Spring Data JPA – @Modifying Annotation
Last Updated :
05 Apr, 2024
@Modifying annotation in Spring Data JPA allows modification queries such as update and delete and enhances the capabilities of the @Query annotation. It enables data transformation actions beyond simple data retrieval, ensuring transaction integrity and improving performance.
The @Modifying annotation is used to enhance the @Query annotation so that we can not only execute the SELECT queries, but also INSERT, UPDATE, DELETE, and even DDL queries that modify the structure of the underlying database schema using Spring Data JPA
Benefits of @Modifying Annotation
@Modifying annotation is used when you want to execute modifying queries, such as updates or deletes. This annotation is necessary because, by default, Spring Data JPA repository methods are considered read-only and are optimized for querying data, not modifying it.Â
- Improved Performance: Improves performance by allowing changing queries to be executed.
- Transactional Integrity: Ensures transaction integrity while performing data changes.
- Support for native queries: Enables native queries for data transformation.
- Automatic Flush and Clear: Supports automatic flushing and clearing of durability references.
Implementation of @Modifying Annotation of Spring Data JPA
Step 1: Define Entity Class
Define an entity class named User that contains fields such as id, name, age, and isActive to represent user data.
Java
@Entity
@Data
public class User {
// Primary key
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
// User's name
private String name;
// User's age
private int age;
// Indicates if the user is active
@Column(name="active")
private Boolean isActive = true;
}
Step 2: Define Repository Interface
Now we will create a repository interface named UserDao that extends JpaRepository with @Modifying and is annotated with @Query annotation to perform update actions.
Java
@Repository
public interface UserDao extends JpaRepository<User, Integer>{
// Modifying query to update user's active status based on age
@Modifying
@Query(nativeQuery = true, value = "update user u set u.active=0 where u.age < :age")
void updateUser(@Param("age") int age);
}
Step 3: Create Service Class
Now create one class named UserserviceDemo. Inside this class, call the updateUser method that will update data in the database.
Java
@Service
public class UserServiceDemo {
@Autowired
private UserDao userDao;
//get user data from database..
public List<User> getUserDetail() {
return userDao.findAll();
}
//update user data using our custom method..
public String updateUserDetail(int age) {
userDao.updateUser(age);
return "successfully update !!";
}
}
Step 4: Implement Controller
Inside the controller, create one class named UserControllerDemo to process HTTP requests, including using endpoints to retrieve user data and update user information.
Java
@RestController
public class UserControllerDemo {
// Autowired UserServiceDemo bean
@Autowired
private UserServiceDemo userServiceDemo;
// GET endpoint to retrieve user data
@GetMapping("user")
public List<User> getUser() {
return userServiceDemo.getUserDetail();
}
// PUT endpoint to update user details based on age
@PutMapping("updateuser/{age}")
public String updateUser(@PathVariable int age) {
return userServiceDemo.updateUserDetail(age);
}
}
Step 5: Run the application
Now we will run the application and verify the update and delete operations, ensuring successful modification of data in the database.
