A memory pool, also known as a memory allocator or a memory management pool, is a software or hardware structure used to manage dynamic memory allocation in a computer program. It is a common technique used to efficiently allocate and deallocate memory for data structures and objects during program execution. It is a pre-allocated region of memory that is divided into fixed-size blocks. Memory pools are a form of dynamic memory allocation that offers a number of advantages over traditional methods such as malloc and free.
A memory pool is a logical division of main memory or storage that is reserved for processing a job or group of jobs
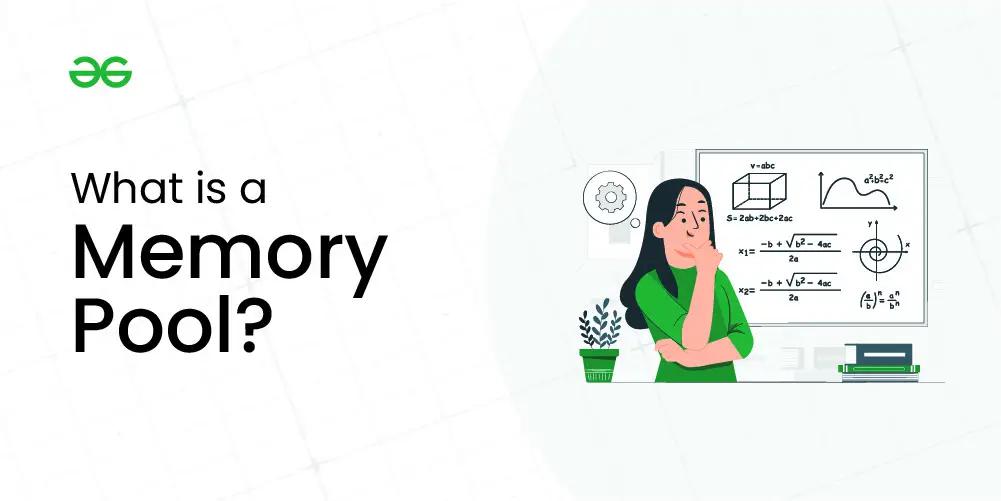
Important Topics for Memory Pool
Types of Memory Pools
There are many types of memory pools:
- Fixed-size Memory Pool: In a fixed-size memory pool, all allocated blocks are of the same size. These pools are simple and efficient for managing objects of uniform size, It is commonly used in embedded systems and real-time applications
- Variable-size Memory Pool: In a variable-size memory pool, blocks can be of different sizes. They are more flexible than fixed-size pools and are useful for managing objects of varying sizes. They are often used in general-purpose memory allocation libraries
- Thread-local Memory Pool: Thread-local memory pools are designed for multi-threaded applications where each thread has its own memory pool. This approach reduces disputes for memory allocation and deallocation operations between threads
- Stack-based Memory Pool: A stack-based memory pool follows a last-in, first-out (LIFO) allocation and deallocation strategy. It is well-suited for scenarios where temporary data is frequently pushed and popped from the stack
- Garbage-collected Memory Pool: Garbage-collected memory pools include automatic memory management, often used in programming languages with garbage collection (e.g., Java, C#). They help manage memory by reclaiming objects that are no longer in use
- Real-time Memory Pool: Real-time memory pools are designed for applications with strict timing constraints. They prioritize predictable and deterministic memory allocation and deallocation
What are Memory Allocation and Deallocation
Memory Allocation
Memory allocation is the process of reserving a portion of a computer’s memory for the use of a program. It involves setting aside a block of memory to store data, variables, objects, or other information that the program requires. The allocated memory can be used to create data structures, arrays, variables, and other dynamic entities. Memory allocation is typically achieved using functions or system calls provided by the programming language or the operating system, for eg. “malloc”, “new”, etc.
Memory Deallocation
Memory deallocation, also known as memory release or freeing memory, is the process of releasing previously allocated memory that is no longer needed. It is essential to prevent memory leaks and ensure efficient use of system resources. When memory is deallocated, it becomes available for reuse by the program or the operating system. Common memory deallocation functions and techniques include: “free”, “delete”, etc.
