TypeScript Function
Last Updated :
06 Nov, 2023
TypeScript Functions are the most crucial aspect of TypeScript as it is a functional programming language. Functions are pieces of code that execute specified tasks. They are used to implement object-oriented programming principles like classes, objects, polymorphism, and abstraction. It is used to ensure the reusability and maintainability of the program.
Syntax:
function functionName(arg: argType) {
//function Body
}
Where:
- functionName: It is the name of the function
- arg: Argument Name
- argType: Type of the argument
TypeScript Function Types
- Parameter type annotations: We can specify the type of the parameter after the name of the parameter.
- Return type annotations: We can write the return type of the function post parameter list. This is called Return type annotation.
- Functions Which Return Promises: If a function is returning a Promise then you can write Promise<Type> as return type
- Anonymous Function: An anonymous function is a function without a name. At runtime, these kinds of functions are dynamically defined as an expression. We may save it in a variable and eliminate the requirement for function names. They accept inputs and return outputs in the same way as normal functions do. We may use the variable name to call it when we need it. The functions themselves are contained inside the variable.
Example 1:
Javascript
function greet(name: string) {
console.log( "Hello, " + name + "!!" );
}
greet( "GeeksforGeeks" )
|
Output:

Example 2:
Javascript
function greet(): string {
return ( "Hello, GeeksforGeeks!!" );
}
console.log(greet())
|
Output:

Example 3:
Javascript
async function greet(): Promise<string> {
return ( "Hello, GeeksforGeeks!!" );
}
greet().then(
(result) => {
console.log(result)
}
)
|
Output:

Example 4:
Javascript
let myFunction = function (a: number, b: number): number {
return a + b;
};
console.log(myFunction(7, 5));
|
Output:
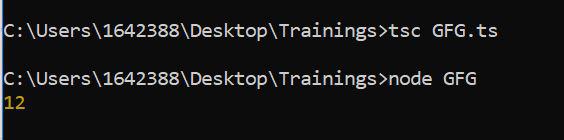
Conclusion: In this article we have seen the functions in typescript that whar are the types of fucntion with code examples. It makes easy to deal with the code and helps in code reusability and readability.
Share your thoughts in the comments
Please Login to comment...