Output:
Update activation status: false where age less than 20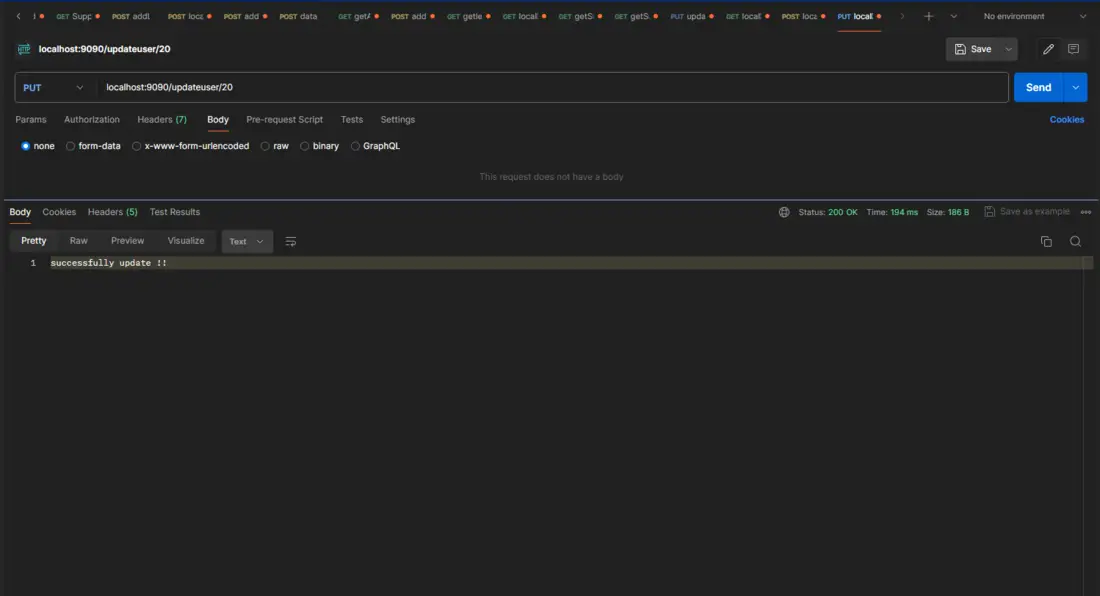
Here, we can see the status update properly in the database.
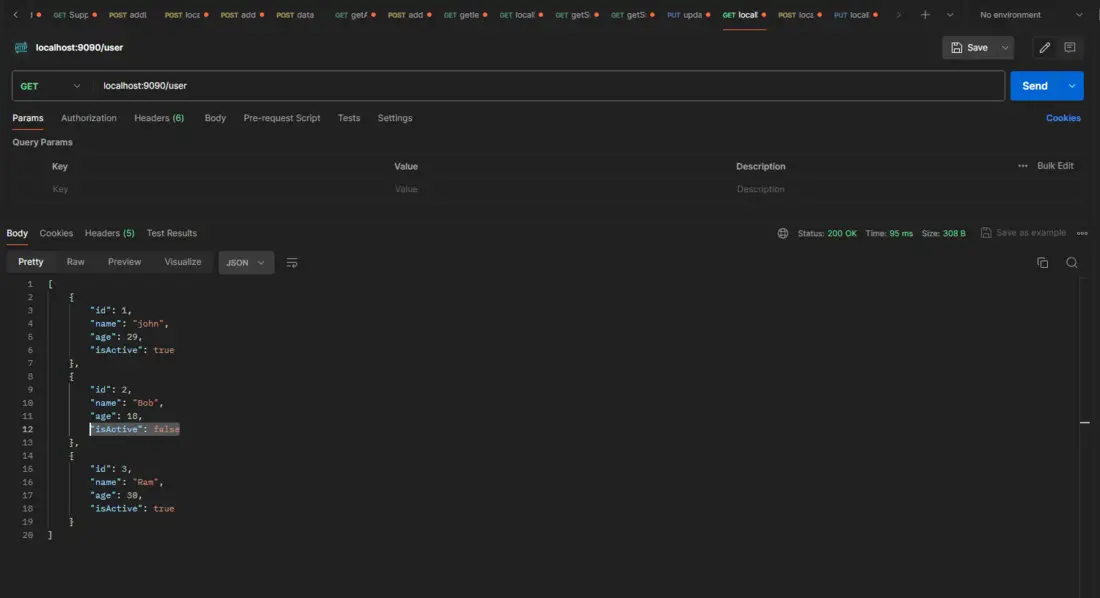
The above is updating data using the @Modifying annotation. If we have to delete data from the database using the @Modifying annotation, then add the below method to the repository.
@Modifying
@Query(nativeQuery = true,value = "delete from user u where u.active = false")
void deleteDeactivatedUsers();
It can successfully delete data from a database where the activation status is false.
