What are Filters in Bootstrap ?
Last Updated :
15 Feb, 2022
Using Bootstrap we can search or filter out the required output that we want to be displayed when searching for a particular item/word on our website. Although Bootstrap does not provide us with a component or utility that allows filtering of elements. For that purpose, we have to use jQuery to filter out or for searching purposes.
In this article, we would be discussing some filter methods that we can use on our application.
Bootstrap filter table: You can filter table elements by using Bootstrap and jQuery together on the table body excluding the table headers. Case-insensitive filtering can be done.
Example: The code below has been implemented on the table for filtering elements.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Filters in Bootstrap</ title >
< meta charset = "utf-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 ">
< link rel = "stylesheet" href =
< script src =
</ script >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< div class = "container mt-3" >
< h2 >Bootstrap filter for table</ h2 >
< input type = "text"
class = "form-control"
placeholder = "Search Here"
id = "txtInputTable" >
< br >
< table class = "table table-bordered" >
< thead >
< tr >
< th >Id</ th >
< th >Name</ th >
< th >Email</ th >
< th >Phone No.</ th >
</ tr >
</ thead >
< tbody id = "tableDetails" >
< tr >
< td >101</ td >
< td >Ram</ td >
< td >ram@abc.com</ td >
< td >8541236548</ td >
</ tr >
< tr >
< td >102</ td >
< td >Manish</ td >
< td >manish@abc.com</ td >
< td >2354678951</ td >
</ tr >
< tr >
< td >104</ td >
< td >Rahul</ td >
< td >rahul@abc.com</ td >
< td >5789632569</ td >
</ tr >
< tr >
< td >105</ td >
< td >Kirti</ td >
< td >kirti@abc.com</ td >
< td >5846325968</ td >
</ tr >
</ tbody >
</ table >
</ div >
< script >
$(document).ready(function () {
$("#txtInputTable").on("keyup", function () {
var value = $(this).val().toLowerCase();
$("#tableDetails tr").filter(function () {
$(this).toggle($(this).text()
.toLowerCase().indexOf(value) > -1)
});
});
});
</ script >
</ body >
</ html >
|
Output:
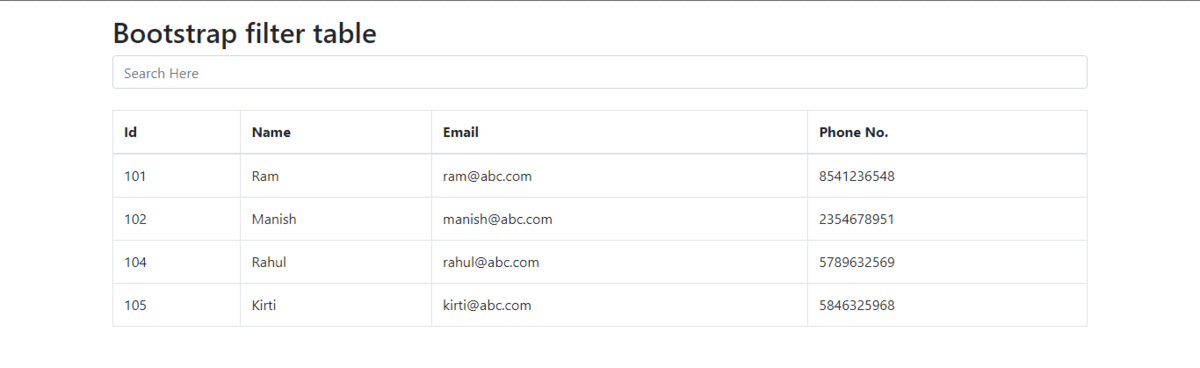
Bootstrap filter table
Bootstrap filter anything: We can filter anything that is present on our website whether it be a table, list, button, paragraph, or anything.
