What is JavaScript Rendering ?
Last Updated :
26 Apr, 2024
Javascript rendering refers to the process of dynamically updating the content of a web page using JavaScript. This process also known as client-side rendering, means that it generates Html content dynamically on the user’s web browser. In this tutorial, we will see the process of JavaScript rendering.
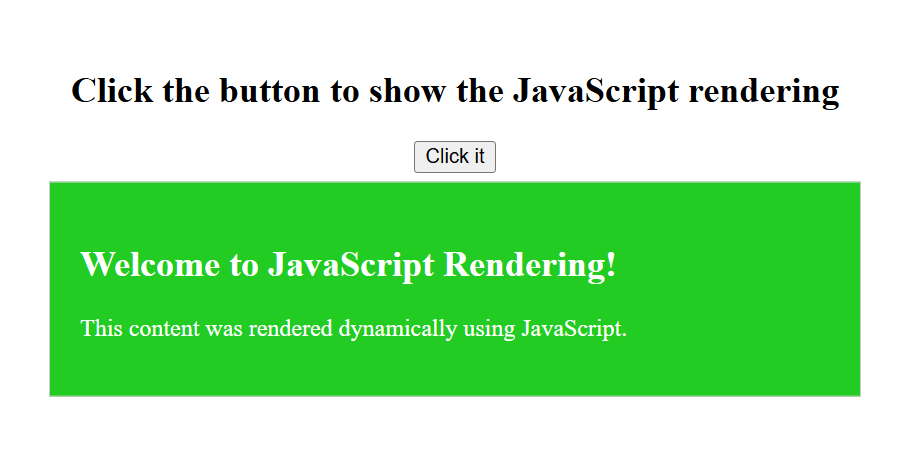
Preview
Impact of JavaScript Rendering on SEO
Javascript rendering can have a significant impact for Search Engine Optimization (SEO) because search engines need to crawl and index the content of web pages to rank them in search results.
There are many key impacts of JavaScript rendering on SEO, Some of them are:
- Initial Rendering: If the content is generated dynamically using javascript then web crawlers may or may not see the fully render content. So it can be hamper of our web page in indexing.
- Time to Render : If we render some content dynamically then it can take more time to load and render then those page which are fully html based. Then this slow-loading page can negatively impact on SEO.
- Content Accessibility : If our web page generate content dymanically then if a user search for a content which is also present in our page but it is generate dynamically by the user action like “click” the button etc, then it can impact on SEO.
Techniques to improve JavaScript Rendering
Improving javascript rendering in term of preformence of a web applications is a good task.
Here are several techniques to enhance JavaScript rendering:
- Code Optimization: Write clean, efficient JavaScript code to reduce execution time and minimize browser overhead.
- Lazy Loading: We can use the lazy loading process for our JavaScript resources and components.For that you can load javascript files asynchronously. And you can reduce initial page load times and improving overall performance.
- Use Server-Side Rendering (SSR): We can implement SSR to generate HTML content on the server and can send pre-rendered pages to the client.This ca help to improve SEO, because the content is available immediately without requiring client-side rendering.
JavaScript client-side rendering
- At first create a HTML structure with a basic structure with a container div and an output div.
- The container div contains a heading, a button, and an output div where we show the dynamic content using the javascript rendering.
- In the javascript get the reference of the needed tags and elements. Because the JavaScript is used to handle user interaction and dynamically render content.
- Then attached an event listener to the button and handle the “click” event for update the content when the button is clicked.
- You can render and update the dynamic content by the help of javascript DOM (Document Object Model) manipulation.