Proper memory allocation and deallocation are critical to preventing memory leaks, improving program performance, and ensuring efficient use of memory resources. Failure to deallocate memory can lead to memory leaks, where the program consumes increasing amounts of memory over time, potentially causing it to crash or become sluggish
Memory pool allocation algorithms
Memory pool allocation algorithms are used to efficiently manage memory allocation within memory pools. These algorithms determine how memory blocks are allocated and deallocated from a memory pool to optimize performance and resource utilization. The choice of allocation algorithm depends on the specific use case and requirements of the application. Some of the commonly used algorithms are:
- First-fit: The first-fit algorithm allocates the first available memory block in the pool that is large enough to accommodate the requested size, It is simple and fast but can lead to fragmentation over time
- Best Fit: The best-fit algorithm searches for the smallest available memory block that can accommodate the requested size. It aims to minimize fragmentation, but it may be slower than first-fit due to the need to search for the best-fit block.
- Worst Fit: The worst-fit algorithm allocates the largest available memory block, which can help reduce fragmentation. However, it may lead to less efficient use of memory.
- Buddy System: The buddy system allocates memory blocks in sizes that are powers of two. When a block is allocated, it is split into two smaller “buddy” blocks. When a block is deallocated, the buddy blocks are merged back together. This algorithm helps prevent external fragmentation.
- Segregated Lists: Segregated lists maintain multiple lists, each containing memory blocks of a specific size range. When an allocation request is made, the algorithm selects the appropriate list based on the requested size and allocates from the corresponding list. This approach can reduce fragmentation and improve allocation speed.
- Slab Allocation: Slab allocation divides memory into fixed-size slabs, and each slab is further divided into fixed-size objects. When an allocation is requested, the algorithm allocates from an appropriate slab. It is commonly used in the Linux kernel and helps reduce fragmentation.
What is Fragmentation and Garbage Collection?
Fragmentation is a common concern in memory management, and it becomes especially relevant when dealing with memory pool management. Memory fragmentation refers to the phenomenon where available memory becomes divided into small, non-contiguous blocks, making it challenging to allocate large contiguous blocks of memory. fragmentation can be of two types:
External Fragmentation
External fragmentation occurs when free memory blocks are scattered throughout the memory pool, with used and unused blocks interleaved. This type of fragmentation can lead to inefficient use of memory because it may be challenging to allocate large contiguous blocks, even if there is sufficient free memory. External fragmentation can result from the allocation and deallocation of memory blocks over time, leaving gaps in the memory pool.
Internal Fragmentation
Internal fragmentation occurs when allocated memory blocks are larger than the amount of data they hold. This wasted space within allocated blocks is inefficient. Internal fragmentation can result from memory pools that allocate fixed-size blocks, where the allocated blocks are often larger than the actual data they store.
Garbage collection
Garbage collection is an automatic memory management technique used in many programming languages to reclaim memory occupied by objects that are no longer in use. It is designed to reduce the burden of manual memory allocation and deallocation, reducing the risk of memory leaks and making memory management more convenient for developers
How memory pools are implemented?
Memory pools are implemented using a combination of data structures and algorithms to manage the allocation and deallocation of memory in a structured and efficient manner. The specific implementation details can vary depending on the language, platform, and use case, but the following are common components and steps in implementing memory pools:
- Initialization: Allocate a large, continuous block of memory from the system, which serves as the memory pool. Divide this memory into smaller blocks or chunks of a fixed or variable size, depending on the requirements of the application.
- Data Structures: Use data structures to manage the allocation status of each block in the memory pool. Common data structures include linked lists, bitmaps, or arrays.
- Allocation Algorithm: Implement an allocation algorithm to determine which block to allocate when a memory request is made. Update the data structure to mark the allocated block as in use.
- Deallocation Algorithm: Implement a deallocation algorithm to release memory blocks that are no longer needed. Update the data structure to mark the deallocated block as free.
- Block Coalescing: In some memory pool implementations, adjacent free blocks are combined (coalesced) to form larger free blocks. This helps minimize fragmentation and optimize memory utilization.
- Thread Safety: If the application is multi-threaded, implement thread safety mechanisms to ensure that multiple threads can allocate and deallocate memory without conflicts or data corruption.