Example: The code below has been implemented to filter anything that is present on your website.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Filters in Bootstrap</ title >
< meta charset = "utf-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1" >
< link rel = "stylesheet"
href =
< script src =
</ script >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< div class = "container mt-3" >
< h2 >Bootstrap filter for anything</ h2 >
< input class = "form-control"
id = "txtInputAnything"
type = "text"
placeholder = "Search Here" >
< div id = "myWeb" class = "mt-3" >
< p >Type a paragraph which you want to find</ p >
< button class = "btn btn-secondary" >First button</ button >
< div >Search anything you want</ div >
< p >Another paragraph.</ p >
< button class = "btn btn-danger" >Another button</ button >
</ div >
</ div >
< script >
$(document).ready(function () {
$("#txtInputAnything").on("keyup", function () {
var value = $(this).val().toLowerCase();
$("#myWeb *").filter(function () {
$(this).toggle($(this).text()
.toLowerCase().indexOf(value) > -1)
});
});
});
</ script >
</ body >
</ html >
|
Output:
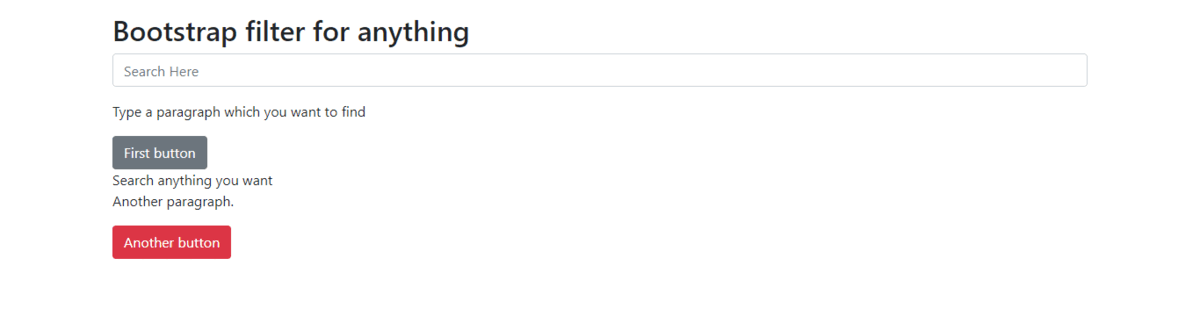
Bootstrap filter anything
Bootstrap filter list: It is similar to table filtering and works on lists. There is usually no difference between table filtering and list filtering.
Example: The code below has been implemented on the list for filtering items.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Filters in Bootstrap</ title >
< meta charset = "utf-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1" >
< link rel = "stylesheet"
href =
< script src =
</ script >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< div class = "container mt-3" >
< h2 >Bootstrap filter for list</ h2 >
< input class = "form-control"
id = "txtInputList"
type = "text"
placeholder = "Search Here" >
< br >
< ul class = "list-group" id = "myList" >
< li class = "list-group-item" >First list item</ li >
< li class = "list-group-item" >Second list item</ li >
< li class = "list-group-item" >Third list item</ li >
< li class = "list-group-item" >Fourth list item</ li >
< li class = "list-group-item" >Sixth list item</ li >
< li class = "list-group-item" >Seventh list item</ li >
</ ul >
</ div >
< script >
$(document).ready(function () {
$("#txtInputList").on("keyup", function () {
var value = $(this).val().toLowerCase();
$("#myList li").filter(function () {
$(this).toggle($(this).text()
.toLowerCase().indexOf(value) > -1)
});
});
});
</ script >
</ body >
</ html >
|
Output:
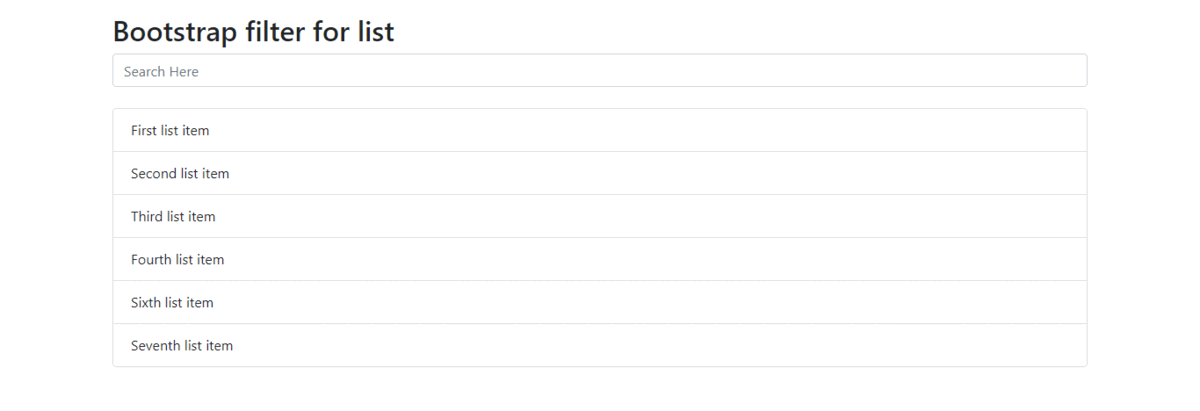
Bootstrap filter list
Share your thoughts in the comments
Please Login to comment...