TypeScript InstanceType<Type> Utility Type
Last Updated :
29 Oct, 2023
In this article, we are going to learn about InstanceType<Type> Utility Type in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, the InstanceType<Type> utility type is used to extract the instance type of a constructor function or class type. It allows you to infer the type of instances that can be created from a constructor or class.
Syntax
type T1 = InstanceType<Type>;
Parameters
- Type: This is the type parameter that represents the constructor function or class type from which you want to extract the instance type.
- T1: This is the name of the utility type that extracts the instance type.
Example 1
In this example,
- We have a Person class with a constructor that takes a name and an age.
- We use InstanceType<typeof Person> to extract the instance type of the Person class, which is equivalent to { name: string; age: number; }.
- We create an instance of Person using new Person(“Alice”, 30) and assign it to the person variable.
- TypeScript infers that person is of type PersonInstance, which allows us to access the name and age properties of the person object with type safety.
Javascript
class Person {
constructor(public name: string, public age: number) { }
}
type PersonInstance = InstanceType< typeof Person>;
const person: PersonInstance = new Person( "Alice" , 30);
console.log(person.name);
console.log(person.age);
|
Output
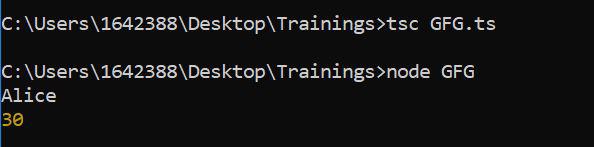
Example 2
In this example,
- We have a Product class with a constructor that takes a name and a price.
- We also have a ShoppingCart class that can hold instances of Product.
- InstanceType<typeof Product> is used to specify the type of products that can be added to the shopping cart.
- We create instances of Product (e.g., laptop and smartphone) and an instance of ShoppingCart (e.g., cart).
- We add products to the cart using the addItem method.
- We calculate the total price of the items in the cart using the getTotalPrice method
Javascript
class Product {
constructor(public name: string, public price: number) { }
}
class ShoppingCart {
private items: InstanceType< typeof Product>[] = [];
addItem(product: InstanceType< typeof Product>): void {
this .items.push(product);
}
getTotalPrice(): number {
return this .items.reduce((total, product
) => total + product.price, 0);
}
}
const laptop = new Product( "Laptop" , 1000);
const smartphone = new Product( "Smartphone" , 500);
const cart = new ShoppingCart();
cart.addItem(laptop);
cart.addItem(smartphone);
const totalPrice = cart.getTotalPrice();
console.log(`Total Price: $${totalPrice}`);
|
Output

Reference: https://www.typescriptlang.org/docs/handbook/utility-types.html#instancetypetype
Share your thoughts in the comments
Please Login to comment...