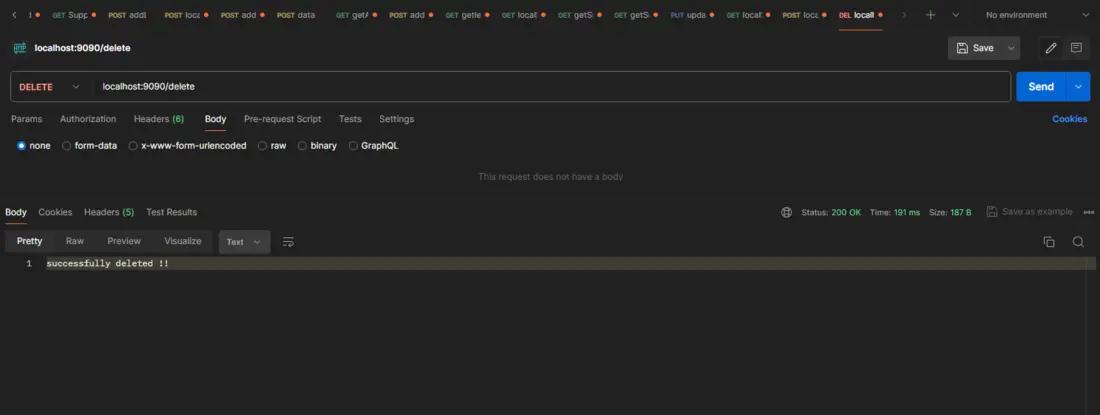
Here, we can see the data was properly deleted in the database.
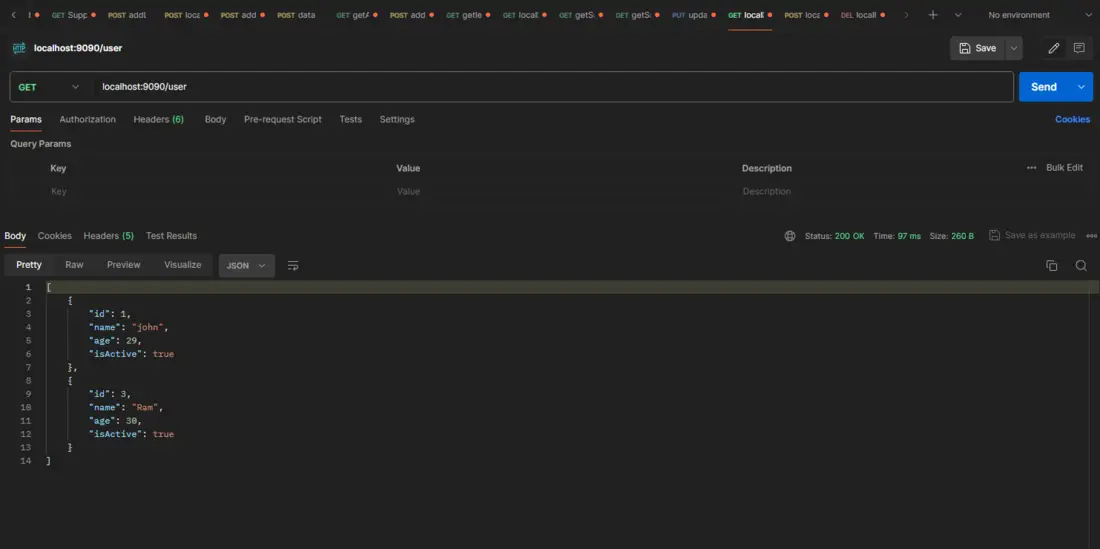
We can see updates and deletes using the @Modifying annotation. Now we can work with DDL queries using the @Modifying annotation, which means alter, drop, etc.
@Modifying
@Query(value = "alter table user u drop column u.login_date", nativeQuery = true)
void deletedColumn();
The above method can be used to drop/delete a column from a database that will be unnecessary in the table. Now we can alter the table using the @Modifying annotation.
Output:
Before executing the above method, the login_date column is unnecessary in database table.
