TypeScript Differences Between Type Aliases and Interfaces Type
Last Updated :
02 Nov, 2023
TypeScript is an open-source programming language that adds static typing and other valuable features to JavaScript. TypeScript is also known as a super-set of JavaScript. Type Aliases and Interfaces Type are used to create custom types in TypeScript. In this article, we will learn about the Differences Between TypeScript Type Aliases and TypeScript Interfaces type.
Type Aliases
Type Aliases in TypeScript allow you to create a new custom type name that can be reused throughout the code in other words a name for any type. Type Aliases are used to create custom names for primitives, arrays, unions, tuples, objects, and function signatures.
Syntax:
type TypeAliaseName = anyType;
Where:
- type is the keyword used for defining the type
- TypeAliaseName is the name of that type
- anyType is the type you want to declare
Example: This example shows the use of the type alises in typescript.
Javascript
type BookName = string;
type BookSerialNum = number;
type IsAvailable = boolean;
type BookReviews = string[];
type Author = [string, number]
type Book = {
book: BookName,
serialNum: BookSerialNum,
isAvailable: IsAvailable,
reviews: BookReviews,
authorInfo: Author,
}
type BookPriceUSA = number;
type BookPriceUK = number;
type BookPrice = BookPriceUSA | BookPriceUK;
type DisplayInfo = (arg: Book) => void;
let book1: BookName =
"Harry Potter and the Philosopher's Stone"
let book1Number: BookSerialNum = 1741239
let book1isAvailable: IsAvailable = true
let book1Reviews: BookReviews =
['good ', ' nice, 10/10 ', ' excellent ']
let book1Author: Author = [' J.K. Rowling', 57]
let book: Book = {
book: book1,
serialNum: book1Number,
isAvailable: book1isAvailable,
reviews: book1Reviews,
authorInfo: book1Author,
}
const display: DisplayInfo = (arg) => {
console.log(arg);
}
display(book);
|
Output:
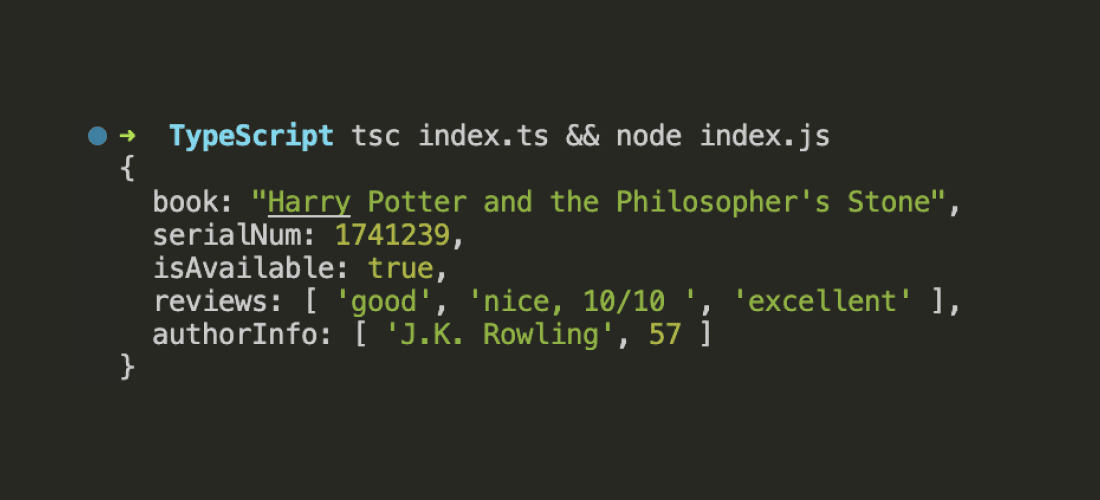
Type aliases example output
Interfaces
Interface in TypeScript is similar to type aliases but It is used to define the shape of an object and the properties and methods it must have. In TypeScript, interface cannot create custom primitives types, arrays, tuples and union types like type aliases.
Syntax:
interface InterfaceName {
// key value pair field
}
Where:
- interface is the keyword used for declaration of interface
- InterfaceName is the name of interface
Example: This example shows the us of interfaces in typescript.
Javascript
interface User {
name: string,
age: number,
}
const person1: User = {
name: 'harry' ,
age: 31,
}
console.log(person1);
interface UserDetails {
(name: string, age: number): void;
}
function displayInfo(
name: string, age: number): void {
console.log(name);
console.log(age);
}
let display: UserDetails = displayInfo;
display( 'Jim' , 35)
|
Output:
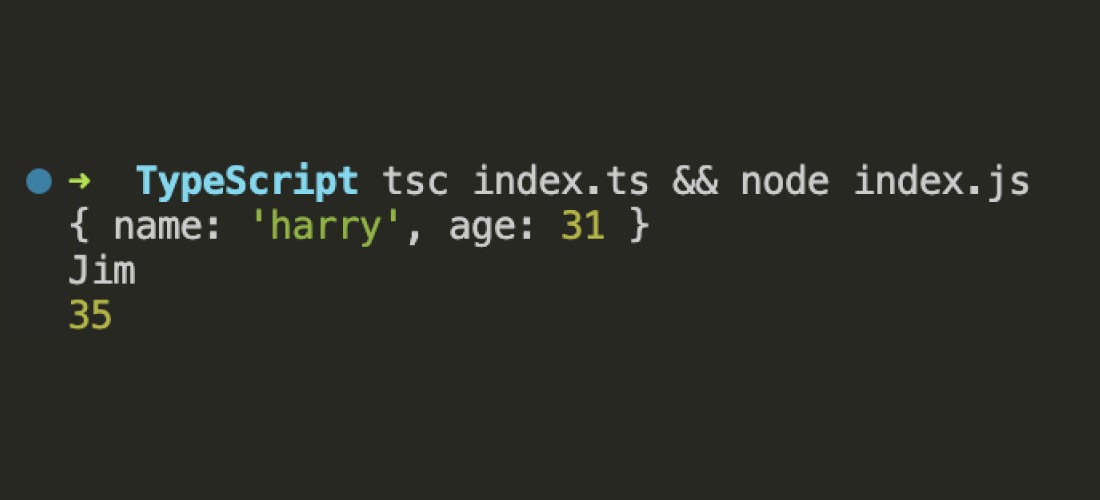
Interface example output
Differences Between TypeScript Type Aliases and TypeScript Interfaces Type:
Type Aliases
|
Interfaces Type
|
Type Aliases use the `type` keyword to define a new type.
|
Interface Type use the `interface` keyword to define a new type.
|
Type aliases support extending other type aliases by intersection type. The `&` symbol is used to create an intersection type. Type aliases also extends interface types.
|
Interface Type also supports extending other interfaces by using the `extends` keyword. Interface type also extends type aliases.
|
Type Aliases can be implemented by class with the use of the `implements` keyword. but they cannot be extended or implemented.
|
Interface Type can also be implemented by class with the use of the `implements` keyword.
|
Type Aliases don’t support declaration merging where declaring the same type of aliases again a second time with another type gives an error.
|
Interface type does support declaration merging where declaring the same interface again a second time merges with previous attributes of the last type interface.
|
Conclusion: In TypeScript, both type aliases and interfaces serve different purposes, and the choice between them depends on your specific use case and coding style. But when it comes to object shaping, Interface Type is best to use. It gives more detailed error messages than type aliases and can be extended with other interfaces, giving more flexibility while working with objects.
Share your thoughts in the comments
Please Login to comment...