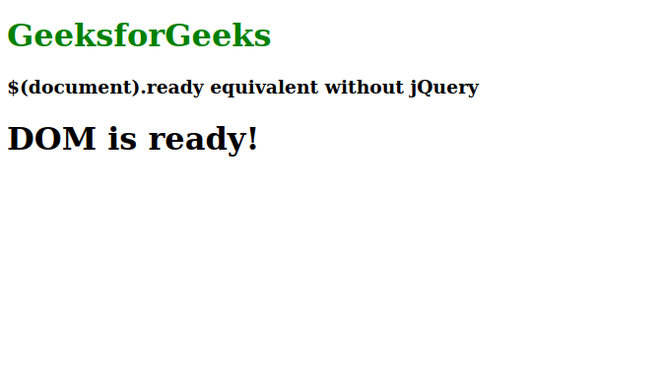
Approach 2: Deferred loading
This method ensures that the JavaScript code inside the script tag is run after all of the HTML content has been loaded and parsed by positioning the <script> tag immediately before the closing </body> element at the conclusion of the HTML body. The code will thereafter have access to all DOM elements in this manner.
Example: The code example below demonstrates how we can implement the second approach. This code shows how we can actually use this approach to mimic the functionality of the $(document).ready() without using the DOMContentLoaded event.
HTML
<!DOCTYPE html>
< html >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
$(document).ready
equivalent without jQuery
</ h3 >
< strong style="color: sienna;
margin: 2rem;" id = "myText" >
DOM not loaded yet...
</ strong >
< br >
< strong style="color: sienna;
margin-left: 2rem;" id = "myText2" >
</ strong >
< script >
let text = document
.getElementById("myText");
let text2 = document
.getElementById("myText2");
text.textContent =
"In this code same result is achieved using";
text2.textContent =
"Using the second aprroach!";
</ script >
</ body >
</ html >
|
Output:
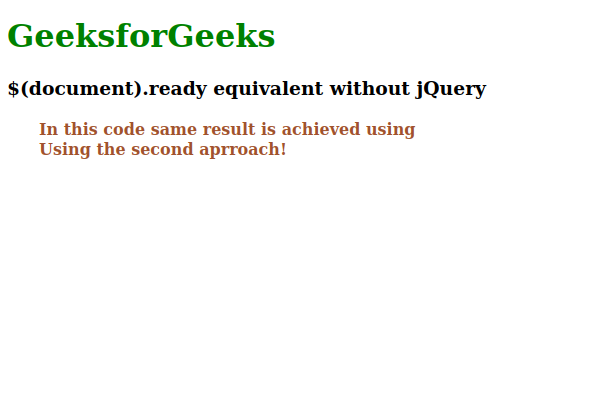
Share your thoughts in the comments
Please Login to comment...