Spring Boot – @Async Annotation
Last Updated :
19 Feb, 2024
Synchronization means every process or thread should be Synchronized together. It means executing process by process that’s we can say Synchronization. This is used when one task is complete and only then does another task want to come and be executed. so It looks like there are some limitations. That’s why asynchronization comes into the picture. The Asynchronization process means We are making a different thread for the same processing. Process or thread does not need to wait for someone to execute. This is The Asynchronization process.
Benefits of @Async Annotation
@Async annotation is used when you wanna make any method asynchronize. Annotating a method of a bean with @Async will make it execute in a separate thread. In other words, the caller will not wait for the completion of the called method.
- Easier to implement
- Easier to debug
- More intuitive code flow
- Improved Performance
- Better Scalability
Example Project
Let’s Assume One example. This is a simple Java code that makes the User.
Java
@Entity
@Table (name = "user" )
public class User {
@Id
@GeneratedValue (strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
}
|
This is Synchronize method this method run with main thread.
Java
public UserDto saveUser(UserDto userDto) {
User user = userToDto(userDto);
System.out.println( "user saved :::" +Thread.currentThread().getName());
User saveUser = userRepository.save(user);
return dtoToUser(saveUser);
}
|
We are Using @Async annotation and make method as asynchronized.
Java
@Async
@Override
public void sendNotification(UserDto user)
{
try {
Thread.sleep( 3000 );
}
catch (Exception e) {
e.printStackTrace();
}
System.out.println( "notification sent ::"
+ Thread.currentThread().getName());
}
|
@EnableAsync this application shoud enabling synchronization.
Java
@EnableAsync
@SpringBootApplication
public class BatchPrac3Application {
public static void main(String[] args) {
SpringApplication.run(BatchPrac3Application. class , args);
}
}
|
Output:
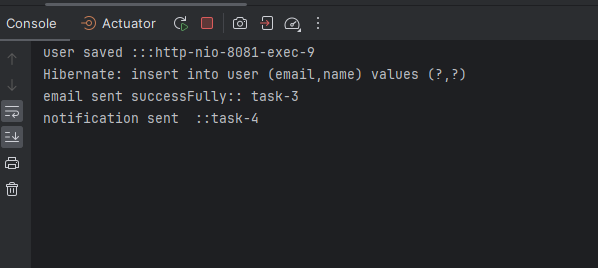
Now, we can see user save task and notification task going into different thread. because of implementing async annotations. It means notification task executed according that required time not need wait for previous task.
Share your thoughts in the comments
Please Login to comment...