Spring Boot – Project Deployment Using Tomcat
Last Updated :
09 Nov, 2023
Spring Boot is a microservice-based framework and making a production-ready application in it takes very little time. Spring Boot is built on the top of the spring and contains all the features of spring. And is becoming a favorite of developers these days because it’s a rapid production-ready environment that enables the developers to directly focus on the logic instead of struggling with the configuration and setup.
And Tomcat is a very popular Java Servlet Container. Tomcat is the default spring boot server which can manage multiple applications within the same application which avoids multiple setups for each application in a single application.
In this article, we will create a simple spring boot application in which we will deploy the application using the Tomcat server.
Process for Project Deployment on Spring Boot
Spring Boot application deployment on the Tomcat Server involves three steps:
- Create a sample Spring Boot Application
- Ways of creation of a Spring Boot WAR
- Deploying the WAR to Tomcat – Preferably higher versions of Tomcat are required.
To use the appropriate Tomcat version and Java version, it will be helpful to visit the HTML file.
Step 1: Creating a sample Spring Boot Application for Tomcat
This is a spring boot web application project, i.e. the project needs to be deployed on Tomcat. The project can be created as a maven-based project and hence required dependencies we can specify in the pom.xml file.
pom.xml->Configurations can be specified in a Maven project via pom.xml
As the project needs to be deployed using Tomcat, it has to be packaged as “WAR”(Web Application Resource or Web Application Archive).
Basically, pom.xml should have spring boot related dependencies like
- spring-boot-starter-parent
- spring-boot-starter-web
- spring-boot-starter-tomcat and its scope is set to be provided “geeks-web-services” should be the name of “WAR” file as per pom.xml
Example 1:
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >com.geeksforgeeks</ groupId >
< artifactId >spring-boot-war-deployment-example-on-tomcat</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< packaging >war</ packaging >
< name >SpringBootWarDeploymentOnTomcatServer</ name >
< description >Demo project for Spring Boot deployable on Tomcat</ description >
< parent >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-parent</ artifactId >
< version >2.2.2.RELEASE</ version >
< relativePath />
</ parent >
< properties >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
< project.reporting.outputEncoding >UTF-8</ project.reporting.outputEncoding >
< java.version >1.8</ java.version >
</ properties >
< dependencies >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-web</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-tomcat</ artifactId >
< scope >provided</ scope >
</ dependency >
< build >
< finalName >geeks-web-services</ finalName >
< plugins >
< plugin >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-maven-plugin</ artifactId >
</ plugin >
</ plugins >
</ build >
</ project >
|
A controller class is required through which we can able to invoke the services either as a GET method or POST method. As a sample, let us see “SampleRestControllerExample.java” which contains two methods as “GET” methods
Two methods are written which provide a static text as output.
As this is a simple example, just let us show as on calling “geeks-web-services/hello”, displays Hello Geek, this is a simple hello message to take care and have a nice day.
on calling “geeks-web-services/greet”, displays Hello Geek, Fast and easy development can be possible on Spring-based applications by reducing source code.
@RestController is a specialized version of the controller. It includes the @Controller and @ResponseBody annotations, and as a result, simplifies the controller implementation.
Example 2: SampleRestControllerExample.java
Java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SampleRestControllerExample {
@GetMapping ( "/hello" )
public String hello()
{
return "Hello Geek, this is a simple hello message to take care and have a nice day." ;
}
@GetMapping ( "/greet" )
public String greet()
{
return "Hello Geek, Fast and easy development can be possible on Spring-based applications by reducing source code;." ;
}
}
|
Step 2: Ways of creation of a Spring Boot WAR
There are 3 ways of creating a Spring Boot WAR:
- “Main” class should contain with “extends SpringBootServletInitializer” class in the main class.
- “Embedded servlet container should be marked as provided.
