Spring Boot – Scheduling
Last Updated :
28 Mar, 2022
Spring Boot provides the ability to schedule tasks for execution at a given time period with the help of @Scheduled annotation. This article provides a step by step guideline on how we can schedule tasks to run in a spring boot application
Implementation:
It is depicted below stepwise as follows:
Step 1: Creating a spring boot application using Spring Initializer for which one can refer to the basics of creating a Spring class.
Step 2: Specifying @EnableScheduling annotation in the Spring Boot application class.
Java
package com.Scheduler;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class SchedulerApplication {
public static void main(String[] args)
{
SpringApplication.run(SchedulerApplication. class ,
args);
}
}
|
@EnableScheduling annotation facilitates Spring Boot with scheduled task execution capability.
Step 3: Creating a @Component class Scheduler which defines the method scheduleTask() for scheduling a task using the @Scheduled annotation.
The method scheduleTask() in Scheduler class simply prints the date and time at which the task is running.
Scheduling tasks using a cron expression
Java
package com.Scheduler;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class Scheduler {
@Scheduled (cron = "0 * 19 * * ?" )
public void scheduleTask()
{
SimpleDateFormat dateFormat = new SimpleDateFormat(
"dd-MM-yyyy HH:mm:ss.SSS" );
String strDate = dateFormat.format( new Date());
System.out.println(
"Cron job Scheduler: Job running at - "
+ strDate);
}
}
|
The cron element specified in the @Scheduled annotation allows defining cron-like expressions to include triggers on the second, minute, hour, day of the month, month, and day of the week. The expression specified here in the cron element directs spring boot to trigger the scheduler every one minute between 19:00.00 to 19:59.00.
On Running the Spring Boot Application, we can see the output in the console as follows:
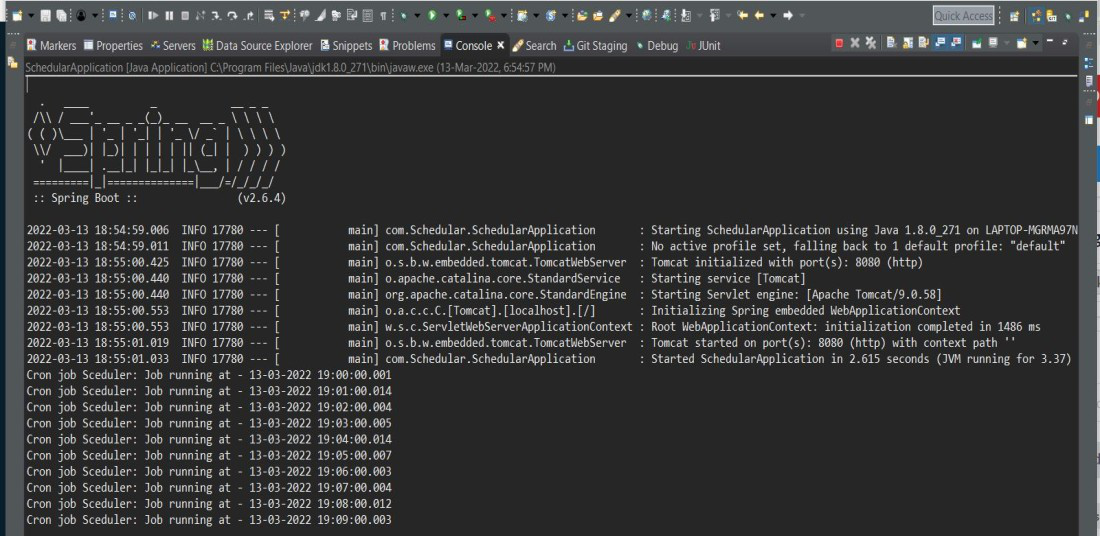
Scheduling tasks at Fixed Rate
Java
package com.Scheduler;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class Scheduler {
@Scheduled (fixedRate = 2000 ) public void scheduleTask()
{
SimpleDateFormat dateFormat = new SimpleDateFormat(
"dd-MM-yyyy HH:mm:ss.SSS" );
String strDate = dateFormat.format( new Date());
System.out.println(
"Fixed rate Scheduler: Task running at - "
+ strDate);
}
}
|
The fixedRate element specified in the @Scheduled annotation executes the annotated method at a fixed time period between invocations. It does not wait for the previous task to be complete. The time value specified for this element is in milliseconds.
Here a fixed rate scheduler is defined which runs every 2 seconds starting at 19:11:58.
