TypeScript Control Flow Analysis
Last Updated :
26 Oct, 2023
In this article, we are going to learn about Control flow analysis in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. Control flow analysis in TypeScript refers to the process by which the TypeScript compiler analyzes the flow of your code and makes inferences about the types of variables at different points in your program.
Usage of Control Flow Analysis
- It helps narrow down the possible types of variables based on conditional logic, assignments, and other control flow structures. This narrowing of types allows TypeScript to provide better type-checking and more accurate type information.
- Control flow analysis is often used in conjunction with conditional statements (such as if, else, switch, and while) to determine which branch of code will execute and what the resulting type of variables will be within that branch.
- Control flow analysis is used to narrow the type of a variable within a specific code block or branch of conditional statements.
- When TypeScript determines that a variable can only have a certain type within a block of code, it narrows the type of that variable to a more specific type.
Example 1: In this example, TypeScript uses control flow analysis to narrow the type of the value variable within each if and else if block based on the type guards (typeof). This enables safe and accurate type-checking within each code block.
Javascript
function processValue(value: string | number | null ) {
if ( typeof value === 'string' ) {
console.log(value.toUpperCase());
} else if ( typeof value === 'number' ) {
console.log(value.toFixed(2));
} else {
console.log( 'Value is null or not a string/number' );
}
}
processValue( 'Hello' );
processValue(42);
processValue( null );
|
Output:
Example 2: In this example, the processData function takes an argument data that can be of type string, number, or boolean. TypeScript uses control flow analysis to narrow the type of data within each conditional branch. When data is determined to be a string, the code inside the first if block operates on it as a string. similarly it will check for number and boolean.
Javascript
function processData(data: string | number | boolean) {
if ( typeof data === 'string' ) {
console.log(`Length of the string: ${data.length}`);
} else if ( typeof data === 'number' ) {
if (data > 0) {
console.log(`Square of the number: ${data * data}`);
} else {
console.log( 'The number is not positive.' );
}
} else {
if (data) {
console.log( 'The boolean value is true.' );
} else {
console.log( 'The boolean value is false.' );
}
}
}
processData( 'Hello' );
processData(3);
processData( false );
|
Output: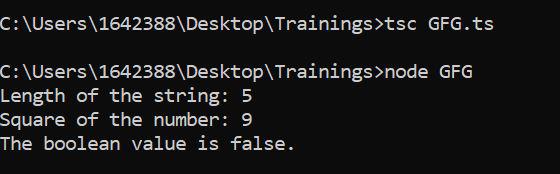
Share your thoughts in the comments
Please Login to comment...