Example : To demonsrtate the Client side rendering in JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>JavaScript Rendering Example</title>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
margin-top: 10rem;
}
#output {
padding: 20px;
background-color: #22cc22;
border: 1px solid #ccc;
width: 500px;
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
margin-top: 30px;
color: #fff;
}
</style>
</head>
<body>
<div class="container">
<h2>Click the button to show the JavaScript rendering</h2>
<button class="btn">Click it</button>
</div>
<div id="output">
<!-- Content will be rendered here dynamically -->
</div>
<script>
let outputDiv = document.getElementById('output');
let btn = document.querySelector(".btn");
// Generate some dynamic content
let dynamicContent = "<h2>Welcome to JavaScript Rendering!</h2>";
dynamicContent += "<p>This content was rendered dynamically using JavaScript.</p>";
// Set the content of the output div to the dynamic content
btn.addEventListener("click", function () {
outputDiv.innerHTML = dynamicContent;
outputDiv.style.display = "block";
});
</script>
</body>
</html>
Output:
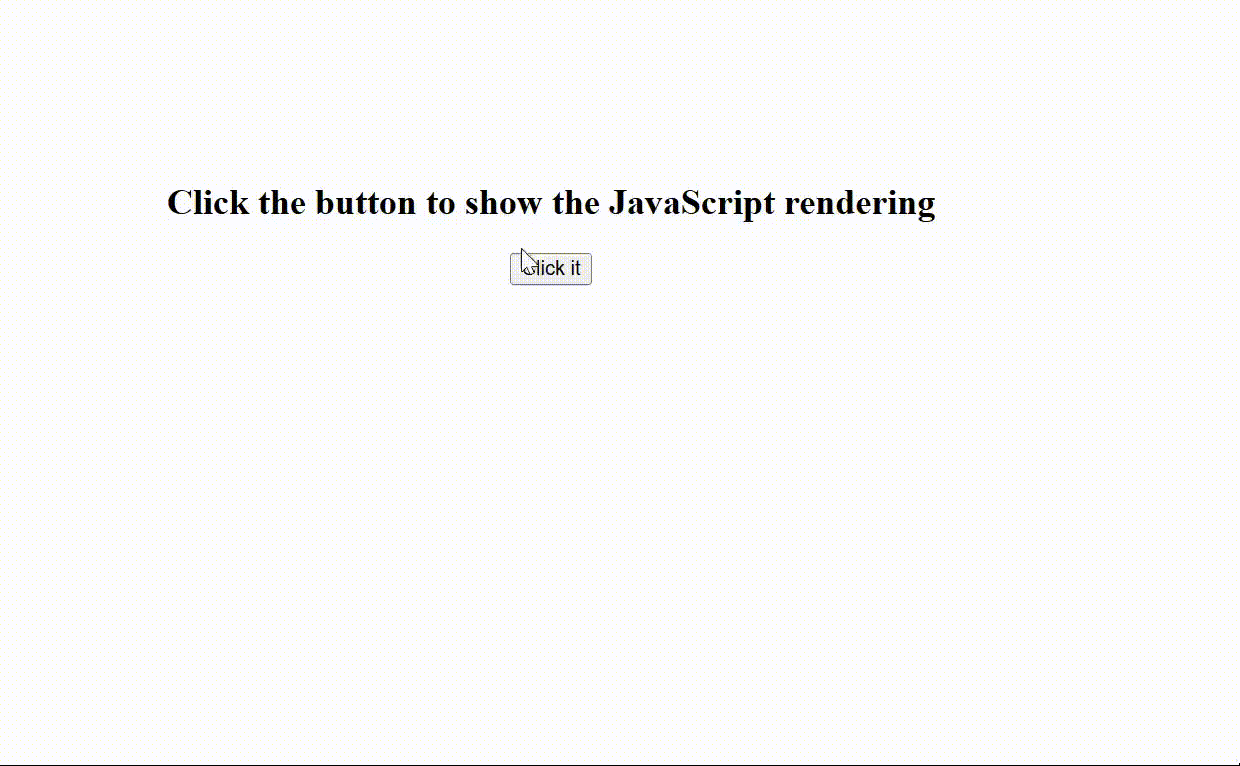
Output : JavaScript rendering
Share your thoughts in the comments
Please Login to comment...