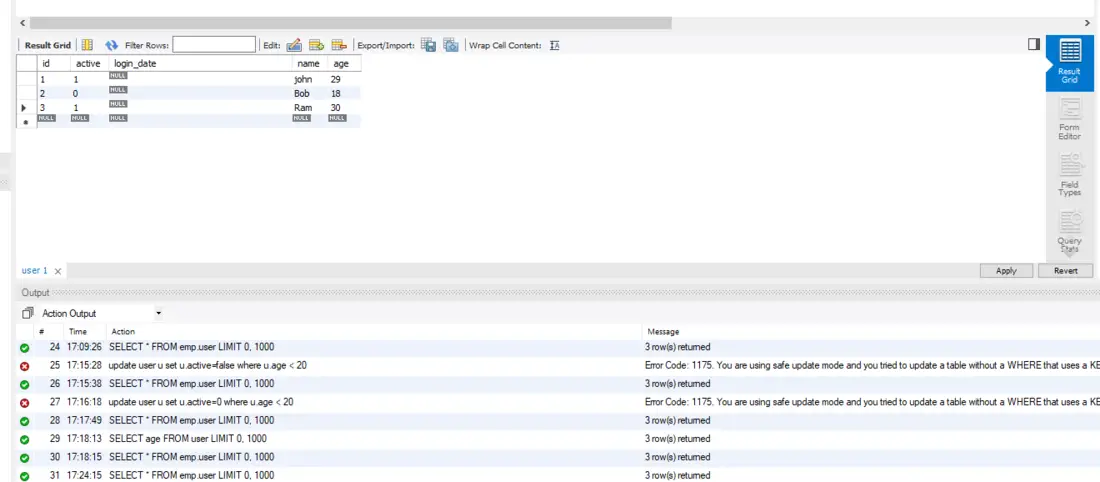
Now, we can execute the deleteColumn() method.
.png)
After executing the method, we can see the column was successfully deleted.
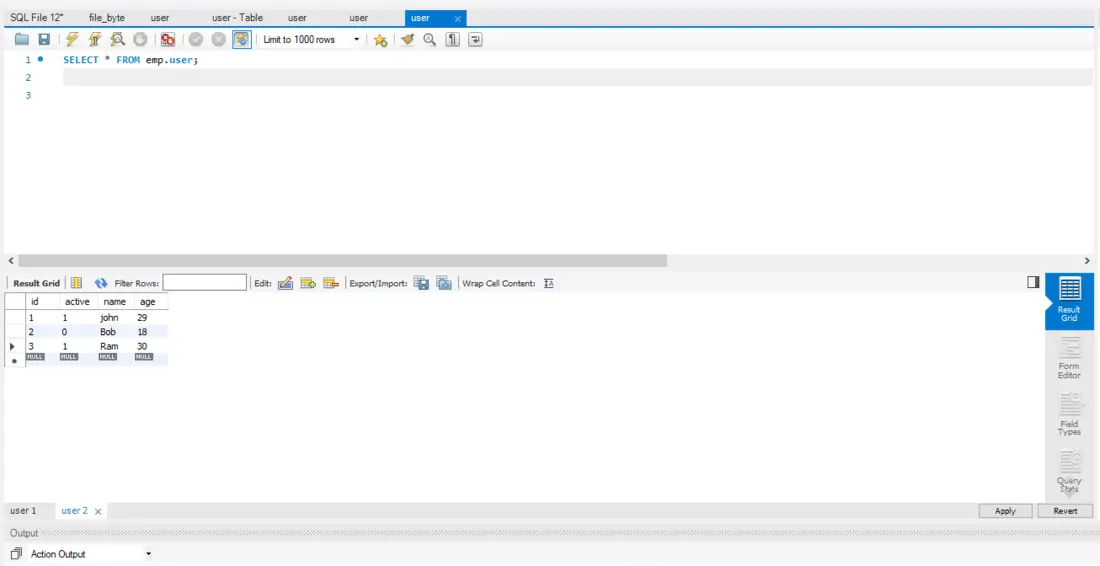
Share your thoughts in the comments
Please Login to comment...