- Error Handling: Implement error-handling mechanisms to handle cases where memory is exhausted or allocation requests fail. This can include returning NULL (in C/C++), raising exceptions (in languages like Java or Python), or other custom error handling approaches.
- Customization and Tuning: Change the memory pool implementation to the specific requirements of the application. This might include adjusting block sizes, allocation algorithms, or other parameters to optimize memory usage and performance.
- Memory Pool Management: Manage the memory pool’s lifecycle, including creation, destruction, resizing (if supported), and deallocation of the entire memory pool when it is no longer needed.
- Documentation and Testing: Thoroughly document the memory pool’s behavior and provide clear guidelines for developers who will use it. Conduct testing to ensure the memory pool functions correctly, handles edge cases, and performs efficiently.
Use cases for memory pools
Memory pools can be used in a wide variety of applications. Some common use cases for memory pools include:
- Game development
- Network servers
- Databases
- Embedded systems
- CXL with memory pools
CXL in Memory Pools
Compute Express Link, is an emerging high-speed interconnect technology that enables efficient communication between various system components, such as CPUs, GPUs, and memory devices. It extends the capabilities of PCIe (Peripheral Component Interconnect Express) by allowing for high-bandwidth, low-latency connections between devices in a heterogeneous computing environment.
Memory pools, on the other hand, are a memory management technique used to efficiently allocate and deallocate memory in software. When combined with CXL, memory pools can benefit from the increased bandwidth and lower latency provided by CXL’s high-speed links. This can lead to more efficient memory allocation and management, particularly in applications with diverse memory requirements, such as real-time systems, where predictable and fast memory operations are essential.
Advantages of memory pools
- Faster memory allocation and deallocation.
- Reduced memory fragmentation.
- Predictable memory usage.
- Improved performance and stability.
Disadvantages of memory pools
- Limited flexibility for variable-sized allocations.
- Complexity in managing multiple memory pools.
- Potential for memory leaks if not used carefully.
Guidelines for effective use of memory pools
- Use memory pools for objects that are frequently allocated and deallocated.
- Choose the right allocation algorithm for the application.
- Use thread-safe memory pools in multithreaded applications.
- Use memory pool management techniques to reduce fragmentation.
Alternatives to Memory Pools
While memory pools can be a useful memory management technique in certain scenarios, there are alternative approaches to managing memory in a software application, Such as:
- Malloc and Free: Traditional dynamic memory allocation using functions like malloc and free in C and C++ or similar memory allocation and deallocation functions in other languages. This approach is flexible but can lead to fragmentation.
- Smart Pointers: In languages like C++, you can use smart pointers to manage memory automatically, which helps prevent memory leaks.
- Garbage Collection: Automatic memory management techniques, as seen in languages like Java and C#, use garbage collection to automatically free memory when objects are no longer in use.
- Stack Allocation: Allocate memory on the stack for local variables with automatic storage duration. The memory is automatically reclaimed when the variable goes out of scope. This is fast but limited in size.
- Memory Mapping: For specific use cases, you can use memory mapping techniques to map files or shared memory regions into the process’s address space, allowing for efficient memory access.
Security and safety of memory pools
Memory pools can help to improve the security and safety of applications by reducing the risk of memory errors. Memory errors can be caused by a number of factors, such as buffer overflows and use-after-free vulnerabilities. Memory pools can help to reduce the risk of these errors by ensuring that memory is allocated and deallocated in a controlled manner.
If you have the flexibility to choose the programming language for your project, consider modern languages like Rust or languages with built-in memory safety features (e.g., C# with the .NET memory management). These languages can help prevent common memory-related issues and provide a safer environment for memory pool management, reducing the chances of memory leaks and vulnerabilities.
Conclusion
Memory pools are a structured way to allocate and deallocate memory, reduce fragmentation, and improve the overall efficiency of memory usage in software applications. They provide faster memory allocation and deallocation, reduced memory fragmentation, and predictable memory usage, leading to improved performance and stability. However, they have limited flexibility for variable-sized allocations, add complexity in managing multiple memory pools, and have the potential for memory leaks if not used carefully. Despite these drawbacks, memory pools are a valuable tool for optimizing memory management in software applications
Share your thoughts in the comments
Please Login to comment...