On Running the Spring Boot Application, we can see the output in the console as follows:
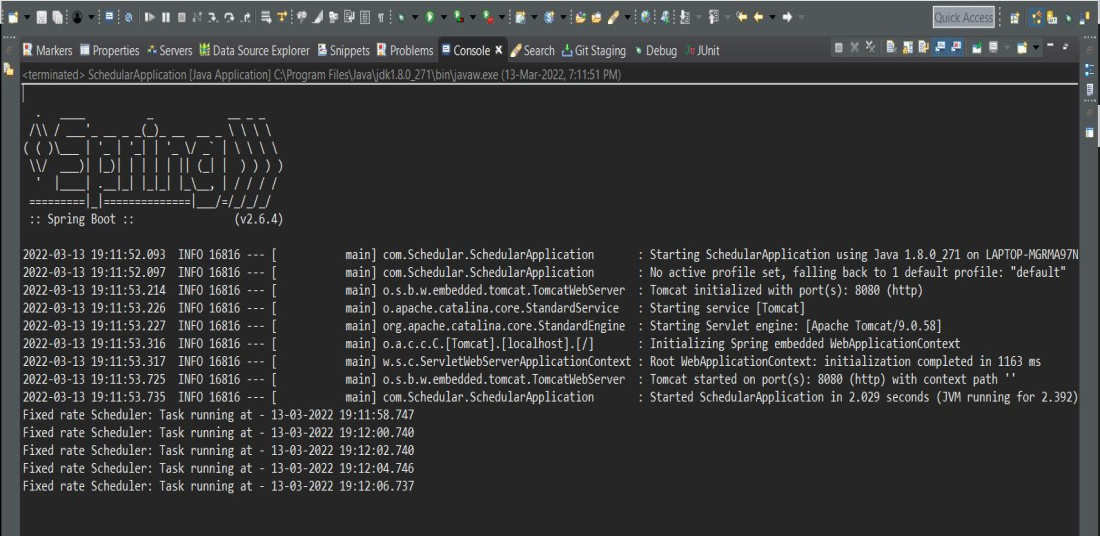
Scheduling tasks to run at Fixed Delay
Java
package com.Scheduler;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class Scheduler {
@Scheduled (fixedDelay = 3000 , initialDelay = 5000 )
public void scheduleTask()
{
SimpleDateFormat dateFormat = new SimpleDateFormat(
"dd-MM-yyyy HH:mm:ss.SSS" );
String strDate = dateFormat.format( new Date());
System.out.println(
"Fixed Delay Scheduler: Task running at - "
+ strDate);
}
}
|
The fixedDelay element specified in the @Scheduled annotation executes the annotated method at a fixed time period between the end of the previous invocation and the start of the next invocation. It basically waits for the previous task to be complete. The time value specified for this element is in milliseconds.
The initialDelay element specified here allows mentioning the amount of time it waits before the invocation of the first task. The time value specified for this element is in milliseconds.
Here the scheduler defined starts with an initial delay of 5 seconds and goes on to execute the task at a fixed delay of 3 seconds.
On Running the Spring Boot Application, we can see the output in the console as follows:
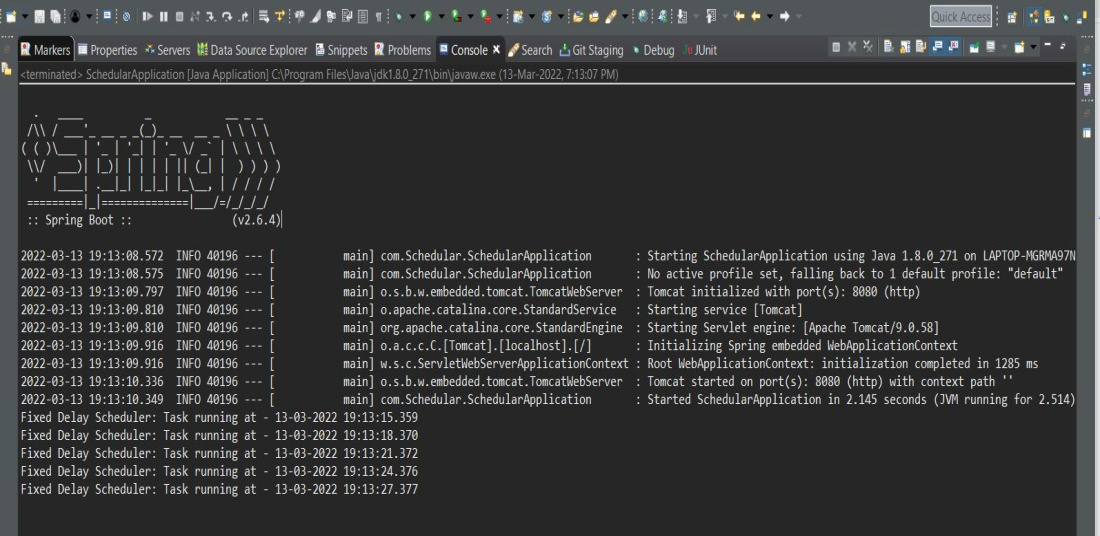
Share your thoughts in the comments
Please Login to comment...