Shell Script to validate the date, taking into account leap year rules
Last Updated :
23 Sep, 2022
Prerequisites: Bash Scripting, Shell Function Library
We will build a bash script to check whether the entered date is valid or not according to the Gregorian Calendar rules. If the date is invalid, the script will tell the reason for its invalidity.
- The script takes three arguments – day, month, and year in numeric form.
- The month specified out of the range [1-12] will be shown as invalid.
- Year 0 will be shown as invalid since there is no such year in Gregorian Calendar.
- The months and their corresponding total number of days will be stored in an array.
- The day specified by the user will be checked against the correct day values stored in the script.
- The script takes leap year into account.
Creating Shell Script
The name of the script will be dateChecker.sh. Make it an executable file.
$ touch dateChecker.sh
$ chmod +x dateChecker.sh
Step 1: Handling Arguments
$ ./dateChecker.sh 12 09 #invalid syntax (only 2 args)
$ ./dateChecker.sh twelve 01 2001 #invalid syntax (date should be in numeric form)
$ ./dateChecker.sh 10 10 2020 #valid syntax
Open the file and add the following script :
#!/bin/bash
# if number of args is not 3, show correct syntax
if [ $# -ne 3 ] ; then
echo "The Valid Syntax is :"
echo "\$ ./$(basename $0)"
echo "\$ ./$(basename $0) [d|dd] [m|mm] yyyy"
exit 1
else
day=$1 month=$2 year=$3
fi
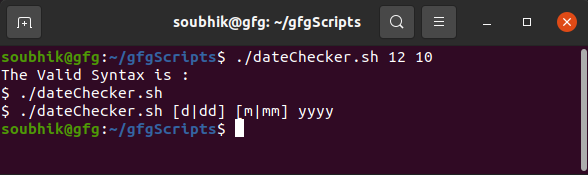
The script shows the correct syntax to the user
Step 2: Checking common errors in the Date
MSG=”Invalid Date!”
# if the args contain any character other than digits
if [[ $day =~ [^[:digit:]] ]] || [[ “$month” == [^[:digit:]] ]] || [[ “$year” == [^[:digit:]] ]] ; then
echo “The day, month and year must be positive numeric only!”
exit 1
# if the months specified is more than 12
elif [ $month -gt 12 ] ; then
echo “There are only 12 months”
echo $MSG
exit 0
# if day, month or year specified is 0
elif [ $year -eq 0 ] || [ $month -eq 0 ] || [ $day -eq 0 ] ; then
echo “There is no zero day, month or year in Gregorian Calendar!”
echo $MSG
exit 0
fi
- If the entered day, month, and year contains any character other than the digits, the script will exit 1.
- If a Month is out of the range [1-12], then it’s an invalid date.
- If the year is 0, it’s invalid.
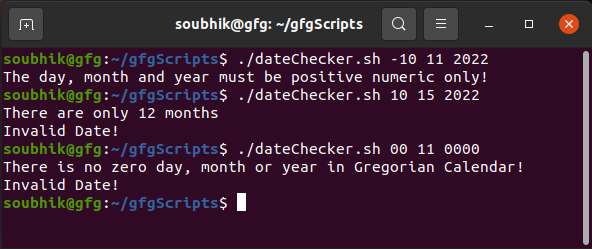
Handling common errors in date
Step 3: Storing the values
#if day, month or year have leading zeroes, stripping them away
day=$((10#$day))
month=$((10#$month))
year=$((10#$year))
#array with months as index and the number of days it has as values
array_months=( 31 28 31 30 31 30 31 31 30 31 30 31 )
month_names=( "January" "February" "March" "April" "May" "June" "July"
"August" "September" "October" "November" "December" )
- Strip away leading zeroes in the day, month, and year variable if any.
- Store the corresponding days of months in array_months.
- Store the names of months in a different array
Step 4: Create the main function
function main ()
{
# determining whether the year specified is leap
if (( $year % 400 == 0 )) || (( $year % 4 == 0 && $year % 100 != 0 )) ; then
#if leap year, February will have 29 days
array_months[1]=29
fi
# zero based indexing in bash arrays
index=$(( $month -1 ))
if [ $day -gt ${array_months[index]} ] ; then
echo "${month_names[index]} has ${array_months[index]} days!"
echo $MSG
else
echo "Valid Date."
fi
}
main
- If it’s a leap year, then we will update the number of days at index 1 (February) of array_months to 29.
- Total Number of Days in a Month = ${array_months[index]} where index = $month – 1 (zero-based indexing).
- Number of Days specified by user = $day
- If $day is greater than the total number of days in that particular month, the date is invalid.
- Otherwise print the date is valid.
Step 5: Putting everything together
#!/bin/bash
# if number of args is not 3, show correct syntax
if [ $# -ne 3 ] ; then
echo “The Valid Syntax is :”
echo “\$ ./$(basename $0)”
echo “\$ ./$(basename $0) [d|dd] [m|mm] yyyy”
exit 1
else
day=$1 month=$2 year=$3
fi
MSG=”Invalid Date!”
# if the args contain any character other than digits
if [[ $day =~ [^[:digit:]] ]] || [[ “$month” == [^[:digit:]] ]] || [[ “$year” == [^[:digit:]] ]] ; then
echo “The day, month and year must be positive numeric only!”
exit 1
# if the months specified is more than 12
elif [ $month -gt 12 ] ; then
echo “There are only 12 months”
echo $MSG
exit 0
# if day, month or year specified is 0
elif [ $year -eq 0 ] || [ $month -eq 0 ] || [ $day -eq 0 ] ; then
echo “There is no zero day, month or year in Gregorian Calendar!”
echo $MSG
exit 0
fi
# if day, month or year have leading zeroes, stripping them away
day=$((10#$day))
month=$((10#$month))
year=$((10#$year))
#array with months as index and the number of days it has as values
array_months=( 31 28 31 30 31 30 31 31 30 31 30 31 )
month_names=( “January” “February” “March” “April” “May” “June” “July”
“August” “September” “October” “November” “December” )
function main ()
{
#determining whether the year specified is leap
if (( $year % 400 == 0 )) || (( $year % 4 == 0 && $year % 100 != 0 )) ; then
#if leap year, February will have 29 days
array_months[1]=29
fi
#zero based indexing in bash arrays
index=$(( $month -1 ))
if [ $day -gt ${array_months[index]} ] ; then
echo “${month_names[index]} has ${array_months[index]} days!”
echo $MSG
else
echo “Valid Date.”
fi
}
main
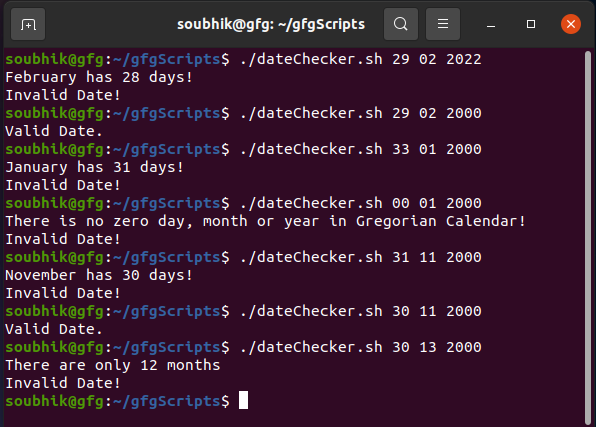
Displaying which dates are valid and which dates are invalid and the reason as well.
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...