TypeScript OmitThisParameter<Type> Utility Type
Last Updated :
24 Oct, 2023
In this article, we are going to learn about OmitThisParameter<Type> Utility Type in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, the OmitThisParameter<Type> utility type is used to create a new function type that is the same as the input Type but with this parameter removed. This can be useful when you want to remove the context parameter (this) from a function type.
Syntax
type NewFunctionType = OmitThisParameter<Type>;
Where-
- NewFunctionType is the name you choose for the new function type without this parameter.
- OmitThisParameter is the utility type used to omit this parameter.
- Type in the function is type from which you want to remove this parameter.
Approach
Let us see how we can use the OmitThisParameter<Type> step-by-step:
Step 1: Define the Original Function Type, Start by defining the original function type for which you want to omit this parameter:
type OriginalFunctionType = (this: { message: string }, name: string) => void;
Step 2: Apply the OmitThisParameter<Type> utility to the original function type to create a new function type without this parameter:
type NewFunctionType = OmitThisParameter<OriginalFunctionType>;
Step 3: You can now use the new function type in various ways, such as defining functions, method signatures, or variables:
const greet: NewFunctionType = function (name) {
console.log(`${this.message}, ${name}!`);
};
const context = { message: "Hello" };
greet.call(context, "GeeksforGeeks");
Step 4: Now you can pass any type of parameter to that function, It will accept it all.
Example 1
In this example, we will create a function that will have it’s all its inherited properties instead of only this parameter type. First of all, we will define a function having this parameter and another string-type parameter. Now with the help of OmitThisParameter<Type> we will create another function omitting that ‘this’ parameter type which can accept any parameter having any type.
Javascript
type OriginalFunctionType =
( this : { message: string }, name: string) => void;
type NewFunctionType = OmitThisParameter<OriginalFunctionType>;
const greet: NewFunctionType = function (name) {
console.log(`${ this .message}, ${name}!`);
};
const context = { message: "Hello" };
greet.call(context, "GeeksforGeeks" );
|
Output
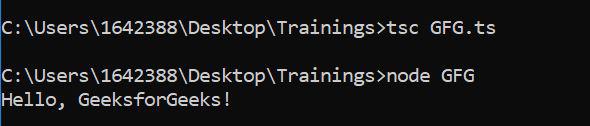
Example 2
In this example, we will create a function that will have it’s all its inherited properties instead of only this parameter type. First of all, we will define a function having this parameter and another number-type parameter. Now with the help of OmitThisParameter<Type> we will create another function omitting that ‘this’ parameter type which can accept any parameter having any type. In this function it will increment or add the value passed by the function and return it. Does not matter the type of value, If it a number it will add it to another one else it will concatinate as a string.
Javascript
type OriginalFunctionType =
( this : { value: number }, increment: number) => void;
type NewFunctionType = OmitThisParameter<OriginalFunctionType>;
const incrementValue: NewFunctionType = function (increment) {
this .value += increment;
};
const context = { value: 5 };
incrementValue.call(context, 3);
console.log(context)
|
Output

Share your thoughts in the comments
Please Login to comment...