TypeScript Exclude<UnionType, ExcludedMembers> Utility Type
Last Updated :
18 Oct, 2023
In this article, we will learn about Exclude<UnionType, ExcludedMembers> Utility Type in Typescript. In TypeScript, the Exclude<UnionType, ExcludedMembers> utility type is used to create a new type by excluding specific members (types) from a union type. It allows you to narrow down a union type by removing certain members to create a more specific type.
Syntax
type ExcludedType = Exclude<UnionType, ExcludedMembers>;
Where,
- ExcludedType: The name of the new type that will contain the members from UnionType excluding the ExcludedMembers.
- UnionType: The original union type from which you want to exclude members.
- ExcludedMembers: The members you want to exclude from UnionType.
Example 1: In this example, We have a union-type Fruit that represents different types of fruits. We use the Exclude<Fruit, “banana” | “date”> utility to create a new type ExcludedFruits, which excludes the “banana” and “date” members from the Fruit union type. When we declare the selectedFruit variable with the type ExcludedFruits, we can assign it a value of “apple” or “cherry” (members that were not excluded). When we attempt to assign “banana” to the rejectedFruit variable of type ExcludedFruits, TypeScript raises an error because “banana” has been explicitly excluded from the type.
Javascript
type Fruit = "apple" | "banana" | "cherry" | "date" ;
type ExcludedFruits = Exclude<Fruit, "banana" | "date" >;
const selectedFruit: ExcludedFruits = "apple" ;
console.log(selectedFruit)
|
Output:

Example 2: In this example, We have a union-type Weather representing different weather conditions. We define a function filterWeather that takes an array of weatherConditions and an excludedWeather of the type Exclude<Weather, “stormy”>. This means that the function will exclude the “stormy” weather conditions from the array. Inside the function, we use the filter method to remove any occurrences of excludedWeather from the weatherConditions array. When we call filterWeather with the all-weather array and “stormy” as the excluded weather condition, it returns an array containing all weather conditions except “stormy”.
Javascript
type Weather = "sunny" | "cloudy" | "rainy" | "stormy" ;
function filterWeather(
weatherConditions: Weather[],
excludedWeather: Exclude<Weather, "stormy" >
): Weather[] {
return weatherConditions.filter((condition) =>
condition !== excludedWeather);
}
const allWeather: Weather[] = [ "sunny" ,
"cloudy" ,
"rainy" ,
"stormy" ,
"sunny" ];
const filteredWeather =
filterWeather(allWeather,
"stormy" as Exclude<Weather,
"stormy" >);
console.log(filteredWeather);
|
Output:
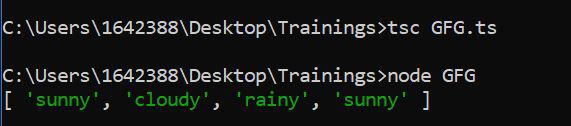
Reference: https://www.typescriptlang.org/docs/handbook/utility-types.html#excludeuniontype-excludedmembers
Share your thoughts in the comments
Please Login to comment...