- Packaging should be WAR
SpringBootWarDeploymentOnTomcatServer.java is our Main class
Example:
Java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
@SpringBootApplication
public class SpringBootWarDeploymentOnTomcatServer
extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder
configure(SpringApplicationBuilder application){
return application.sources(
SpringBootWarDeploymentOnTomcatServer. class );
}
public static void main(String[] args){
SpringApplication.run(
SpringBootWarDeploymentOnTomcatServer. class ,
args);
}
}
|
We can run the maven steps using the command line,
mvn clean install -X
As an image let us see the project structure and the target folder:
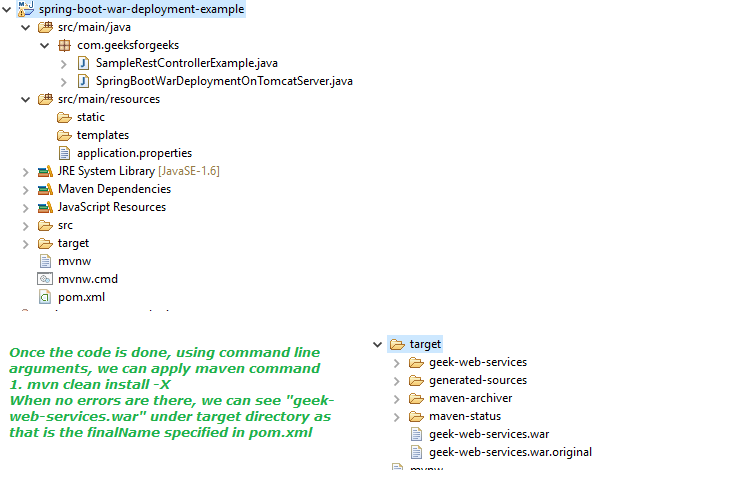
When the WAR file created successfully, it will show the WAR File path with a message i.e. “BUILD SUCCESS” in the console, as shown in the below image:
.webp)
WAR File has created
Step 3: Deploying the WAR to Tomcat
Apache Tomcat Server has to be installed if not installed earlier. Preferably higher versions of tomcat will be helpful. As an example, We are using tomcat version 9.0.x.
To use the appropriate tomcat version and java version, it will be helpful to check the HTML file. In our previous step, we have got “geek-web-services.war” and it has to be copied under the “webapps” folder of tomcat.
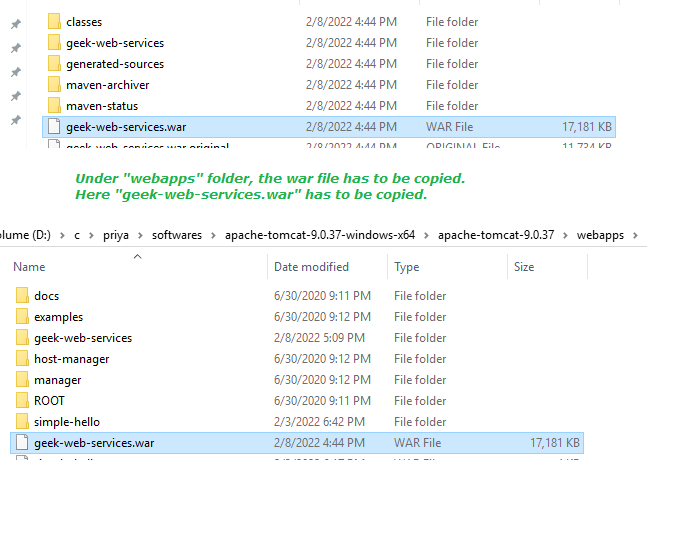
Now, open the command prompt and point to the tomcat location’s bin folder,

From bin folder “startup.bat” needs to be given
.webp)
Image shows Spring Boot has started
And also our war file is deployed in tomcat too. The below screenshot confirms that:

geek-web-services war file deployed
We can test the same by executing the below URL in a browser:
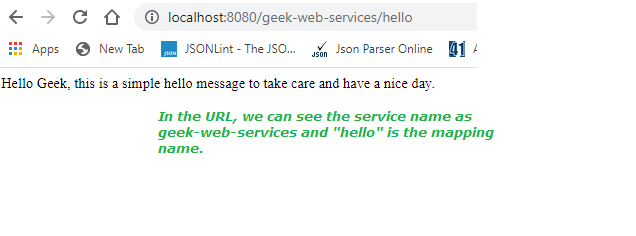
geek-web-services/hello output
2nd Output:
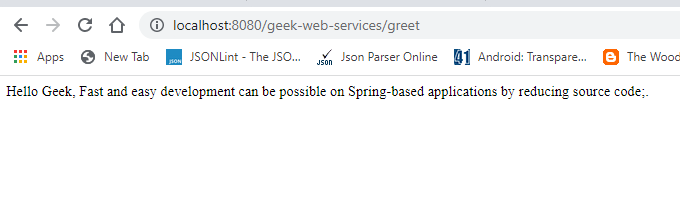
geek-web-services/greetoutput
Project Explanation: It is as justified via video provided below as follows:
Conclusion
As explained above, a spring boot project can be deployable in Tomcat. Do remember certain important points as listed:
- By default, Spring Boot 1.4.4.RELEASE requires Java 7 and Spring Framework 4.3.6.RELEASE or above
- Higher versions of Tomcat will be helpful to deploy spring-boot applications.
Share your thoughts in the comments
Please Login to comment...